#include <konq_historymgr.h>
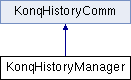
Public Slots | |
bool | loadHistory () |
bool | saveHistory () |
void | emitClear () |
Signals | |
void | loadingFinished () |
void | entryAdded (const KonqHistoryEntry *entry) |
void | entryRemoved (const KonqHistoryEntry *entry) |
Public Member Functions | |
KonqHistoryManager (TQObject *parent, const char *name) | |
void | emitSetMaxCount (TQ_UINT32 count) |
void | emitSetMaxAge (TQ_UINT32 days) |
void | emitRemoveFromHistory (const KURL &url) |
void | emitRemoveFromHistory (const KURL::List &urls) |
TQ_UINT32 | maxCount () const |
TQ_UINT32 | maxAge () const |
void | addPending (const KURL &url, const TQString &typedURL=TQString::null, const TQString &title=TQString::null) |
void | confirmPending (const KURL &url, const TQString &typedURL=TQString::null, const TQString &title=TQString::null) |
void | removePending (const KURL &url) |
TDECompletion * | completionObject () const |
const KonqHistoryList & | entries () const |
virtual void | insert (const TQString &) |
virtual void | remove (const TQString &) |
virtual void | clear () |
Static Public Member Functions | |
static KonqHistoryManager * | kself () |
Protected Member Functions | |
void | adjustSize () |
bool | isExpired (KonqHistoryEntry *entry) |
void | emitAddToHistory (const KonqHistoryEntry &entry) |
virtual void | notifyHistoryEntry (KonqHistoryEntry e, TQCString saveId) |
virtual void | notifyMaxCount (TQ_UINT32 count, TQCString saveId) |
virtual void | notifyMaxAge (TQ_UINT32 days, TQCString saveId) |
virtual void | notifyClear (TQCString saveId) |
virtual void | notifyRemove (KURL url, TQCString saveId) |
virtual void | notifyRemove (KURL::List urls, TQCString saveId) |
virtual TQStringList | allURLs () const |
void | addToHistory (bool pending, const KURL &url, const TQString &typedURL=TQString::null, const TQString &title=TQString::null) |
virtual bool | filterOut (const KURL &url) |
void | addToUpdateList (const TQString &url) |
![]() | |
KonqHistoryComm (TQCString objId) | |
Protected Attributes | |
TQStringList | m_updateURLs |
Detailed Description
This class maintains and manages a history of all URLs visited by one Konqueror instance.
Additionally it synchronizes the history with other Konqueror instances via DCOP to keep one global and persistant history.
It keeps the history in sync with one TDECompletion object
Definition at line 74 of file konq_historymgr.h.
Member Function Documentation
◆ addPending()
void KonqHistoryManager::addPending | ( | const KURL & | url, |
const TQString & | typedURL = TQString::null , |
||
const TQString & | title = TQString::null |
||
) |
Adds a pending entry to the history.
Pending means, that the entry is not verified yet, i.e. it is not sure url
does exist at all. You probably don't know the title of the url in that case either. Call confirmPending() as soon you know the entry is good and should be updated.
If an entry with url
already exists, it will be updated (lastVisited date will become the current time and the number of visits will be incremented).
- Parameters
-
url The url of the history entry typedURL the string that the user typed, which resulted in url Doesn't have to be a valid url, e.g. "slashdot.org". title The title of the URL. If you don't know it (yet), you may specify it in confirmPending().
Definition at line 256 of file konq_historymgr.cpp.
◆ addToHistory()
|
protected |
Does the work for addPending() and confirmPending().
Adds an entry to the history. If an entry with url
already exists, it will be updated (lastVisited date will become the current time and the number of visits will be incremented). pending
means, the entry has not been "verified", it's been added right after typing the url. If pending
is false, url
will be removed from the pending urls (if available) and NOT be added again in that case.
Definition at line 270 of file konq_historymgr.cpp.
◆ adjustSize()
|
protected |
Resizes the history list to contain less or equal than m_maxCount entries.
The first (oldest) entries are removed.
Definition at line 236 of file konq_historymgr.cpp.
◆ allURLs()
|
protectedvirtual |
- Returns
- a list of all urls in the history.
Implements KonqHistoryComm.
Definition at line 675 of file konq_historymgr.cpp.
◆ completionObject()
|
inline |
- Returns
- the TDECompletion object.
Definition at line 169 of file konq_historymgr.h.
◆ confirmPending()
void KonqHistoryManager::confirmPending | ( | const KURL & | url, |
const TQString & | typedURL = TQString::null , |
||
const TQString & | title = TQString::null |
||
) |
Confirms and updates the entry for url
.
Definition at line 262 of file konq_historymgr.cpp.
◆ emitAddToHistory()
|
protected |
Notifes all running instances about a new HistoryEntry via DCOP.
Definition at line 350 of file konq_historymgr.cpp.
◆ emitClear
|
slot |
Clears the history and tells all other Konqueror instances via DCOP to do the same.
The history is saved afterwards, if necessary.
Definition at line 413 of file konq_historymgr.cpp.
◆ emitRemoveFromHistory() [1/2]
void KonqHistoryManager::emitRemoveFromHistory | ( | const KURL & | url | ) |
Removes the history entry for url
, if existant.
Tells all other Konqueror instances via DCOP to do the same.
The history is saved after receiving the DCOP call.
Definition at line 395 of file konq_historymgr.cpp.
◆ emitRemoveFromHistory() [2/2]
void KonqHistoryManager::emitRemoveFromHistory | ( | const KURL::List & | urls | ) |
Removes the history entries for the given list of urls
.
Tells all other Konqueror instances via DCOP to do the same.
The history is saved after receiving the DCOP call.
Definition at line 404 of file konq_historymgr.cpp.
◆ emitSetMaxAge()
void KonqHistoryManager::emitSetMaxAge | ( | TQ_UINT32 | days | ) |
Sets a new maximum age of history entries and removes all entries that are older than days
.
Notifies all other Konqueror instances via DCOP to do the same.
An age of 0 means no expiry based on the age.
The history is saved after receiving the DCOP call.
Definition at line 431 of file konq_historymgr.cpp.
◆ emitSetMaxCount()
void KonqHistoryManager::emitSetMaxCount | ( | TQ_UINT32 | count | ) |
Sets a new maximum size of history and truncates the current history if necessary.
Notifies all other Konqueror instances via DCOP to do the same.
The history is saved after receiving the DCOP call.
Definition at line 422 of file konq_historymgr.cpp.
◆ entries()
|
inline |
- Returns
- the list of all history entries, sorted by date (oldest entries first)
Definition at line 175 of file konq_historymgr.h.
◆ entryAdded
|
signal |
Emitted after a new entry was added.
◆ entryRemoved
|
signal |
Emitted after an entry was removed from the history Note, that this entry will be deleted immediately after you got that signal.
◆ filterOut()
|
protectedvirtual |
- Returns
- true if the given
url
should be filtered out and not be added to the history. By default, all local urls (url.isLocalFile()) will return true, as well as urls with an empty host.
Definition at line 664 of file konq_historymgr.cpp.
◆ insert()
|
virtual |
Reimplemented in such a way that all URLs that would be filtered out normally (see filterOut()) will still be added to the history.
By default, file:/ urls will be filtered out, but if they come thru the HistoryProvider interface, they are added to the history.
Definition at line 336 of file konq_historymgr.cpp.
◆ isExpired()
|
inlineprotected |
- Returns
- true if
entry
is older than the given maximum age, otherwise false.
Definition at line 237 of file konq_historymgr.h.
◆ loadHistory
|
slot |
Loads the history and fills the completion object.
Definition at line 81 of file konq_historymgr.cpp.
◆ loadingFinished
|
signal |
Emitted after the entire history was loaded from disk.
◆ maxAge()
|
inline |
- Returns
- the current maximum age (in days) of history entries.
Definition at line 131 of file konq_historymgr.h.
◆ maxCount()
|
inline |
- Returns
- the current maximum number of history entries.
Definition at line 126 of file konq_historymgr.h.
◆ notifyClear()
|
protectedvirtual |
Clears the history completely.
Called via DCOP by some config-module
Implements KonqHistoryComm.
Definition at line 522 of file konq_historymgr.cpp.
◆ notifyHistoryEntry()
|
protectedvirtual |
Every konqueror instance broadcasts new history entries to the other konqueror instances.
Those add the entry to their list, but don't save the list, because the sender saves the list.
- Parameters
-
e the new history entry saveId is the DCOPObject::objId() of the sender so that only the sender saves the new history.
Implements KonqHistoryComm.
Definition at line 443 of file konq_historymgr.cpp.
◆ notifyMaxAge()
|
protectedvirtual |
Called when the configuration of the maximum age of history-entries changed.
Called via DCOP by some config-module
Implements KonqHistoryComm.
Definition at line 506 of file konq_historymgr.cpp.
◆ notifyMaxCount()
|
protectedvirtual |
Called when the configuration of the maximum count changed.
Called via DCOP by some config-module
Implements KonqHistoryComm.
Definition at line 490 of file konq_historymgr.cpp.
◆ notifyRemove() [1/2]
|
protectedvirtual |
Notifes about a url that has to be removed from the history.
The instance where saveId == objId() has to save the history.
Implements KonqHistoryComm.
Definition at line 534 of file konq_historymgr.cpp.
◆ notifyRemove() [2/2]
|
protectedvirtual |
Notifes about a list of urls that has to be removed from the history.
The instance where saveId == objId() has to save the history.
Implements KonqHistoryComm.
Definition at line 558 of file konq_historymgr.cpp.
◆ removePending()
void KonqHistoryManager::removePending | ( | const KURL & | url | ) |
Removes a pending url from the history, e.g.
when the url does not exist, or the user aborted loading.
Definition at line 364 of file konq_historymgr.cpp.
◆ saveHistory
|
slot |
Saves the entire history.
Definition at line 200 of file konq_historymgr.cpp.
Member Data Documentation
◆ m_updateURLs
|
protected |
The list of urls that is going to be emitted in slotEmitUpdated.
Add urls to it whenever you modify the list of history entries and start m_updateTimer.
Definition at line 325 of file konq_historymgr.h.
The documentation for this class was generated from the following files: