#include <kdecoration.h>
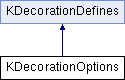
Public Member Functions | |
const TQColor & | color (ColorType type, bool active=true) const |
const TQColorGroup & | colorGroup (ColorType type, bool active=true) const |
const TQFont & | font (bool active=true, bool small=false) const |
bool | customButtonPositions () const |
TQString | titleButtonsLeft () const |
TQString | titleButtonsRight () const |
bool | showTooltips () const |
BorderSize | preferredBorderSize (KDecorationFactory *factory) const |
bool | moveResizeMaximizedWindows () const |
WindowOperation | operationMaxButtonClick (TQt::ButtonState button) const |
virtual unsigned long | updateSettings ()=0 |
Protected Attributes | |
KDecorationOptionsPrivate * | d |
Additional Inherited Members | |
![]() | |
enum | Position { PositionCenter = 0x00 , PositionLeft = 0x01 , PositionRight = 0x02 , PositionTop = 0x04 , PositionBottom = 0x08 , PositionTopLeft = PositionLeft | PositionTop , PositionTopRight = PositionRight | PositionTop , PositionBottomLeft = PositionLeft | PositionBottom , PositionBottomRight = PositionRight | PositionBottom } |
enum | MaximizeMode { MaximizeRestore = 0 , MaximizeVertical = 1 , MaximizeHorizontal = 2 , MaximizeFull = MaximizeVertical | MaximizeHorizontal } |
enum | WindowOperation { MaximizeOp = 5000 , RestoreOp , MinimizeOp , MoveOp , UnrestrictedMoveOp , ResizeOp , UnrestrictedResizeOp , CloseOp , OnAllDesktopsOp , ShadeOp , KeepAboveOp , KeepBelowOp , OperationsOp , WindowRulesOp , ToggleStoreSettingsOp = WindowRulesOp , HMaximizeOp , VMaximizeOp , LowerOp , FullScreenOp , NoBorderOp , NoOp , SetupWindowShortcutOp , ApplicationRulesOp , ShadowOp , SuspendWindowOp , ResumeWindowOp } |
enum | ColorType { ColorTitleBar , ColorTitleBlend , ColorFont , ColorButtonBg , ColorFrame , ColorHandle , NUM_COLORS } |
enum | { SettingDecoration = 1 << 0 , SettingColors = 1 << 1 , SettingFont = 1 << 2 , SettingButtons = 1 << 3 , SettingTooltips = 1 << 4 , SettingBorder = 1 << 5 } |
enum | BorderSize { BorderTiny , BorderNormal , BorderLarge , BorderVeryLarge , BorderHuge , BorderVeryHuge , BorderOversized , BordersCount } |
enum | Ability { AbilityAnnounceButtons = 0 , AbilityButtonMenu = 1000 , AbilityButtonOnAllDesktops = 1001 , AbilityButtonSpacer = 1002 , AbilityButtonHelp = 1003 , AbilityButtonMinimize = 1004 , AbilityButtonMaximize = 1005 , AbilityButtonClose = 1006 , AbilityButtonAboveOthers = 1007 , AbilityButtonBelowOthers = 1008 , AbilityButtonShade = 1009 , AbilityButtonResize = 1010 , ABILITY_DUMMY = 10000000 } |
enum | Requirement { REQUIREMENT_DUMMY = 1000000 } |
Detailed Description
This class holds various configuration settings for the decoration.
It is accessible from the decorations either as KDecoration::options() or KDecorationFactory::options().
- Since
- 3.2
Definition at line 190 of file kdecoration.h.
Member Function Documentation
◆ color()
const TQColor & KDecorationOptions::color | ( | ColorType | type, |
bool | active = true |
||
) | const |
Returns the color that should be used for the given part of the decoration.
The changed flags for this setting is SettingColors.
- Parameters
-
type The requested color type. active Whether the color should be for active or inactive windows.
Definition at line 378 of file kdecoration.cpp.
◆ colorGroup()
const TQColorGroup & KDecorationOptions::colorGroup | ( | ColorType | type, |
bool | active = true |
||
) | const |
Returns a colorgroup using the given decoration color as the background.
The changed flags for this setting is SettingColors.
- Parameters
-
type The requested color type. active Whether to return the color for active or inactive windows.
Definition at line 391 of file kdecoration.cpp.
◆ customButtonPositions()
bool KDecorationOptions::customButtonPositions | ( | ) | const |
Returns true if the style should use custom button positions The changed flags for this setting is SettingButtons.
- See also
- titleButtonsLeft
- titleButtonsRight
Definition at line 403 of file kdecoration.cpp.
◆ font()
const TQFont & KDecorationOptions::font | ( | bool | active = true , |
bool | small = false |
||
) | const |
Returns the active or inactive decoration font.
The changed flags for this setting is SettingFont.
- Parameters
-
active Whether to return the color for active or inactive windows. small If true, returns a font that's suitable for tool windows.
Definition at line 383 of file kdecoration.cpp.
◆ preferredBorderSize()
KDecorationOptions::BorderSize KDecorationOptions::preferredBorderSize | ( | KDecorationFactory * | factory | ) | const |
The preferred border size selected by the user, e.g.
for accessibility reasons, or when using high resolution displays. It's up to the decoration to decide which borders or if any borders at all will obey this setting. It is guaranteed that the returned value will be one of those returned by KDecorationFactory::borderSizes(), so if that one hasn't been reimplemented, BorderNormal is always returned. The changed flags for this setting is SettingBorder.
- Parameters
-
factory the decoration factory used
Definition at line 423 of file kdecoration.cpp.
◆ showTooltips()
bool KDecorationOptions::showTooltips | ( | ) | const |
- Returns
- true if the style should use tooltips for window buttons The changed flags for this setting is SettingTooltips.
Definition at line 418 of file kdecoration.cpp.
◆ titleButtonsLeft()
TQString KDecorationOptions::titleButtonsLeft | ( | ) | const |
If customButtonPositions() returns true, titleButtonsLeft returns which buttons should be on the left side of the titlebar from left to right.
Characters in the returned string have this meaning :
- 'M' menu button
- 'S' on_all_desktops button
- 'H' quickhelp button
- 'I' minimize ( iconify ) button
- 'A' maximize button
- 'X' close button
- 'F' keep_above_others button
- 'B' keep_below_others button
- 'L' shade button
- 'R' resize button
- '_' spacer
The default ( which is also returned if customButtonPositions returns false ) is "MS". Unknown buttons in the returned string must be ignored. The changed flags for this setting is SettingButtons.
Definition at line 408 of file kdecoration.cpp.
◆ titleButtonsRight()
TQString KDecorationOptions::titleButtonsRight | ( | ) | const |
If customButtonPositions() returns true, titleButtonsRight returns which buttons should be on the right side of the titlebar from left to right.
Characters in the return string have the same meaning like in titleButtonsLeft().
The default ( which is also returned if customButtonPositions returns false ) is "HIAX". Unknown buttons in the returned string must be ignored. The changed flags for this setting is SettingButtons.
Definition at line 413 of file kdecoration.cpp.
The documentation for this class was generated from the following files: