#include <katesupercursor.h>
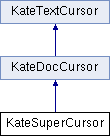
Signals | |
void | positionDirectlyChanged () |
void | positionChanged () |
void | positionUnChanged () |
void | positionDeleted () |
void | charInsertedAt () |
void | charDeletedBefore () |
void | charDeletedAfter () |
Public Member Functions | |
KateSuperCursor (KateDocument *doc, bool privateC, const KateTextCursor &cursor, TQObject *parent=0L, const char *name=0L) | |
KateSuperCursor (KateDocument *doc, bool privateC, int lineNum=0, int col=0, TQObject *parent=0L, const char *name=0L) | |
void | position (uint *line, uint *col) const |
bool | setPosition (uint line, uint col) |
bool | insertText (const TQString &text) |
bool | removeText (uint numberOfCharacters) |
TQChar | currentChar () const |
bool | atStartOfLine () const |
bool | atEndOfLine () const |
bool | moveOnInsert () const |
void | setMoveOnInsert (bool moveOnInsert) |
operator TQString () | |
virtual void | setLine (int lineNum) |
virtual void | setCol (int colNum) |
virtual void | setPos (const KateTextCursor &pos) |
virtual void | setPos (int lineNum, int colNum) |
void | editTextInserted (uint line, uint col, uint len) |
void | editTextRemoved (uint line, uint col, uint len) |
void | editLineWrapped (uint line, uint col, bool newLine=true) |
void | editLineUnWrapped (uint line, uint col, bool removeLine=true, uint length=0) |
void | editLineInserted (uint line) |
void | editLineRemoved (uint line) |
![]() | |
KateDocCursor (KateDocument *doc) | |
KateDocCursor (int line, int col, KateDocument *doc) | |
bool | validPosition (uint line, uint col) |
bool | validPosition () |
bool | gotoNextLine () |
bool | gotoPreviousLine () |
bool | gotoEndOfNextLine () |
bool | gotoEndOfPreviousLine () |
int | nbCharsOnLineAfter () |
bool | moveForward (uint nbChar) |
bool | moveBackward (uint nbChar) |
void | position (uint *line, uint *col) const |
bool | setPosition (uint line, uint col) |
bool | insertText (const TQString &text) |
bool | removeText (uint numberOfCharacters) |
TQChar | currentChar () const |
uchar | currentAttrib () const |
bool | nextNonSpaceChar () |
bool | previousNonSpaceChar () |
![]() | |
KateTextCursor (int line, int col) | |
void | pos (int *pline, int *pcol) const |
int | line () const |
int | col () const |
Additional Inherited Members | |
![]() | |
KateDocument * | m_doc |
![]() | |
int | m_line |
int | m_col |
Detailed Description
Possible additional features:
- Notification when a cursor enters or exits a view
- suggest something :)
Unresolved issues:
- testing, testing, testing
- ie. everything which hasn't already been tested, you can see that which has inside the #ifdefs
- api niceness A cursor which updates and gives off various interesting signals.
This aims to be a working version of what KateCursor was originally intended to be.
Definition at line 45 of file katesupercursor.h.
Constructor & Destructor Documentation
◆ KateSuperCursor()
KateSuperCursor::KateSuperCursor | ( | KateDocument * | doc, |
bool | privateC, | ||
const KateTextCursor & | cursor, | ||
TQObject * | parent = 0L , |
||
const char * | name = 0L |
||
) |
bool privateC says: if private, than don't show to apps using the cursorinterface in the list, all internally only used SuperCursors should be private or people could modify them from the outside breaking kate's internals
Definition at line 28 of file katesupercursor.cpp.
Member Function Documentation
◆ atEndOfLine()
bool KateSuperCursor::atEndOfLine | ( | ) | const |
- Returns
- true if the cursor is situated at the end of the line, false if it isn't.
Definition at line 90 of file katesupercursor.cpp.
◆ atStartOfLine()
bool KateSuperCursor::atStartOfLine | ( | ) | const |
- Returns
- true if the cursor is situated at the start of the line, false if it isn't.
Definition at line 85 of file katesupercursor.cpp.
◆ charDeletedAfter
|
signal |
The character immediately after the cursor was deleted.
◆ charDeletedBefore
|
signal |
The character immediately before the cursor was deleted.
◆ charInsertedAt
|
signal |
A character was inserted immediately before the cursor.
Whether the char was inserted before or after this cursor depends on moveOnInsert():
- true -> the char was inserted before
- false -> the char was inserted after
◆ moveOnInsert()
bool KateSuperCursor::moveOnInsert | ( | ) | const |
Returns how this cursor behaves when text is inserted at the cursor.
Defaults to not moving on insert.
Definition at line 95 of file katesupercursor.cpp.
◆ operator TQString()
KateSuperCursor::operator TQString | ( | ) |
Debug: output the position.
Definition at line 275 of file katesupercursor.cpp.
◆ positionChanged
|
signal |
The cursor's position was changed.
◆ positionDeleted
|
signal |
The cursor's surrounding characters were both deleted simultaneously.
The cursor is automatically placed at the start of the deleted region.
◆ positionDirectlyChanged
|
signal |
The cursor's position was directly changed by the program.
◆ positionUnChanged
|
signal |
Athough an edit took place, the cursor's position was unchanged.
◆ setMoveOnInsert()
void KateSuperCursor::setMoveOnInsert | ( | bool | moveOnInsert | ) |
Change the behavior of the cursor when text is inserted at the cursor.
If moveOnInsert
is true, the cursor will end up at the end of the insert.
Definition at line 100 of file katesupercursor.cpp.
The documentation for this class was generated from the following files: