#include <kateautoindent.h>
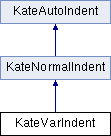
Public Types | |
enum | pairs { Parens =1 , Braces =2 , Brackets =4 , AngleBrackets =8 } |
Public Member Functions | |
KateVarIndent (KateDocument *doc) | |
virtual void | processNewline (KateDocCursor &cur, bool needContinue) |
virtual void | processChar (TQChar c) |
virtual void | processLine (KateDocCursor &line) |
virtual void | processSection (const KateDocCursor &begin, const KateDocCursor &end) |
virtual bool | canProcessLine () const |
virtual uint | modeNumber () const |
![]() | |
KateNormalIndent (KateDocument *doc) | |
virtual | ~KateNormalIndent () |
virtual bool | canProcessNewLine () const |
![]() | |
KateAutoIndent (KateDocument *doc) | |
virtual | ~KateAutoIndent () |
Additional Inherited Members | |
![]() | |
virtual void | updateConfig () |
![]() | |
virtual void | updateConfig () |
![]() | |
static KateAutoIndent * | createIndenter (KateDocument *doc, uint mode) |
static TQStringList | listModes () |
static TQString | modeName (uint mode) |
static TQString | modeDescription (uint mode) |
static uint | modeNumber (const TQString &name) |
static bool | hasConfigPage (uint mode) |
static IndenterConfigPage * | configPage (TQWidget *parent, uint mode) |
![]() | |
uchar | commentAttrib |
uchar | doxyCommentAttrib |
uchar | regionAttrib |
uchar | symbolAttrib |
uchar | alertAttrib |
uchar | tagAttrib |
uchar | wordAttrib |
uchar | keywordAttrib |
uchar | normalAttrib |
uchar | extensionAttrib |
uchar | preprocessorAttrib |
uchar | stringAttrib |
uchar | charAttrib |
![]() | |
bool | isBalanced (KateDocCursor &begin, const KateDocCursor &end, TQChar open, TQChar close, uint &pos) const |
bool | skipBlanks (KateDocCursor &cur, KateDocCursor &max, bool newline) const |
uint | measureIndent (KateDocCursor &cur) const |
TQString | tabString (uint length) const |
![]() | |
uint | tabWidth |
uint | indentWidth |
bool | useSpaces |
bool | mixedIndent |
bool | keepProfile |
![]() | |
KateDocument * | doc |
Detailed Description
This indenter uses document variables to determine when to add/remove indents.
It attempts to get the following variables from the document:
- var-indent-indent-after: A rerular expression which will cause a line to be indented by one unit, if the first non-whitespace-only line above matches.
- var-indent-indent: A regular expression, which will cause a matching line to be indented by one unit.
- var-indent-unindent: A regular expression which will cause the line to be unindented by one unit if matching.
- var-indent-triggerchars: a list of characters that should cause the indentiou to be recalculated immediately when typed.
- var-indent-handle-couples: a list of paren sets to handle. Any combination of 'parens' 'braces' and 'brackets'. Each set type is handled the following way: If there are unmatched opening instances on the above line, one indent unit is added, if there are unmatched closing instances on the current line, one indent unit is removed.
- var-indent-couple-attribute: When looking for unmatched couple openings/closings, only characters with this attribute is considered. The value must be the attribute name from the syntax xml file, for example "Symbol". If it's not specified, attribute 0 is used (usually 'Normal Text').
The idea is to provide a somewhat intelligent indentation for perl, php, bash, scheme and in general formats with humble indentation needs.
Definition at line 492 of file kateautoindent.h.
Member Enumeration Documentation
◆ pairs
enum KateVarIndent::pairs |
Purely for readability, couples we know and love.
Definition at line 500 of file kateautoindent.h.
Member Function Documentation
◆ canProcessLine()
|
inlinevirtual |
Set to true if an actual implementation of 'processLine' is present.
This is used to prevent a needless Undo action from being created.
Reimplemented from KateNormalIndent.
Definition at line 516 of file kateautoindent.h.
◆ modeNumber()
|
inlinevirtual |
Mode index of this mode.
- Returns
- modeNumber
Reimplemented from KateNormalIndent.
Definition at line 518 of file kateautoindent.h.
◆ processChar()
|
virtual |
Called every time a character is inserted into the document.
- Parameters
-
c character inserted
Reimplemented from KateNormalIndent.
Definition at line 2188 of file kateautoindent.cpp.
◆ processLine()
|
virtual |
Aligns/indents the given line to the proper indent position.
Reimplemented from KateNormalIndent.
Definition at line 2204 of file kateautoindent.cpp.
◆ processNewline()
|
virtual |
Called every time a newline character is inserted in the document.
- Parameters
-
cur The position to start processing. Contains the new cursor position after the indention. needContinue Used to determine whether to calculate a continue indent or not.
Reimplemented from KateNormalIndent.
Definition at line 2180 of file kateautoindent.cpp.
◆ processSection()
|
virtual |
Processes a section of text, indenting each line in between.
Reimplemented from KateNormalIndent.
Definition at line 2341 of file kateautoindent.cpp.
The documentation for this class was generated from the following files: