#include <knuminput.h>
Inherits TQSpinBox.
Public Slots | |
virtual void | setValue (double value) |
Signals | |
void | valueChanged (double value) |
Public Member Functions | |
KDoubleSpinBox (TQWidget *parent=0, const char *name=0) | |
KDoubleSpinBox (double lower, double upper, double step, double value, int precision=2, TQWidget *parent=0, const char *name=0) | |
bool | acceptLocalizedNumbers () const |
virtual void | setAcceptLocalizedNumbers (bool accept) |
void | setRange (double lower, double upper, double step=0.01, int precision=2) |
int | precision () const |
void | setPrecision (int precision) |
virtual void | setPrecision (int precision, bool force) |
double | value () const |
double | minValue () const |
void | setMinValue (double value) |
double | maxValue () const |
void | setMaxValue (double value) |
double | lineStep () const |
void | setLineStep (double step) |
void | setValidator (const TQValidator *) |
Protected Slots | |
void | slotValueChanged (int value) |
Protected Member Functions | |
virtual TQString | mapValueToText (int) |
virtual int | mapTextToValue (bool *) |
virtual void | virtual_hook (int id, void *data) |
Detailed Description
A spin box for fractional numbers.
This class provides a spin box for fractional numbers.
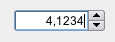
See below for code examples on how to use this class.
Parameters
To make successful use of KDoubleSpinBox, you need to understand the relationship between precision and available range.
- precision: The number of digits after the decimal point.
- maxValue/minValue: upper and lower bounds of the valid range
- lineStep: the size of the step that is made when the user hits the up or down buttons
Since we work with fixed-point numbers internally, the maximum precision is a function of the valid range and vice versa. More precisely, the following relationships hold:
Since the value, bounds and lineStep are rounded to the current precision, you may find that the order of setting these parameters matters. As an example, the following are not equivalent (try it!):
- Version
- $Id$
- Since
- 3.1
Definition at line 838 of file knuminput.h.
Constructor & Destructor Documentation
◆ KDoubleSpinBox() [1/2]
KDoubleSpinBox::KDoubleSpinBox | ( | TQWidget * | parent = 0 , |
const char * | name = 0 |
||
) |
Constructs a KDoubleSpinBox with parent parent
and default values for range and value (whatever QRangeControl uses) and precision (2).
Definition at line 1011 of file knuminput.cpp.
◆ KDoubleSpinBox() [2/2]
KDoubleSpinBox::KDoubleSpinBox | ( | double | lower, |
double | upper, | ||
double | step, | ||
double | value, | ||
int | precision = 2 , |
||
TQWidget * | parent = 0 , |
||
const char * | name = 0 |
||
) |
Constructs a KDoubleSpinBox with parent parent
, range [ lower
, upper
], lineStep step
, precision precision
and initial value value
.
Definition at line 1020 of file knuminput.cpp.
◆ ~KDoubleSpinBox()
|
virtual |
Definition at line 1032 of file knuminput.cpp.
Member Function Documentation
◆ acceptLocalizedNumbers()
bool KDoubleSpinBox::acceptLocalizedNumbers | ( | ) | const |
- Returns
- whether the spinbox uses localized numbers
Definition at line 1036 of file knuminput.cpp.
◆ lineStep()
double KDoubleSpinBox::lineStep | ( | ) | const |
- Returns
- the current step size
Definition at line 1142 of file knuminput.cpp.
◆ mapTextToValue()
|
protectedvirtual |
Definition at line 1162 of file knuminput.cpp.
◆ mapValueToText()
|
protectedvirtual |
Definition at line 1154 of file knuminput.cpp.
◆ maxValue()
double KDoubleSpinBox::maxValue | ( | ) | const |
- Returns
- the current upper bound
Definition at line 1131 of file knuminput.cpp.
◆ minValue()
double KDoubleSpinBox::minValue | ( | ) | const |
- Returns
- the current lower bound
Definition at line 1119 of file knuminput.cpp.
◆ precision()
int KDoubleSpinBox::precision | ( | ) | const |
- Returns
- the current number of digits displayed to the right of the decimal point.
Definition at line 1057 of file knuminput.cpp.
◆ setAcceptLocalizedNumbers()
|
virtual |
Sets whether to use and accept localized numbers as returned by TDELocale::formatNumber()
Definition at line 1042 of file knuminput.cpp.
◆ setLineStep()
void KDoubleSpinBox::setLineStep | ( | double | step | ) |
Sets the step size for clicking the up/down buttons to step
, subject to the constraints that step
is first rounded to the current precision and then clipped to the meaningful interval [ 1, maxValue()
- minValue()
].
Definition at line 1146 of file knuminput.cpp.
◆ setMaxValue()
void KDoubleSpinBox::setMaxValue | ( | double | value | ) |
Sets the upper bound of the range to value
, subject to the contraints that value
is first rounded to the current precision and then clipped to the maximum range interval that can be handled at that precision.
- See also
- minValue, maxValue, setMinValue, setRange
Definition at line 1135 of file knuminput.cpp.
◆ setMinValue()
void KDoubleSpinBox::setMinValue | ( | double | value | ) |
Sets the lower bound of the range to value
, subject to the contraints that value
is first rounded to the current precision and then clipped to the maximum range interval that can be handled at that precision.
- See also
- maxValue, minValue, setMaxValue, setRange
Definition at line 1123 of file knuminput.cpp.
◆ setPrecision() [1/2]
void KDoubleSpinBox::setPrecision | ( | int | precision | ) |
Equivalent to setPrecision( precision
, false
); Needed since Qt's moc doesn't ignore trailing parameters with default args when searching for a property setter method.
Definition at line 1061 of file knuminput.cpp.
◆ setPrecision() [2/2]
|
virtual |
Sets the precision (number of digits to the right of the decimal point).
Note that there is a tradeoff between the precision used and the available range of values. See the class documentation above for more information on this.
- Parameters
-
precision the new precision to use force if true, disables checking of bounds violations that can arise if you increase the precision so much that the minimum and maximum values can't be represented anymore. Disabling is useful if you were going to disable range control in any case.
Definition at line 1065 of file knuminput.cpp.
◆ setRange()
void KDoubleSpinBox::setRange | ( | double | lower, |
double | upper, | ||
double | step = 0.01 , |
||
int | precision = 2 |
||
) |
Sets a new range for the spin box values.
Note that lower
, upper
and step
are rounded to precision
decimal points first.
Definition at line 1047 of file knuminput.cpp.
◆ setValidator()
void KDoubleSpinBox::setValidator | ( | const TQValidator * | ) |
Overridden to ignore any setValidator() calls.
Definition at line 1176 of file knuminput.cpp.
◆ setValue
|
virtualslot |
Sets the current value to value
, subject to the constraints that value
is first rounded to the current precision and then clipped to the interval [ minValue()
, maxValue()
].
Definition at line 1106 of file knuminput.cpp.
◆ slotValueChanged
|
protectedslot |
Definition at line 1180 of file knuminput.cpp.
◆ value()
double KDoubleSpinBox::value | ( | ) | const |
- Returns
- the current value
Definition at line 1102 of file knuminput.cpp.
◆ valueChanged
|
signal |
Emitted whenever TQSpinBox::valueChanged( int ) is emitted.
◆ virtual_hook()
|
protectedvirtual |
Definition at line 1205 of file knuminput.cpp.
The documentation for this class was generated from the following files: