#include <kpanelmenu.h>
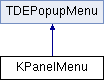
Public Slots | |
void | reinitialize () |
void | deinitialize () |
Public Member Functions | |
KPanelMenu (TQWidget *parent=0, const char *name=0) | |
KPanelMenu (const TQString &startDir, TQWidget *parent=0, const char *name=0) | |
virtual | ~KPanelMenu () |
const TQString & | path () const |
void | setPath (const TQString &p) |
bool | initialized () const |
void | setInitialized (bool on) |
void | disableAutoClear () |
![]() | |
TDEPopupMenu (TQWidget *parent=0, const char *name=0) | |
~TDEPopupMenu () | |
int | insertTitle (const TQString &text, int id=-1, int index=-1) |
int | insertTitle (const TQPixmap &icon, const TQString &text, int id=-1, int index=-1) |
void | changeTitle (int id, const TQString &text) |
void | changeTitle (int id, const TQPixmap &icon, const TQString &text) |
TQString | title (int id=-1) const |
TQPixmap | titlePixmap (int id) const |
void | setKeyboardShortcutsEnabled (bool enable) |
void | setKeyboardShortcutsExecute (bool enable) |
TDEPopupMenu (const TQString &title, TQWidget *parent=0, const char *name=0) TDE_DEPRECATED | |
void | setTitle (const TQString &title) TDE_DEPRECATED |
TQPopupMenu * | contextMenu () |
const TQPopupMenu * | contextMenu () const |
void | hideContextMenu () |
virtual void | activateItemAt (int index) |
TQt::ButtonState | state () const |
Protected Slots | |
virtual void | slotAboutToShow () |
virtual void | slotExec (int id)=0 |
virtual void | initialize ()=0 |
void | slotClear () |
![]() | |
TQString | underlineText (const TQString &text, uint length) |
void | resetKeyboardVars (bool noMatches=false) |
void | itemHighlighted (int whichItem) |
void | showCtxMenu (TQPoint pos) |
void | ctxMenuHiding () |
void | ctxMenuHideShowingMenu () |
Protected Member Functions | |
virtual void | hideEvent (TQHideEvent *ev) |
void | init (const TQString &path=TQString::null) |
virtual void | virtual_hook (int id, void *data) |
![]() | |
virtual void | closeEvent (TQCloseEvent *) |
virtual void | keyPressEvent (TQKeyEvent *e) |
virtual void | mouseReleaseEvent (TQMouseEvent *e) |
virtual void | mousePressEvent (TQMouseEvent *e) |
virtual bool | focusNextPrevChild (bool next) |
virtual void | contextMenuEvent (TQContextMenuEvent *e) |
virtual void | hideEvent (TQHideEvent *) |
virtual void | virtual_hook (int id, void *data) |
Additional Inherited Members | |
![]() | |
void | aboutToShowContextMenu (TDEPopupMenu *menu, int menuItem, TQPopupMenu *ctxMenu) |
![]() | |
static TDEPopupMenu * | contextMenuFocus () |
static int | contextMenuFocusItem () |
Detailed Description
Base class to build dynamically loaded menu entries for the K-menu, or the panel.
This class allows to build menu entries that will be dynamically added either to the K-menu, or to the panel as a normal button. These dynamic menus are located in shared libraries that will be loaded at runtime by Kicker (the TDE panel).
To build such a menu, you have to inherit this class and implement the pure virtual functions initialize() and slotExec(). You also have to provide a factory object in your library, see KLibFactory. This factory is only used to construct the menu object.
Finally, you also have to provide a desktop file describing your dynamic menu. The relevant entries are: Name, Comment, Icon and X-TDE-Library (which contains the library name without any extension). This desktop file has to be installed in $TDEDIR/share/apps/kicker/menuext/.
Definition at line 53 of file kpanelmenu.h.
Constructor & Destructor Documentation
◆ KPanelMenu() [1/2]
KPanelMenu::KPanelMenu | ( | TQWidget * | parent = 0 , |
const char * | name = 0 |
||
) |
Construct a KPanelMenu object.
This is the normal constructor to use when building extrernal menu entries.
Definition at line 49 of file kpanelmenu.cpp.
◆ KPanelMenu() [2/2]
KPanelMenu::KPanelMenu | ( | const TQString & | startDir, |
TQWidget * | parent = 0 , |
||
const char * | name = 0 |
||
) |
Constructor used internally by Kicker.
You don't really want to use it.
- Parameters
-
startDir a directory to associate with this menu parent parent object name name of the object
Definition at line 43 of file kpanelmenu.cpp.
◆ ~KPanelMenu()
|
virtual |
Destructor.
Definition at line 74 of file kpanelmenu.cpp.
Member Function Documentation
◆ deinitialize
|
slot |
Deinitialize the menu: the menu is cleared and the initialized state is set to false.
initialize() is NOT called. It will be called before the menu is next shown, however. Use this slot if you want a delayed reinitialization.
- Since
- 3.1
Definition at line 143 of file kpanelmenu.cpp.
◆ disableAutoClear()
void KPanelMenu::disableAutoClear | ( | ) |
Disable the automatic clearing of the menu.
Kicker uses a cache system for its menus. After a specific configurable delay, the menu will be cleared. Use this function if you want to disable kicker's cache system, and avoid the clearing of your menu.
Definition at line 109 of file kpanelmenu.cpp.
◆ hideEvent()
|
protectedvirtual |
Re-implemented for internal reasons.
Reimplemented from TDEPopupMenu.
Definition at line 98 of file kpanelmenu.cpp.
◆ init()
|
protected |
◆ initialize
|
protectedpure virtualslot |
This slots is called to initialize the menu.
It is called automatically by slotAboutToShow(). By re-implementing this functions, you can reconstruct the menu before it is being shown. At the end of this function, you should call setInitialize() with true to tell the system that the menu is OK. You applet must re-implement this function.
- See also
- slotAboutToShow(), initialized(), setInitialized()
◆ initialized()
bool KPanelMenu::initialized | ( | ) | const |
Tell if the menu has been initialized, that is it already contains items.
This is useful when you need to know if you have to clear the menu, or to fill it.
- Returns
- the initial state
- See also
- setInitialized(), initialize()
Definition at line 124 of file kpanelmenu.cpp.
◆ path()
const TQString & KPanelMenu::path | ( | ) | const |
Get the directory path associated with this menu, or TQString::null if there's no such associated path.
- Returns
- the associated directory path
- See also
- setPath()
Definition at line 114 of file kpanelmenu.cpp.
◆ reinitialize
|
slot |
Reinitialize the menu: the menu is first cleared, the initial state is set to false, and finally initialize() is called.
Use this if you want to refill your menu immediately.
Definition at line 134 of file kpanelmenu.cpp.
◆ setInitialized()
void KPanelMenu::setInitialized | ( | bool | on | ) |
Set the initial state.
Set it to true when you menu is filled with the items you want.
- Parameters
-
on the initial state
- See also
- initialized(), initialize()
Definition at line 129 of file kpanelmenu.cpp.
◆ setPath()
void KPanelMenu::setPath | ( | const TQString & | p | ) |
Set a directory path to be associated with this menu.
- Parameters
-
p the directory path
- See also
- path()
Definition at line 119 of file kpanelmenu.cpp.
◆ slotAboutToShow
|
protectedvirtualslot |
This slot is called just before the menu is shown.
This allows your menu to update itself if needed. However you should instead re-implement initialize to provide this feature. This function is responsible for the cache system handling, so if you re-implement it, you should call the base function also. Calls initialize().
- See also
- disableAutoClear()
Definition at line 79 of file kpanelmenu.cpp.
◆ slotClear
|
protectedslot |
Clears the menu, and update the initial state accordingly.
- See also
- initialized()
Definition at line 92 of file kpanelmenu.cpp.
◆ slotExec
|
protectedpure virtualslot |
This is slot is called when an item from the menu has been selected.
Your applet is then supposed to perform some action. You must re-implement this function.
- Parameters
-
id the ID associated with the selected item
◆ virtual_hook()
|
protectedvirtual |
Reimplemented from TDEPopupMenu.
Definition at line 156 of file kpanelmenu.cpp.
The documentation for this class was generated from the following files: