#include <tdefontcombo.h>
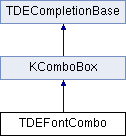
Public Member Functions | |
TDEFontCombo (TQWidget *parent, const char *name=0) | |
TDEFontCombo (const TQStringList &fonts, TQWidget *parent, const char *name=0) | |
virtual | ~TDEFontCombo () |
void | setFonts (const TQStringList &fonts) |
void | setCurrentFont (const TQString &family) |
TQString | currentFont () const |
void | setBold (bool bold) |
bool | bold () const |
void | setItalic (bool italic) |
bool | italic () const |
void | setUnderline (bool underline) |
bool | underline () const |
void | setStrikeOut (bool strikeOut) |
bool | strikeOut () const |
void | setSize (int size) |
int | size () const |
virtual void | setCurrentItem (int i) |
![]() | |
KComboBox (TQWidget *parent=0, const char *name=0) | |
KComboBox (bool rw, TQWidget *parent=0, const char *name=0) | |
virtual | ~KComboBox () |
void | setEditURL (const KURL &url) |
void | insertURL (const KURL &url, int index=-1) |
void | insertURL (const TQPixmap &pixmap, const KURL &url, int index=-1) |
void | changeURL (const KURL &url, int index) |
void | changeURL (const TQPixmap &pixmap, const KURL &url, int index) |
int | cursorPosition () const |
virtual void | setAutoCompletion (bool autocomplete) |
bool | autoCompletion () const |
virtual void | setContextMenuEnabled (bool showMenu) |
bool | isContextMenuEnabled () const |
void | setURLDropsEnabled (bool enable) |
bool | isURLDropsEnabled () const |
bool | contains (const TQString &text) const |
void | setTrapReturnKey (bool trap) |
bool | trapReturnKey () const |
virtual bool | eventFilter (TQObject *, TQEvent *) |
TDECompletionBox * | completionBox (bool create=true) |
virtual void | setLineEdit (TQLineEdit *) |
![]() | |
TDECompletion * | completionObject (bool hsig=true) |
virtual void | setCompletionObject (TDECompletion *compObj, bool hsig=true) |
virtual void | setHandleSignals (bool handle) |
bool | isCompletionObjectAutoDeleted () const |
void | setAutoDeleteCompletionObject (bool autoDelete) |
void | setEnableSignals (bool enable) |
bool | handleSignals () const |
bool | emitSignals () const |
virtual void | setCompletionMode (TDEGlobalSettings::Completion mode) |
TDEGlobalSettings::Completion | completionMode () const |
bool | setKeyBinding (KeyBindingType item, const TDEShortcut &key) |
const TDEShortcut & | getKeyBinding (KeyBindingType item) const |
void | useGlobalKeyBindings () |
virtual void | setCompletedText (const TQString &text)=0 |
virtual void | setCompletedItems (const TQStringList &items)=0 |
TDECompletion * | compObj () const |
Static Public Member Functions | |
static bool | displayFonts () |
Protected Slots | |
void | slotModified (int i) |
![]() | |
virtual void | itemSelected (TQListBoxItem *) |
virtual void | makeCompletion (const TQString &) |
Protected Member Functions | |
void | updateFonts () |
virtual void | virtual_hook (int id, void *data) |
![]() | |
virtual void | setCompletedText (const TQString &, bool) |
virtual void | create (WId=0, bool initializeWindow=true, bool destroyOldWindow=true) |
virtual void | wheelEvent (TQWheelEvent *ev) |
virtual void | virtual_hook (int id, void *data) |
![]() | |
KeyBindingMap | getKeyBindings () const |
void | setDelegate (TDECompletionBase *delegate) |
TDECompletionBase * | delegate () const |
Friends | |
class | TDEFontListItem |
Additional Inherited Members | |
![]() | |
enum | KeyBindingType |
![]() | |
void | rotateText (TDECompletionBase::KeyBindingType type) |
virtual void | setCompletedText (const TQString &) |
void | setCompletedItems (const TQStringList &items) |
void | setCurrentItem (const TQString &item, bool insert=false, int index=-1) |
void | setCurrentItem (int index) |
![]() | |
void | returnPressed () |
void | returnPressed (const TQString &) |
void | completion (const TQString &) |
void | substringCompletion (const TQString &) |
void | textRotation (TDECompletionBase::KeyBindingType) |
void | completionModeChanged (TDEGlobalSettings::Completion) |
void | aboutToShowContextMenu (TQPopupMenu *p) |
![]() | |
TextCompletion | |
PrevCompletionMatch | |
NextCompletionMatch | |
SubstringCompletion | |
Detailed Description
A combobox that lists the available fonts.
The items are painted using the respective font itself, so the user can easily choose a font based on its look. This can be turned off globally if the user wishes so.
Definition at line 35 of file tdefontcombo.h.
Constructor & Destructor Documentation
◆ TDEFontCombo() [1/2]
TDEFontCombo::TDEFontCombo | ( | TQWidget * | parent, |
const char * | name = 0 |
||
) |
Constructor.
- Parameters
-
parent The parent widget name The object name for the widget
Definition at line 175 of file tdefontcombo.cpp.
◆ TDEFontCombo() [2/2]
TDEFontCombo::TDEFontCombo | ( | const TQStringList & | fonts, |
TQWidget * | parent, | ||
const char * | name = 0 |
||
) |
Constructor that takes an already initialzed font list.
- Parameters
-
fonts A list of fonts to show parent The parent widget name The object name for the widget
Definition at line 184 of file tdefontcombo.cpp.
◆ ~TDEFontCombo()
|
virtual |
Destructor.
Definition at line 289 of file tdefontcombo.cpp.
Member Function Documentation
◆ bold()
bool TDEFontCombo::bold | ( | ) | const |
Returns the current bold status.
- Returns
- true if fonts are bold
Definition at line 302 of file tdefontcombo.cpp.
◆ currentFont()
TQString TDEFontCombo::currentFont | ( | ) | const |
- Returns
- the currently selected font.
Definition at line 266 of file tdefontcombo.cpp.
◆ displayFonts()
|
static |
Returns the user's setting of whether the items should be painted in the respective fonts or not.
- Returns
- True if the respective fonts are used for painting
Definition at line 375 of file tdefontcombo.cpp.
◆ italic()
bool TDEFontCombo::italic | ( | ) | const |
Returns the current italic status.
- Returns
- True if fonts are italic
Definition at line 315 of file tdefontcombo.cpp.
◆ setBold()
void TDEFontCombo::setBold | ( | bool | bold | ) |
Sets the listed fonts to bold or normal.
- Parameters
-
bold Set to true to display fonts in bold
Definition at line 294 of file tdefontcombo.cpp.
◆ setCurrentFont()
void TDEFontCombo::setCurrentFont | ( | const TQString & | family | ) |
Sets the currently selected font.
- Parameters
-
family Font to select.
Definition at line 201 of file tdefontcombo.cpp.
◆ setCurrentItem()
|
virtual |
Definition at line 273 of file tdefontcombo.cpp.
◆ setFonts()
void TDEFontCombo::setFonts | ( | const TQStringList & | fonts | ) |
Sets the font list.
- Parameters
-
fonts Font list to show
Definition at line 191 of file tdefontcombo.cpp.
◆ setItalic()
void TDEFontCombo::setItalic | ( | bool | italic | ) |
Sets the listed fonts to italic or regular.
- Parameters
-
italic Set to true to display fonts italic
Definition at line 307 of file tdefontcombo.cpp.
◆ setSize()
void TDEFontCombo::setSize | ( | int | size | ) |
Sets the listed fonts' size.
- Parameters
-
size Set to the point size to display the fonts in
Definition at line 346 of file tdefontcombo.cpp.
◆ setStrikeOut()
void TDEFontCombo::setStrikeOut | ( | bool | strikeOut | ) |
Sets the listed fonts to striked out or not.
- Parameters
-
strikeOut Set to true to display fonts striked out
Definition at line 333 of file tdefontcombo.cpp.
◆ setUnderline()
void TDEFontCombo::setUnderline | ( | bool | underline | ) |
Sets the listed fonts to underlined or not underlined.
- Parameters
-
underline Set to true to display fonts underlined
Definition at line 320 of file tdefontcombo.cpp.
◆ size()
int TDEFontCombo::size | ( | ) | const |
Returns the current font size.
- Returns
- The point size of the fonts
Definition at line 358 of file tdefontcombo.cpp.
◆ slotModified
|
protectedslot |
Listens to highlighted(int)
Definition at line 261 of file tdefontcombo.cpp.
◆ strikeOut()
bool TDEFontCombo::strikeOut | ( | ) | const |
Returns the current strike out status.
- Returns
- True if fonts are striked out
Definition at line 341 of file tdefontcombo.cpp.
◆ underline()
bool TDEFontCombo::underline | ( | ) | const |
Returns the current underline status.
- Returns
- True if fonts are underlined
Definition at line 328 of file tdefontcombo.cpp.
◆ updateFonts()
|
protected |
Updated the combo's listBox() to reflect changes made to the fonts' attributed.
Definition at line 363 of file tdefontcombo.cpp.
◆ virtual_hook()
|
protectedvirtual |
Reimplemented from KComboBox.
Definition at line 381 of file tdefontcombo.cpp.
Friends And Related Function Documentation
◆ TDEFontListItem
|
friend |
Definition at line 171 of file tdefontcombo.h.
The documentation for this class was generated from the following files: