#include <tdewallet.h>
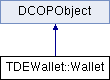
Public Types | |
enum | EntryType { Unknown =0 , Password , Stream , Map , Unused =0xffff } |
enum | OpenType { Synchronous =0 , Asynchronous , Path , OpenTypeUnused =0xff } |
Signals | |
void | walletClosed () |
void | folderUpdated (const TQString &folder) |
void | folderListUpdated () |
void | folderRemoved (const TQString &folder) |
void | walletOpened (bool success) |
Public Member Functions | |
virtual | ~Wallet () |
virtual int | sync () |
virtual int | lockWallet () |
virtual const TQString & | walletName () const |
virtual bool | isOpen () const |
virtual void | requestChangePassword (WId w=0) |
virtual TQStringList | folderList () |
virtual bool | hasFolder (const TQString &f) |
virtual bool | setFolder (const TQString &f) |
virtual bool | removeFolder (const TQString &f) |
virtual bool | createFolder (const TQString &f) |
virtual const TQString & | currentFolder () const |
virtual TQStringList | entryList () |
virtual int | renameEntry (const TQString &oldName, const TQString &newName) |
virtual int | readEntry (const TQString &key, TQByteArray &value) |
virtual int | readMap (const TQString &key, TQMap< TQString, TQString > &value) |
virtual int | readPassword (const TQString &key, TQString &value) |
int | readEntryList (const TQString &key, TQMap< TQString, TQByteArray > &value) |
int | readMapList (const TQString &key, TQMap< TQString, TQMap< TQString, TQString > > &value) |
int | readPasswordList (const TQString &key, TQMap< TQString, TQString > &value) |
virtual int | writeEntry (const TQString &key, const TQByteArray &value, EntryType entryType) |
virtual int | writeEntry (const TQString &key, const TQByteArray &value) |
virtual int | writeMap (const TQString &key, const TQMap< TQString, TQString > &value) |
virtual int | writePassword (const TQString &key, const TQString &value) |
virtual bool | hasEntry (const TQString &key) |
virtual int | removeEntry (const TQString &key) |
virtual EntryType | entryType (const TQString &key) |
![]() | |
DCOPObject (TQObject *obj) | |
DCOPObject (const TQCString &objId) | |
TQCString | objId () const |
bool | setObjId (const TQCString &objId) |
virtual bool | process (const TQCString &fun, const TQByteArray &data, TQCString &replyType, TQByteArray &replyData) |
virtual bool | processDynamic (const TQCString &fun, const TQByteArray &data, TQCString &replyType, TQByteArray &replyData) |
virtual QCStringList | functionsDynamic () |
virtual QCStringList | interfacesDynamic () |
virtual QCStringList | interfaces () |
virtual QCStringList | functions () |
void | emitDCOPSignal (const TQCString &signal, const TQByteArray &data) |
bool | connectDCOPSignal (const TQCString &sender, const TQCString &senderObj, const TQCString &signal, const TQCString &slot, bool Volatile) |
bool | disconnectDCOPSignal (const TQCString &sender, const TQCString &senderObj, const TQCString &signal, const TQCString &slot) |
DCOPClient * | callingDcopClient () |
Static Public Member Functions | |
static TQStringList | walletList () |
static bool | isEnabled () |
static bool | isOpen (const TQString &name) |
static int | closeWallet (const TQString &name, bool force) |
static int | deleteWallet (const TQString &name) |
static bool | disconnectApplication (const TQString &wallet, const TQCString &app) |
static Wallet * | openWallet (const TQString &name, WId w=0, OpenType ot=Synchronous) |
static TQStringList | users (const TQString &wallet) |
static const TQString | LocalWallet () |
static const TQString | NetworkWallet () |
static const TQString | PasswordFolder () |
static const TQString | FormDataFolder () |
static void | changePassword (const TQString &name, WId w=0) |
static bool | folderDoesNotExist (const TQString &wallet, const TQString &folder) |
static bool | keyDoesNotExist (const TQString &wallet, const TQString &folder, const TQString &key) |
![]() | |
static bool | hasObject (const TQCString &objId) |
static DCOPObject * | find (const TQCString &objId) |
static TQPtrList< DCOPObject > | match (const TQCString &partialId) |
static TQCString | objectName (TQObject *obj) |
Protected Member Functions | |
Wallet (int handle, const TQString &name) | |
Wallet (const Wallet &) | |
virtual void | virtual_hook (int id, void *data) |
Detailed Description
KDE Wallet.
This class implements a generic system-wide Wallet for KDE. This is the ONLY public interface. The DCOP client is unsupported and considered to be private.
KDE Wallet Class
Definition at line 49 of file tdewallet.h.
Member Enumeration Documentation
◆ EntryType
enum TDEWallet::Wallet::EntryType |
Definition at line 67 of file tdewallet.h.
◆ OpenType
enum TDEWallet::Wallet::OpenType |
Definition at line 122 of file tdewallet.h.
Constructor & Destructor Documentation
◆ Wallet() [1/2]
|
protected |
Construct a TDEWallet object.
Definition at line 75 of file tdewallet.cpp.
◆ Wallet() [2/2]
◆ ~Wallet()
|
virtual |
Member Function Documentation
◆ changePassword()
|
static |
Request to the wallet service to change the password of the wallet name
.
- Parameters
-
name The the wallet to change the password of. w The window id to associate any dialogs with.
Definition at line 129 of file tdewallet.cpp.
◆ closeWallet()
|
static |
Close the wallet name
.
The wallet will only be closed if it is open but not in use (rare), or if it is forced closed.
- Parameters
-
name The name of the wallet to close. force Set true to force the wallet closed even if it is in use by others.
- Returns
- Returns 0 on success, non-zero on error.
Definition at line 154 of file tdewallet.cpp.
◆ createFolder()
|
virtual |
Created the folder f
.
- Parameters
-
f the name of the folder to create
- Returns
- Returns true if the folder was successfully created.
Definition at line 331 of file tdewallet.cpp.
◆ currentFolder()
|
virtual |
Determine the current working folder in the wallet.
If the folder name is empty, it is working in the global folder, which is valid but discouraged.
- Returns
- Returns the current working folder.
Definition at line 392 of file tdewallet.cpp.
◆ deleteWallet()
|
static |
Delete the wallet name
.
The wallet will be forced closed first.
- Parameters
-
name The name of the wallet to delete.
- Returns
- Returns 0 on success, non-zero on error.
Definition at line 164 of file tdewallet.cpp.
◆ disconnectApplication()
|
static |
Disconnect the application app
from wallet
.
- Parameters
-
wallet The name of the wallet to disconnect. app The name of the application to disconnect.
- Returns
- Returns true on success, false on error.
Definition at line 206 of file tdewallet.cpp.
◆ entryList()
|
virtual |
Return the list of keys of all entries in this folder.
- Returns
- Returns an empty list if the wallet is not open, or if the folder is empty.
Definition at line 299 of file tdewallet.cpp.
◆ entryType()
|
virtual |
Determine the type of the entry key
in this folder.
- Parameters
-
key The key to look up.
- Returns
- Returns an enumerated type representing the type of the entry.
Definition at line 628 of file tdewallet.cpp.
◆ folderDoesNotExist()
|
static |
Determine if a folder does not exist in a wallet.
This does not require decryption of the wallet. This is a handy optimization to avoid prompting the user if your data is certainly not in the wallet.
- Parameters
-
wallet The wallet to look in. folder The folder to look up.
- Returns
- Returns true if the folder does NOT exist in the wallet, or the wallet does not exist.
Definition at line 689 of file tdewallet.cpp.
◆ folderList()
|
virtual |
Obtain the list of all folders contained in the wallet.
- Returns
- Returns an empty list if the wallet is not open.
Definition at line 283 of file tdewallet.cpp.
◆ folderListUpdated
|
signal |
Emitted when the folder list is changed in this wallet.
◆ folderRemoved
|
signal |
Emitted when a folder in this wallet is removed.
- Parameters
-
folder The folder that was removed.
◆ folderUpdated
|
signal |
Emitted when a folder in this wallet is updated.
- Parameters
-
folder The folder that was updated.
◆ FormDataFolder()
|
static |
The standardized name of the form data folder.
It is automatically created when a wallet is created, but the user may still delete it so you should check for its existence and recreate it if necessary and desired.
Definition at line 69 of file tdewallet.cpp.
◆ hasEntry()
|
virtual |
Determine if the current folder has they entry key
.
- Parameters
-
key The key to search for.
- Returns
- Returns true if the folder contains
key
.
Definition at line 596 of file tdewallet.cpp.
◆ hasFolder()
|
virtual |
Determine if the folder f
exists in the wallet.
- Parameters
-
f the name of the folder to check for
- Returns
- Returns true if the folder exists in the wallet.
Definition at line 315 of file tdewallet.cpp.
◆ isEnabled()
|
static |
Determine if the KDE wallet is enabled.
Normally you do not need to use this because open() will just fail.
- Returns
- Returns true if the wallet enabled, else false.
Definition at line 134 of file tdewallet.cpp.
◆ isOpen() [1/2]
|
virtual |
Determine if the current wallet is open, and is a valid wallet handle.
- Returns
- Returns true if the wallet handle is valid and open.
Definition at line 259 of file tdewallet.cpp.
◆ isOpen() [2/2]
|
static |
Determine if the wallet name
is open by any application.
- Parameters
-
name The name of the wallet to check.
- Returns
- Returns true if the wallet is open, else false.
Definition at line 144 of file tdewallet.cpp.
◆ keyDoesNotExist()
|
static |
Determine if an entry in a folder does not exist in a wallet.
This does not require decryption of the wallet. This is a handy optimization to avoid prompting the user if your data is certainly not in the wallet.
- Parameters
-
wallet The wallet to look in. folder The folder to look in. key The key to look up.
- Returns
- Returns true if the key does NOT exist in the wallet, or the folder or wallet does not exist.
Definition at line 699 of file tdewallet.cpp.
◆ LocalWallet()
|
static |
The name of the wallet used to store local passwords.
Definition at line 36 of file tdewallet.cpp.
◆ lockWallet()
|
virtual |
This closes and locks the current wallet.
It will disconnect all applications using the wallet.
- Returns
- Returns 0 on success, non-zero on error.
Definition at line 236 of file tdewallet.cpp.
◆ NetworkWallet()
|
static |
The name of the wallet used to store network passwords.
Definition at line 54 of file tdewallet.cpp.
◆ openWallet()
|
static |
Open the wallet name
.
The user will be prompted to allow your application to open the wallet, and may be prompted for a password. You are responsible for deleting this object when you are done with it.
- Parameters
-
name The name of the wallet to open. ot If Asynchronous, the call will return immediately with a non-null pointer to an invalid wallet. You must immediately connect the walletOpened() signal to a slot so that you will know when it is opened, or when it fails. w The window id to associate any dialogs with.
- Returns
- Returns a pointer to the wallet if successful, or a null pointer on error or if rejected.
Definition at line 174 of file tdewallet.cpp.
◆ PasswordFolder()
|
static |
The standardized name of the password folder.
It is automatically created when a wallet is created, but the user may still delete it so you should check for its existence and recreate it if necessary and desired.
Definition at line 65 of file tdewallet.cpp.
◆ readEntry()
|
virtual |
Read the entry key
from the current folder.
The entry format is unknown except that it is either a TQByteArray or a TQDataStream, which effectively means that it is anything.
- Parameters
-
key The key of the entry to read. value A buffer to fill with the value.
- Returns
- Returns 0 on success, non-zero on error.
Definition at line 397 of file tdewallet.cpp.
◆ readEntryList()
int Wallet::readEntryList | ( | const TQString & | key, |
TQMap< TQString, TQByteArray > & | value | ||
) |
Read the entries matching key
from the current folder.
The entry format is unknown except that it is either a TQByteArray or a TQDataStream, which effectively means that it is anything.
- Parameters
-
key The key of the entry to read. Wildcards are supported. value A buffer to fill with the value. The key in the map is the entry key.
- Returns
- Returns 0 on success, non-zero on error.
- Since
- 3.4
Definition at line 414 of file tdewallet.cpp.
◆ readMap()
|
virtual |
Read the map entry key
from the current folder.
- Parameters
-
key The key of the entry to read. value A map buffer to fill with the value.
- Returns
- Returns 0 on success, non-zero on error. Will return an error if the key was not originally written as a map.
Definition at line 447 of file tdewallet.cpp.
◆ readMapList()
int Wallet::readMapList | ( | const TQString & | key, |
TQMap< TQString, TQMap< TQString, TQString > > & | value | ||
) |
Read the map entry key
from the current folder.
- Parameters
-
key The key of the entry to read. Wildcards are supported. value A buffer to fill with the value. The key in the map is the entry key.
- Returns
- Returns 0 on success, non-zero on error. Will return an error if the key was not originally written as a map.
- Since
- 3.4
Definition at line 469 of file tdewallet.cpp.
◆ readPassword()
|
virtual |
Read the password entry key
from the current folder.
- Parameters
-
key The key of the entry to read. value A password buffer to fill with the value.
- Returns
- Returns 0 on success, non-zero on error. Will return an error if the key was not originally written as a password.
Definition at line 495 of file tdewallet.cpp.
◆ readPasswordList()
int Wallet::readPasswordList | ( | const TQString & | key, |
TQMap< TQString, TQString > & | value | ||
) |
Read the password entry key
from the current folder.
- Parameters
-
key The key of the entry to read. Wildcards are supported. value A buffer to fill with the value. The key in the map is the entry key.
- Returns
- Returns 0 on success, non-zero on error. Will return an error if the key was not originally written as a password.
- Since
- 3.4
Definition at line 512 of file tdewallet.cpp.
◆ removeEntry()
|
virtual |
Remove the entry key
from the current folder.
- Parameters
-
key The key to remove.
- Returns
- Returns 0 on success, non-zero on error.
Definition at line 612 of file tdewallet.cpp.
◆ removeFolder()
|
virtual |
Remove the folder f
and all its entries from the wallet.
- Parameters
-
f the name of the folder to remove
- Returns
- Returns true if the folder was successfully removed.
Definition at line 372 of file tdewallet.cpp.
◆ renameEntry()
|
virtual |
Rename the entry oldName
to newName
.
- Parameters
-
oldName The original key of the entry. newName The new key of the entry.
- Returns
- Returns 0 on success, non-zero on error.
Definition at line 431 of file tdewallet.cpp.
◆ requestChangePassword()
|
virtual |
Request to the wallet service to change the password of the current wallet.
- Parameters
-
w The window id to associate any dialogs with.
Definition at line 264 of file tdewallet.cpp.
◆ setFolder()
|
virtual |
Set the current working folder to f
.
The folder must exist, or this call will fail. Create a folder with createFolder().
- Parameters
-
f the name of the folder to make the working folder
- Returns
- Returns true if the folder was successfully set.
Definition at line 349 of file tdewallet.cpp.
◆ slotApplicationDisconnected
void Wallet::slotApplicationDisconnected | ( | const TQString & | wallet, |
const TQCString & | application | ||
) |
Definition at line 665 of file tdewallet.cpp.
◆ slotFolderListUpdated
void Wallet::slotFolderListUpdated | ( | const TQString & | wallet | ) |
Definition at line 658 of file tdewallet.cpp.
◆ slotFolderUpdated
void Wallet::slotFolderUpdated | ( | const TQString & | wallet, |
const TQString & | folder | ||
) |
Definition at line 651 of file tdewallet.cpp.
◆ slotWalletClosed
void Wallet::slotWalletClosed | ( | int | handle | ) |
Definition at line 273 of file tdewallet.cpp.
◆ sync()
|
virtual |
This syncs the wallet file on disk with what is in memory.
You don't normally need to use this. It happens automatically on close.
- Returns
- Returns 0 on success, non-zero on error.
Definition at line 226 of file tdewallet.cpp.
◆ users()
|
static |
List the applications that are using the wallet wallet
.
- Parameters
-
wallet The wallet to query.
- Returns
- Returns a list of all DCOP application IDs using the wallet.
Definition at line 216 of file tdewallet.cpp.
◆ virtual_hook()
|
protectedvirtual |
Definition at line 709 of file tdewallet.cpp.
◆ walletClosed
|
signal |
Emitted when this wallet is closed.
◆ walletList()
|
static |
List all the wallets available.
- Returns
- Returns a list of the names of all wallets that are open.
Definition at line 119 of file tdewallet.cpp.
◆ walletName()
|
virtual |
The name of the current wallet.
Definition at line 254 of file tdewallet.cpp.
◆ walletOpened
|
signal |
Emitted when a wallet is opened in asynchronous mode.
- Parameters
-
success True if the wallet was opened successfully.
◆ walletOpenResult
void Wallet::walletOpenResult | ( | int | rc | ) |
Definition at line 674 of file tdewallet.cpp.
◆ writeEntry() [1/2]
|
virtual |
Write key
= value
as a binary entry to the current folder.
- Parameters
-
key The key of the new entry. value The value of the entry.
- Returns
- Returns 0 on success, non-zero on error.
Definition at line 545 of file tdewallet.cpp.
◆ writeEntry() [2/2]
|
virtual |
Write key
= value
as a binary entry to the current folder.
Be careful with this, it could cause inconsistency in the future since you can put an arbitrary entry type in place.
- Parameters
-
key The key of the new entry. value The value of the entry. entryType The type of the entry.
- Returns
- Returns 0 on success, non-zero on error.
Definition at line 529 of file tdewallet.cpp.
◆ writeMap()
|
virtual |
Write key
= value
as a map to the current folder.
- Parameters
-
key The key of the new entry. value The value of the map.
- Returns
- Returns 0 on success, non-zero on error.
Definition at line 561 of file tdewallet.cpp.
◆ writePassword()
|
virtual |
Write key
= value
as a password to the current folder.
- Parameters
-
key The key of the new entry. value The value of the password.
- Returns
- Returns 0 on success, non-zero on error.
Definition at line 580 of file tdewallet.cpp.
The documentation for this class was generated from the following files: