#include <kcommondecoration.h>
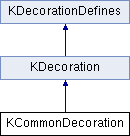
Public Types | |
enum | LayoutMetric { LM_BorderLeft , LM_BorderRight , LM_BorderBottom , LM_TitleHeight , LM_TitleBorderLeft , LM_TitleBorderRight , LM_TitleEdgeLeft , LM_TitleEdgeRight , LM_TitleEdgeTop , LM_TitleEdgeBottom , LM_ButtonWidth , LM_ButtonHeight , LM_ButtonSpacing , LM_ExplicitButtonSpacer , LM_ButtonMarginTop , LM_RightButtonsMarginTop } |
enum | DecorationBehaviour { DB_MenuClose , DB_WindowMask , DB_ButtonHide } |
enum | WindowCorner { WC_TopLeft , WC_TopRight , WC_BottomLeft , WC_BottomRight } |
![]() | |
enum | Position { PositionCenter = 0x00 , PositionLeft = 0x01 , PositionRight = 0x02 , PositionTop = 0x04 , PositionBottom = 0x08 , PositionTopLeft = PositionLeft | PositionTop , PositionTopRight = PositionRight | PositionTop , PositionBottomLeft = PositionLeft | PositionBottom , PositionBottomRight = PositionRight | PositionBottom } |
enum | MaximizeMode { MaximizeRestore = 0 , MaximizeVertical = 1 , MaximizeHorizontal = 2 , MaximizeFull = MaximizeVertical | MaximizeHorizontal } |
enum | WindowOperation { MaximizeOp = 5000 , RestoreOp , MinimizeOp , MoveOp , UnrestrictedMoveOp , ResizeOp , UnrestrictedResizeOp , CloseOp , OnAllDesktopsOp , ShadeOp , KeepAboveOp , KeepBelowOp , OperationsOp , WindowRulesOp , ToggleStoreSettingsOp = WindowRulesOp , HMaximizeOp , VMaximizeOp , LowerOp , FullScreenOp , NoBorderOp , NoOp , SetupWindowShortcutOp , ApplicationRulesOp , ShadowOp , SuspendWindowOp , ResumeWindowOp } |
enum | ColorType { ColorTitleBar , ColorTitleBlend , ColorFont , ColorButtonBg , ColorFrame , ColorHandle , NUM_COLORS } |
enum | { SettingDecoration = 1 << 0 , SettingColors = 1 << 1 , SettingFont = 1 << 2 , SettingButtons = 1 << 3 , SettingTooltips = 1 << 4 , SettingBorder = 1 << 5 } |
enum | BorderSize { BorderTiny , BorderNormal , BorderLarge , BorderVeryLarge , BorderHuge , BorderVeryHuge , BorderOversized , BordersCount } |
enum | Ability { AbilityAnnounceButtons = 0 , AbilityButtonMenu = 1000 , AbilityButtonOnAllDesktops = 1001 , AbilityButtonSpacer = 1002 , AbilityButtonHelp = 1003 , AbilityButtonMinimize = 1004 , AbilityButtonMaximize = 1005 , AbilityButtonClose = 1006 , AbilityButtonAboveOthers = 1007 , AbilityButtonBelowOthers = 1008 , AbilityButtonShade = 1009 , AbilityButtonResize = 1010 , ABILITY_DUMMY = 10000000 } |
enum | Requirement { REQUIREMENT_DUMMY = 1000000 } |
Public Slots | |
void | keepAboveChange (bool above) |
void | keepBelowChange (bool below) |
void | slotMaximize () |
void | slotShade () |
void | slotKeepAbove () |
void | slotKeepBelow () |
void | menuButtonPressed () |
void | menuButtonReleased () |
![]() | |
void | closeWindow () |
void | maximize (ButtonState button) |
void | maximize (MaximizeMode mode) |
void | minimize () |
void | showContextHelp () |
void | setDesktop (int desktop) |
void | toggleOnAllDesktops () |
void | titlebarDblClickOperation () |
void | titlebarMouseWheelOperation (int delta) |
void | setShade (bool set) |
void | setKeepAbove (bool set) |
void | setKeepBelow (bool set) |
void | emitKeepAboveChanged (bool above) |
void | emitKeepBelowChanged (bool below) |
Public Member Functions | |
KCommonDecoration (KDecorationBridge *bridge, KDecorationFactory *factory) | |
virtual TQString | visibleName () const =0 |
virtual TQString | defaultButtonsLeft () const =0 |
virtual TQString | defaultButtonsRight () const =0 |
virtual bool | decorationBehaviour (DecorationBehaviour behaviour) const |
virtual int | layoutMetric (LayoutMetric lm, bool respectWindowState=true, const KCommonDecorationButton *button=0) const |
virtual KCommonDecorationButton * | createButton (ButtonType type)=0 |
virtual TQRegion | cornerShape (WindowCorner corner) |
virtual void | updateWindowShape () |
virtual void | paintEvent (TQPaintEvent *e)=0 |
virtual void | updateCaption () |
int | buttonsLeftWidth () const |
int | buttonsRightWidth () const |
void | updateLayout () const |
void | updateButtons () const |
void | resetButtons () const |
bool | isToolWindow () const |
TQRect | titleRect () const |
virtual void | init () |
virtual void | reset (unsigned long changed) |
virtual void | borders (int &left, int &right, int &top, int &bottom) const |
virtual void | show () |
virtual void | resize (const TQSize &s) |
virtual TQSize | minimumSize () const |
virtual void | maximizeChange () |
virtual void | desktopChange () |
virtual void | shadeChange () |
virtual void | iconChange () |
virtual void | activeChange () |
virtual void | captionChange () |
virtual Position | mousePosition (const TQPoint &point) const |
virtual bool | eventFilter (TQObject *o, TQEvent *e) |
virtual void | resizeEvent (TQResizeEvent *e) |
virtual void | mouseDoubleClickEvent (TQMouseEvent *e) |
virtual void | wheelEvent (TQWheelEvent *e) |
![]() | |
KDecoration (KDecorationBridge *bridge, KDecorationFactory *factory) | |
virtual | ~KDecoration () |
bool | isActive () const |
bool | isCloseable () const |
bool | isMaximizable () const |
MaximizeMode | maximizeMode () const |
bool | isMinimizable () const |
bool | providesContextHelp () const |
int | desktop () const |
bool | isOnAllDesktops () const |
bool | isModal () const |
bool | isShadeable () const |
bool | isShade () const |
bool | isSetShade () const |
bool | keepAbove () const |
bool | keepBelow () const |
bool | isMovable () const |
bool | isResizable () const |
NET::WindowType | windowType (unsigned long supported_types) const |
TQIconSet | icon () const |
TQString | caption () const |
void | showWindowMenu (const TQRect &pos) |
void | showWindowMenu (TQPoint pos) |
void | performWindowOperation (WindowOperation op) |
void | setMask (const TQRegion ®, int mode=0) |
void | clearMask () |
bool | isPreview () const |
TQRect | geometry () const |
TQRect | iconGeometry () const |
TQRegion | unobscuredRegion (const TQRegion &r) const |
TQWidget * | workspaceWidget () const |
WId | windowId () const |
int | width () const |
int | height () const |
void | processMousePressEvent (TQMouseEvent *e) |
virtual void | init ()=0 |
virtual Position | mousePosition (const TQPoint &p) const =0 |
virtual void | borders (int &left, int &right, int &top, int &bottom) const =0 |
virtual void | resize (const TQSize &s)=0 |
virtual TQSize | minimumSize () const =0 |
virtual void | activeChange ()=0 |
virtual void | captionChange ()=0 |
virtual void | iconChange ()=0 |
virtual void | maximizeChange ()=0 |
virtual void | desktopChange ()=0 |
virtual void | shadeChange ()=0 |
virtual bool | drawbound (const TQRect &geom, bool clear) |
virtual bool | animateMinimize (bool minimize) |
virtual bool | windowDocked (Position side) |
virtual void | reset (unsigned long changed) |
void | setMainWidget (TQWidget *) |
void | createMainWidget (TQt::WFlags flags=0) |
TQWidget * | initialParentWidget () const |
TQt::WFlags | initialWFlags () const |
void | helperShowHide (bool show) |
TQWidget * | widget () |
const TQWidget * | widget () const |
KDecorationFactory * | factory () const |
void | grabXServer () |
void | ungrabXServer () |
Additional Inherited Members | |
![]() | |
void | keepAboveChanged (bool) |
void | keepBelowChanged (bool) |
![]() | |
static const KDecorationOptions * | options () |
Detailed Description
This class eases development of decorations by implementing parts of KDecoration which are error prone and common for most decorations.
It takes care of the window layout, button/action handling, and window mask creation.
Definition at line 59 of file kcommondecoration.h.
Member Enumeration Documentation
◆ DecorationBehaviour
Enumerator | |
---|---|
DB_MenuClose | Close window on double clicking the menu. |
DB_WindowMask | Set a mask on the window. |
DB_ButtonHide | Hide buttons when there is not enough space in the titlebar. |
Definition at line 120 of file kcommondecoration.h.
◆ LayoutMetric
Used to calculate the decoration layout.
The basic layout looks like this:
Window:
| LM_TitleEdgeTop | |_______________________________________________________________| | LM_TitleEdgeLeft | [title] | LM_TitleEdgeRight | |__________________|________________________|___________________| | LM_TitleEdgeBottom | |_______________________________________________________________|
|LM_BorderLeft LM_BorderRight| |_|___________________________________________________________|_| | LM_BorderBottom | |_______________________________________________________________|
Title:
| LM_ButtonMarginTop | | LM_ButtonMarginTop | |________________________________| |_________________________________| | [Buttons] | LM_TitleBorderLeft | LM_TitleHeight | LM_TitleBorderRight | [Buttons] | |___________|____________________|________________|_____________________|___________|
Buttons:
| button | spacing | button | spacing | explicit spacer | spacing | ... | spacing | button | |________|_________|________|_________|_________________|_________|________|_________|________|
- See also
- layoutMetric()
Definition at line 100 of file kcommondecoration.h.
◆ WindowCorner
enum KCommonDecoration::WindowCorner |
Definition at line 127 of file kcommondecoration.h.
Constructor & Destructor Documentation
◆ KCommonDecoration()
KCommonDecoration::KCommonDecoration | ( | KDecorationBridge * | bridge, |
KDecorationFactory * | factory | ||
) |
Definition at line 44 of file kcommondecoration.cpp.
◆ ~KCommonDecoration()
|
virtual |
Definition at line 55 of file kcommondecoration.cpp.
Member Function Documentation
◆ activeChange()
|
virtual |
This function is called whenever the window either becomes or stops being active.
Use isActive() to find out the current state.
Implements KDecoration.
Definition at line 581 of file kcommondecoration.cpp.
◆ borders()
|
virtual |
This function should return the distance from each window side to the inner window.
The sizes may depend on the state of the decorated window, such as whether it's shaded. Decorations often turn off their bottom border when the window is shaded, and turn off their left/right/bottom borders when the window is maximized and moving and resizing of maximized windows is disabled. This function mustn't do any repaints or resizes. Also, if the sizes returned by this function don't match the real values, this may result in drawing errors or other problems.
- See also
- KDecorationOptions::moveResizeMaximizedWindows()
Implements KDecoration.
Definition at line 150 of file kcommondecoration.cpp.
◆ buttonsLeftWidth()
int KCommonDecoration::buttonsLeftWidth | ( | ) | const |
Definition at line 291 of file kcommondecoration.cpp.
◆ buttonsRightWidth()
int KCommonDecoration::buttonsRightWidth | ( | ) | const |
Definition at line 296 of file kcommondecoration.cpp.
◆ captionChange()
|
virtual |
This function is called whenever the caption changes.
Use caption() to get it.
Implements KDecoration.
Definition at line 587 of file kcommondecoration.cpp.
◆ cornerShape()
|
virtual |
- Returns
- the mask for the specific window corner.
Definition at line 139 of file kcommondecoration.cpp.
◆ createButton()
|
pure virtual |
Create a new title bar button.
KCommonDecoration takes care of memory management.
- Returns
- a pointer to the button, or 0 if the button should not be created.
◆ decorationBehaviour()
|
virtual |
This controls whether some specific behaviour should be enabled or not.
- See also
- DecorationBehaviour
Definition at line 63 of file kcommondecoration.cpp.
◆ defaultButtonsLeft()
|
pure virtual |
The default title button order on the left.
- See also
- KDecoration::titleButtonsLeft()
- KDecoration::titleButtonsRight()
◆ defaultButtonsRight()
|
pure virtual |
The default title button order on the left.
- See also
- KDecoration::titleButtonsLeft()
- KDecoration::titleButtonsRight()
◆ desktopChange()
|
virtual |
This function is called whenever the desktop for the window changes.
Use desktop() or isOnAllDesktops() to find out the current desktop on which the window is.
Implements KDecoration.
Definition at line 549 of file kcommondecoration.cpp.
◆ eventFilter()
|
virtual |
Definition at line 845 of file kcommondecoration.cpp.
◆ iconChange()
|
virtual |
This function is called whenever the window icon changes.
Use icon() to get it.
Implements KDecoration.
Definition at line 572 of file kcommondecoration.cpp.
◆ init()
|
virtual |
Handles widget and layout creation, call the base implementation when subclassing this member.
Implements KDecoration.
Definition at line 114 of file kcommondecoration.cpp.
◆ isToolWindow()
bool KCommonDecoration::isToolWindow | ( | ) | const |
Convenience method.
- Returns
- true if the window type is NET::Toolbar, NET::Utility, or NET::Menu
Definition at line 875 of file kcommondecoration.cpp.
◆ keepAboveChange
|
slot |
Definition at line 592 of file kcommondecoration.cpp.
◆ keepBelowChange
|
slot |
Definition at line 609 of file kcommondecoration.cpp.
◆ layoutMetric()
|
virtual |
This controls the layout of the decoration in various ways.
It is possible to have a different layout for different window states.
- Parameters
-
lm The layout element. respectWindowState Whether window states should be taken into account or a "default" state should be assumed. button For LM_ButtonWidth and LM_ButtonHeight, the button.
Definition at line 79 of file kcommondecoration.cpp.
◆ maximizeChange()
|
virtual |
This function is called whenever the maximalization state of the window changes.
Use maximizeMode() to get the current state.
Implements KDecoration.
Definition at line 536 of file kcommondecoration.cpp.
◆ menuButtonPressed
|
slot |
Definition at line 649 of file kcommondecoration.cpp.
◆ menuButtonReleased
|
slot |
Definition at line 672 of file kcommondecoration.cpp.
◆ minimumSize()
|
virtual |
This function should return the minimum required size for the decoration.
Note that the returned size shouldn't be too large, because it will be used to keep the decorated window at least as large.
Implements KDecoration.
Definition at line 525 of file kcommondecoration.cpp.
◆ mouseDoubleClickEvent()
|
virtual |
Definition at line 711 of file kcommondecoration.cpp.
◆ mousePosition()
|
virtual |
This function should return mouse cursor position in the decoration.
Positions at the edge will result in window resizing with mouse button pressed, center position will result in moving.
Implements KDecoration.
Definition at line 730 of file kcommondecoration.cpp.
◆ paintEvent()
|
pure virtual |
Draw the window decoration.
◆ reset()
|
virtual |
Handles SettingButtons, call the base implementation when subclassing this member.
Reimplemented from KDecoration.
Definition at line 131 of file kcommondecoration.cpp.
◆ resetButtons()
void KCommonDecoration::resetButtons | ( | ) | const |
Manually call reset() on each button.
Definition at line 248 of file kcommondecoration.cpp.
◆ resize()
|
virtual |
This method is called by twin when the style should resize the decoration window.
The usual implementation is to resize the main widget of the decoration to the given size.
- Parameters
-
s Specifies the new size of the decoration window.
Implements KDecoration.
Definition at line 520 of file kcommondecoration.cpp.
◆ resizeEvent()
|
virtual |
Definition at line 678 of file kcommondecoration.cpp.
◆ shadeChange()
|
virtual |
This function is called whenever the window is shaded or unshaded.
Use isShade() to get the current state.
Implements KDecoration.
Definition at line 560 of file kcommondecoration.cpp.
◆ show()
|
virtual |
Definition at line 513 of file kcommondecoration.cpp.
◆ slotKeepAbove
|
slot |
Definition at line 639 of file kcommondecoration.cpp.
◆ slotKeepBelow
|
slot |
Definition at line 644 of file kcommondecoration.cpp.
◆ slotMaximize
|
slot |
Definition at line 626 of file kcommondecoration.cpp.
◆ slotShade
|
slot |
Definition at line 634 of file kcommondecoration.cpp.
◆ titleRect()
TQRect KCommonDecoration::titleRect | ( | ) | const |
◆ updateButtons()
void KCommonDecoration::updateButtons | ( | ) | const |
Makes sure all buttons are repainted.
Definition at line 242 of file kcommondecoration.cpp.
◆ updateCaption()
|
virtual |
This is used to update the painting of the title bar after the caption has been changed.
Reimplement for a more efficient implementation (default calls update() on the whole decoration).
Definition at line 144 of file kcommondecoration.cpp.
◆ updateLayout()
void KCommonDecoration::updateLayout | ( | ) | const |
TODO: remove?
Definition at line 162 of file kcommondecoration.cpp.
◆ updateWindowShape()
|
virtual |
This updates the window mask using the information provided by cornerShape().
Edges which are aligned to screen corners are not shaped for better usability (remember to paint these areas in paintEvent(), too). You normally don't want/need to reimplement updateWindowShape().
- See also
- cornerShape()
Definition at line 795 of file kcommondecoration.cpp.
◆ visibleName()
|
pure virtual |
The name of the decoration used in the decoration preview.
◆ wheelEvent()
|
virtual |
Definition at line 723 of file kcommondecoration.cpp.
The documentation for this class was generated from the following files: