#include <player.h>
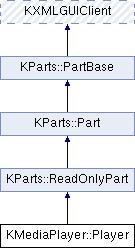
Public Types | |
enum | State { Empty , Stop , Pause , Play } |
Public Slots | |
virtual void | pause (void)=0 |
virtual void | play (void)=0 |
virtual void | stop (void)=0 |
virtual void | seek (unsigned long msec)=0 |
void | setLooping (bool) |
![]() | |
virtual bool | openURL (const KURL &url) |
Signals | |
void | loopingChanged (bool) |
void | stateChanged (int) |
![]() | |
void | started (TDEIO::Job *) |
void | completed () |
void | completed (bool pendingAction) |
void | canceled (const TQString &errMsg) |
![]() | |
void | setWindowCaption (const TQString &caption) |
void | setStatusBarText (const TQString &text) |
Public Member Functions | |
Player (TQObject *parent, const char *name) | |
Player (TQWidget *parentWidget, const char *widgetName, TQObject *parent, const char *name) | |
virtual View * | view (void)=0 |
virtual bool | isSeekable (void) const =0 |
virtual unsigned long | position (void) const =0 |
virtual bool | hasLength (void) const =0 |
virtual unsigned long | length (void) const =0 |
bool | isLooping (void) const |
int | state (void) const |
![]() | |
ReadOnlyPart (TQObject *parent=0, const char *name=0) | |
void | setProgressInfoEnabled (bool show) |
bool | isProgressInfoEnabled () const |
KURL | url () const |
virtual bool | closeURL () |
bool | openStream (const TQString &mimeType, const KURL &url) |
bool | writeStream (const TQByteArray &data) |
bool | closeStream () |
![]() | |
Part (TQObject *parent=0, const char *name=0) | |
virtual void | embed (TQWidget *parentWidget) |
virtual TQWidget * | widget () |
PartManager * | manager () const |
virtual Part * | hitTest (TQWidget *widget, const TQPoint &globalPos) |
virtual void | setSelectable (bool selectable) |
bool | isSelectable () const |
![]() | |
void | setPartObject (TQObject *object) |
![]() | |
KXMLGUIClient (KXMLGUIClient *parent) | |
TDEAction * | action (const char *name) const |
virtual TDEAction * | action (const TQDomElement &element) const |
virtual TDEActionCollection * | actionCollection () const |
virtual TDEInstance * | instance () const |
virtual TQDomDocument | domDocument () const |
virtual TQString | xmlFile () const |
void | setXMLGUIBuildDocument (const TQDomDocument &doc) |
TQDomDocument | xmlguiBuildDocument () const |
void | setFactory (KXMLGUIFactory *factory) |
KXMLGUIFactory * | factory () const |
KXMLGUIClient * | parentClient () const |
void | insertChildClient (KXMLGUIClient *child) |
void | removeChildClient (KXMLGUIClient *child) |
const TQPtrList< KXMLGUIClient > * | childClients () |
void | setClientBuilder (KXMLGUIBuilder *builder) |
KXMLGUIBuilder * | clientBuilder () const |
void | reloadXML () |
void | plugActionList (const TQString &name, const TQPtrList< TDEAction > &actionList) |
void | unplugActionList (const TQString &name) |
void | beginXMLPlug (TQWidget *) |
void | endXMLPlug () |
void | prepareXMLUnplug (TQWidget *) |
Protected Slots | |
void | setState (int) |
Additional Inherited Members | |
![]() | |
enum | PluginLoadingMode |
![]() | |
virtual bool | openFile ()=0 |
virtual void | guiActivateEvent (GUIActivateEvent *event) |
![]() | |
virtual void | setWidget (TQWidget *widget) |
virtual void | partActivateEvent (PartActivateEvent *event) |
virtual void | partSelectEvent (PartSelectEvent *event) |
TQWidget * | hostContainer (const TQString &containerName) |
![]() | |
virtual void | setInstance (TDEInstance *instance) |
virtual void | setInstance (TDEInstance *instance, bool loadPlugins) |
void | loadPlugins (TQObject *parent, KXMLGUIClient *parentGUIClient, TDEInstance *instance) |
void | setPluginLoadingMode (PluginLoadingMode loadingMode) |
![]() | |
virtual void | setXMLFile (const TQString &file, bool merge=false, bool setXMLDoc=true) |
virtual void | setXML (const TQString &document, bool merge=false) |
virtual void | setDOMDocument (const TQDomDocument &document, bool merge=false) |
virtual void | conserveMemory () |
virtual void | stateChanged (const TQString &newstate, ReverseStateChange reverse=StateNoReverse) |
![]() | |
KURL | m_url |
TQString | m_file |
bool | m_bTemp |
![]() | |
DoNotLoadPlugins | |
LoadPlugins | |
LoadPluginsIfEnabled | |
Detailed Description
Player is the center of the KMediaPlayer interface.
It provides all of the necessary media player operations, and optionally provides the GUI to control them.
There are two servicetypes for Player: KMediaPlayer/Player and KMediaPlayer/Engine. KMediaPlayer/Player provides a widget (accessable through view as well as XML GUI TDEActions. KMediaPlayer/Engine omits the user interface facets, for those who wish to provide their own interface.
Member Enumeration Documentation
◆ State
Constructor & Destructor Documentation
◆ Player() [1/2]
KMediaPlayer::Player::Player | ( | TQObject * | parent, |
const char * | name | ||
) |
This constructor is what to use when no GUI is required, as in the case of a KMediaPlayer/Engine.
Definition at line 39 of file player.cpp.
◆ Player() [2/2]
KMediaPlayer::Player::Player | ( | TQWidget * | parentWidget, |
const char * | widgetName, | ||
TQObject * | parent, | ||
const char * | name | ||
) |
This constructor is what to use when a GUI is required, as in the case of a KMediaPlayer/Player.
Definition at line 31 of file player.cpp.
Member Function Documentation
◆ hasLength()
|
pure virtual |
Returns whether the current track has a length.
Some streams are endless, and do not have one.
◆ isLooping()
bool KMediaPlayer::Player::isLooping | ( | void | ) | const |
Return the current looping state.
Definition at line 60 of file player.cpp.
◆ isSeekable()
|
pure virtual |
Returns whether the current track honors seek requests.
◆ length()
|
pure virtual |
Returns the length of the current track.
◆ loopingChanged
|
signal |
Emitted when the looping state is changed.
◆ pause
|
pure virtualslot |
Pause playback of the media track.
◆ play
|
pure virtualslot |
Begin playing the media track.
◆ position()
|
pure virtual |
Returns the current playback position in the track.
◆ seek
|
pure virtualslot |
Move the current playback position to the specified time in milliseconds, if the track is seekable.
Some streams may not be seeked.
◆ setLooping
|
slot |
Set whether the Player should continue playing at the beginning of the track when the end of the track is reached.
Definition at line 51 of file player.cpp.
◆ setState
|
protectedslot |
Implementers use this to control what users see as the current state.
Definition at line 65 of file player.cpp.
◆ state()
int KMediaPlayer::Player::state | ( | void | ) | const |
Return the current state of the player.
Definition at line 74 of file player.cpp.
◆ stateChanged
|
signal |
Emitted when the state changes.
◆ stop
|
pure virtualslot |
Stop playback of the media track and return to the beginning.
◆ view()
|
pure virtual |
The documentation for this class was generated from the following files: