#include <midiout.h>
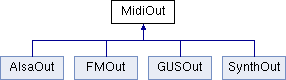
Public Member Functions | |
MidiOut (int d=0) | |
virtual | ~MidiOut () |
virtual void | openDev (int sqfd) |
virtual void | closeDev () |
virtual void | initDev () |
int | deviceType () const |
const char * | deviceName (void) const |
void | setMidiMapper (MidiMapper *map) |
virtual void | noteOn (uchar chn, uchar note, uchar vel) |
virtual void | noteOff (uchar chn, uchar note, uchar vel) |
virtual void | keyPressure (uchar chn, uchar note, uchar vel) |
virtual void | chnPatchChange (uchar chn, uchar patch) |
virtual void | chnPressure (uchar chn, uchar vel) |
virtual void | chnPitchBender (uchar chn, uchar lsb, uchar msb) |
virtual void | chnController (uchar chn, uchar ctl, uchar v) |
virtual void | sysex (uchar *data, ulong size) |
void | allNotesOff (void) |
virtual void | channelSilence (uchar chn) |
virtual void | channelMute (uchar chn, int b) |
virtual void | setVolumePercentage (int volper) |
int | ok (void) |
const char * | midiMapFilename () |
void | sync (int i=0) |
Protected Member Functions | |
void | seqbuf_dump (void) |
void | seqbuf_clean (void) |
Protected Attributes | |
int | seqfd |
int | device |
int | devicetype |
int | volumepercentage |
MidiMapper * | map |
uchar | chnpatch [16] |
int | chnbender [16] |
uchar | chnpressure [16] |
uchar | chncontroller [16][256] |
int | chnmute [16] |
int | _ok |
Detailed Description
External MIDI port output class .
This class is used to send midi events to external midi devices.
MidiOut is inherited by other MIDI devices classes (like SynthOut or FMOut) to support a common API.
In general, you don't want to use MidiOut directly, but within a DeviceManager object, which is the preferred way to generate music.
If you want to add support for other devices (I don't think there are any) you just have to create a class that inherits from MidiOut and create one object of your new class in DeviceManager::initManager().
Sends MIDI events to external MIDI devices
- Version
- 0.9.5 17/01/2000
Constructor & Destructor Documentation
◆ MidiOut()
MidiOut::MidiOut | ( | int | d = 0 | ) |
Constructor.
After constructing a MidiOut device, you must open it (using openDev() ). Additionally you may want to initialize it (with initDev() ),
Definition at line 46 of file midiout.cpp.
◆ ~MidiOut()
|
virtual |
Destructor.
It doesn't matter if you close the device ( closeDev() ) before you destruct the object because in other case, it will be closed here.
Definition at line 57 of file midiout.cpp.
Member Function Documentation
◆ allNotesOff()
void MidiOut::allNotesOff | ( | void | ) |
Send a All Notes Off event to every channel.
Definition at line 220 of file midiout.cpp.
◆ channelMute()
|
virtual |
Mute or "unmute" a given channel .
- Parameters
-
chn channel to work on b if true, the device will ignore subsequent notes played on the chn channel, and mute all notes being played on it. If b is false, the channel is back to work.
Reimplemented in AlsaOut.
Definition at line 240 of file midiout.cpp.
◆ channelSilence()
|
virtual |
Mutes all notes being played on a given channel.
- Parameters
-
chn the channel
Reimplemented in AlsaOut.
Definition at line 230 of file midiout.cpp.
◆ chnController()
|
virtual |
See DeviceManager::chnController()
Reimplemented in AlsaOut, FMOut, GUSOut, and SynthOut.
Definition at line 186 of file midiout.cpp.
◆ chnPatchChange()
|
virtual |
See DeviceManager::chnPatchChange()
Reimplemented in AlsaOut, FMOut, GUSOut, and SynthOut.
Definition at line 147 of file midiout.cpp.
◆ chnPitchBender()
|
virtual |
See DeviceManager::chnPitchBender()
Reimplemented in AlsaOut, FMOut, GUSOut, and SynthOut.
Definition at line 166 of file midiout.cpp.
◆ chnPressure()
|
virtual |
See DeviceManager::chnPressure()
Reimplemented in AlsaOut, FMOut, GUSOut, and SynthOut.
Definition at line 158 of file midiout.cpp.
◆ closeDev()
|
virtual |
◆ deviceName()
const char * MidiOut::deviceName | ( | void | ) | const |
Returns the name and type of this MIDI device.
- See also
- deviceType
Definition at line 280 of file midiout.cpp.
◆ deviceType()
|
inline |
- Returns
- the device type of the object. This is to identify the inherited class that a given object is polymorphed to. The returned value is one of these :
- KMID_EXTERNAL_MIDI if it's a MidiOut object
- KMID_SYNTH if it's a SynthOut object (as an AWE device)
- KMID_FM if it's a FMOut object
- KMID_GUS if it's a GUSOut object
which are defined in midispec.h
- See also
- deviceName
◆ initDev()
|
virtual |
◆ keyPressure()
|
virtual |
See DeviceManager::keyPressure()
Reimplemented in AlsaOut, FMOut, GUSOut, and SynthOut.
Definition at line 140 of file midiout.cpp.
◆ midiMapFilename()
const char * MidiOut::midiMapFilename | ( | void | ) |
Returns the path to the file where the current used MidiMapper object reads the configuration from, or an empty string if there's no MidiMapper.
Definition at line 275 of file midiout.cpp.
◆ noteOff()
|
virtual |
◆ noteOn()
|
virtual |
◆ ok()
|
inline |
◆ openDev()
|
virtual |
Opens the device.
This is generally called from DeviceManager , so you shouldn't call this yourself (except if you created the MidiOut object yourself.
- Parameters
-
sqfd a file descriptor of /dev/sequencer
Reimplemented in AlsaOut, FMOut, GUSOut, and SynthOut.
Definition at line 63 of file midiout.cpp.
◆ seqbuf_clean()
|
protected |
Definition at line 268 of file midiout.cpp.
◆ seqbuf_dump()
|
protected |
Definition at line 254 of file midiout.cpp.
◆ setMidiMapper()
void MidiOut::setMidiMapper | ( | MidiMapper * | map | ) |
Sets a MidiMapper object to be used to modify the midi events before sending them.
- Parameters
-
map the MidiMapper to use.
- See also
- MidiMapper
- midiMapFilename
Definition at line 107 of file midiout.cpp.
◆ setVolumePercentage()
|
inlinevirtual |
Change all channel volume events multiplying it by this percentage correction Instead of forcing a channel to a fixed volume, this method allows to music to fade out even when it was being played softly.
- Parameters
-
volper is an integer value, where 0 is quiet, 100 is used to send an unmodified value, 200 play music twice louder than it should, etc.
◆ sync()
void MidiOut::sync | ( | int | i = 0 | ) |
Sends the buffer to the device and returns when it's played, so you can synchronize XXX: sync should be virtual after next bic release.
Definition at line 294 of file midiout.cpp.
◆ sysex()
|
virtual |
Member Data Documentation
◆ _ok
◆ chnbender
◆ chncontroller
◆ chnmute
◆ chnpatch
◆ chnpressure
◆ device
◆ devicetype
◆ map
|
protected |
◆ seqfd
◆ volumepercentage
The documentation for this class was generated from the following files: