#include <kpassdlg.h>
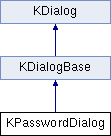
Public Types | |
enum | Types { Password , NewPassword } |
![]() | |
enum | ButtonCode { Help = 0x00000001 , Default = 0x00000002 , Ok = 0x00000004 , Apply = 0x00000008 , Try = 0x00000010 , Cancel = 0x00000020 , Close = 0x00000040 , User1 = 0x00000080 , User2 = 0x00000100 , User3 = 0x00000200 , No = 0x00000080 , Yes = 0x00000100 , Details = 0x00000400 , Filler = 0x40000000 , Stretch = 0x80000000 , NoDefault } |
enum | ActionButtonStyle { ActionStyle0 =0 , ActionStyle1 , ActionStyle2 , ActionStyle3 , ActionStyle4 , ActionStyleMAX } |
enum | DialogType { TreeList = KJanusWidget::TreeList , Tabbed = KJanusWidget::Tabbed , Plain = KJanusWidget::Plain , Swallow = KJanusWidget::Swallow , IconList = KJanusWidget::IconList } |
Public Member Functions | |
KPasswordDialog (Types type, bool enableKeep, int extraBttn, TQWidget *parent=0, const char *name=0) | |
KPasswordDialog (int type, TQString prompt, bool enableKeep=false, int extraBttn=0) TDE_DEPRECATED | |
KPasswordDialog (Types type, bool enableKeep, int extraBttn, const TQString &iconName, TQWidget *parent=0, const char *name=0) | |
virtual | ~KPasswordDialog () |
void | setPrompt (TQString prompt) |
TQString | prompt () const |
void | setKeepWarning (TQString warn) |
void | addLine (TQString key, TQString value) |
void | setAllowEmptyPasswords (bool allowed) |
bool | allowEmptyPasswords () const |
void | setMinimumPasswordLength (int minLength) |
int | minimumPasswordLength () const |
void | setMaximumPasswordLength (int maxLength) |
int | maximumPasswordLength () const |
void | setReasonablePasswordLength (int reasonableLength) |
int | reasonablePasswordLength () const |
void | setPasswordStrengthWarningLevel (int warningLevel) |
int | passwordStrengthWarningLevel () const |
TQString | password () const |
void | clearPassword () |
bool | keep () const |
![]() | |
KDialogBase (TQWidget *parent=0, const char *name=0, bool modal=true, const TQString &caption=TQString::null, int buttonMask=Ok|Apply|Cancel, ButtonCode defaultButton=Ok, bool separator=false, const KGuiItem &user1=KGuiItem(), const KGuiItem &user2=KGuiItem(), const KGuiItem &user3=KGuiItem()) | |
KDialogBase (int dialogFace, const TQString &caption, int buttonMask, ButtonCode defaultButton, TQWidget *parent=0, const char *name=0, bool modal=true, bool separator=false, const KGuiItem &user1=KGuiItem(), const KGuiItem &user2=KGuiItem(), const KGuiItem &user3=KGuiItem()) | |
KDialogBase (KDialogBase::DialogType dialogFace, WFlags f, TQWidget *parent=0, const char *name=0, bool modal=true, const TQString &caption=TQString::null, int buttonMask=Ok|Apply|Cancel, ButtonCode defaultButton=Ok, bool separator=false, const KGuiItem &user1=KGuiItem(), const KGuiItem &user2=KGuiItem(), const KGuiItem &user3=KGuiItem()) | |
KDialogBase (const TQString &caption, int buttonMask=Yes|No|Cancel, ButtonCode defaultButton=Yes, ButtonCode escapeButton=Cancel, TQWidget *parent=0, const char *name=0, bool modal=true, bool separator=false, const KGuiItem &yes=KStdGuiItem::yes(), const KGuiItem &no=KStdGuiItem::no(), const KGuiItem &cancel=KStdGuiItem::cancel()) | |
~KDialogBase () | |
void | setButtonBoxOrientation (int orientation) |
void | setEscapeButton (ButtonCode id) |
virtual void | adjustSize () |
virtual TQSize | sizeHint () const |
virtual TQSize | minimumSizeHint () const |
TQFrame * | plainPage () |
TQFrame * | addPage (const TQString &itemName, const TQString &header=TQString::null, const TQPixmap &pixmap=TQPixmap()) |
TQFrame * | addPage (const TQStringList &items, const TQString &header=TQString::null, const TQPixmap &pixmap=TQPixmap()) |
TQVBox * | addVBoxPage (const TQString &itemName, const TQString &header=TQString::null, const TQPixmap &pixmap=TQPixmap()) |
TQVBox * | addVBoxPage (const TQStringList &items, const TQString &header=TQString::null, const TQPixmap &pixmap=TQPixmap()) |
TQHBox * | addHBoxPage (const TQString &itemName, const TQString &header=TQString::null, const TQPixmap &pixmap=TQPixmap()) |
TQHBox * | addHBoxPage (const TQStringList &items, const TQString &header=TQString::null, const TQPixmap &pixmap=TQPixmap()) |
TQGrid * | addGridPage (int n, Orientation dir, const TQString &itemName, const TQString &header=TQString::null, const TQPixmap &pixmap=TQPixmap()) |
TQGrid * | addGridPage (int n, Orientation dir, const TQStringList &items, const TQString &header=TQString::null, const TQPixmap &pixmap=TQPixmap()) |
void | setFolderIcon (const TQStringList &path, const TQPixmap &pixmap) |
TQFrame * | makeMainWidget () |
TQVBox * | makeVBoxMainWidget () |
TQHBox * | makeHBoxMainWidget () |
TQGrid * | makeGridMainWidget (int n, Orientation dir) |
void | enableButtonSeparator (bool state) |
void | showButton (ButtonCode id, bool state) |
void | showButtonOK (bool state) |
void | showButtonApply (bool state) |
void | showButtonCancel (bool state) |
bool | showPage (int index) |
int | activePageIndex () const |
int | pageIndex (TQWidget *widget) const |
void | setMainWidget (TQWidget *widget) |
TQWidget * | mainWidget () |
void | disableResize () |
void | setInitialSize (const TQSize &s, bool noResize=false) |
void | incInitialSize (const TQSize &s, bool noResize=false) |
TQSize | configDialogSize (const TQString &groupName) const |
TQSize | configDialogSize (TDEConfig &config, const TQString &groupName) const |
void | saveDialogSize (const TQString &groupName, bool global=false) |
void | saveDialogSize (TDEConfig &config, const TQString &groupName, bool global=false) const |
void | setButtonOK (const KGuiItem &item=KStdGuiItem::ok()) |
void | setButtonOKText (const TQString &text=TQString::null, const TQString &tooltip=TQString::null, const TQString &quickhelp=TQString::null) TDE_DEPRECATED |
void | setButtonApply (const KGuiItem &item=KStdGuiItem::apply()) |
void | setButtonApplyText (const TQString &text=TQString::null, const TQString &tooltip=TQString::null, const TQString &quickhelp=TQString::null) TDE_DEPRECATED |
void | setButtonCancel (const KGuiItem &item=KStdGuiItem::cancel()) |
void | setButtonCancelText (const TQString &text=TQString::null, const TQString &tooltip=TQString::null, const TQString &quickhelp=TQString::null) TDE_DEPRECATED |
void | setButtonText (ButtonCode id, const TQString &text) |
void | setButtonTip (ButtonCode id, const TQString &text) |
void | setButtonWhatsThis (ButtonCode id, const TQString &text) |
void | setButtonGuiItem (ButtonCode id, const KGuiItem &item) |
void | setTreeListAutoResize (bool state) |
void | setShowIconsInTreeList (bool state) |
void | setRootIsDecorated (bool state) |
void | unfoldTreeList (bool persist=false) |
void | addWidgetBelowList (TQWidget *widget) |
void | addButtonBelowList (const TQString &text, TQObject *recv, const char *slot) |
void | addButtonBelowList (const KGuiItem &guiitem, TQObject *recv, const char *slot) |
void | setIconListAllVisible (bool state) |
void | showTile (bool state) |
void | getBorderWidths (int &ulx, int &uly, int &lrx, int &lry) const TDE_DEPRECATED |
TQRect | getContentsRect () const TDE_DEPRECATED |
TQSize | calculateSize (int w, int h) const |
TQString | helpLinkText () const |
TQPushButton * | actionButton (ButtonCode id) |
![]() | |
KDialog (TQWidget *parent=0, const char *name=0, bool modal=false, WFlags f=0) | |
Static Public Member Functions | |
static int | getPassword (TQString &password, TQString prompt, int *keep=0L) |
static int | getNewPassword (TQString &password, TQString prompt) |
static void | disableCoreDumps () |
![]() | |
static bool | haveBackgroundTile () |
static const TQPixmap * | backgroundTile () |
static const TQPixmap * | getBackgroundTile () TDE_DEPRECATED |
static void | setBackgroundTile (const TQPixmap *pix) |
![]() | |
static int | marginHint () |
static int | spacingHint () |
static void | resizeLayout (TQWidget *widget, int margin, int spacing) |
static void | resizeLayout (TQLayoutItem *lay, int margin, int spacing) |
static void | centerOnScreen (TQWidget *widget, int screen=-1) |
static bool | avoidArea (TQWidget *widget, const TQRect &area, int screen=-1) |
Protected Slots | |
void | slotOk () |
void | slotCancel () |
void | slotKeep (bool) |
void | slotLayout () |
![]() | |
virtual void | slotHelp () |
virtual void | slotDefault () |
virtual void | slotDetails () |
virtual void | slotUser3 () |
virtual void | slotUser2 () |
virtual void | slotUser1 () |
virtual void | slotOk () |
virtual void | slotApply () |
virtual void | slotTry () |
virtual void | slotYes () |
virtual void | slotNo () |
virtual void | slotCancel () |
virtual void | slotClose () |
virtual void | applyPressed () |
void | updateGeometry () |
void | slotDelayedDestruct () |
Protected Member Functions | |
virtual bool | checkPassword (const TQString &) |
virtual void | virtual_hook (int id, void *data) |
![]() | |
virtual void | keyPressEvent (TQKeyEvent *e) |
virtual void | hideEvent (TQHideEvent *) |
virtual void | closeEvent (TQCloseEvent *e) |
virtual void | virtual_hook (int id, void *data) |
virtual void | keyPressEvent (TQKeyEvent *) |
virtual void | virtual_hook (int id, void *data) |
Additional Inherited Members | |
![]() | |
void | enableButton (ButtonCode id, bool state) |
void | enableButtonOK (bool state) |
void | enableButtonApply (bool state) |
void | enableButtonCancel (bool state) |
void | enableLinkedHelp (bool state) |
void | delayedDestruct () |
void | setHelpLinkText (const TQString &text) |
void | setHelp (const TQString &anchor, const TQString &appname=TQString::null) |
void | helpClickedSlot (const TQString &) |
void | setDetails (bool showDetails) |
void | setDetailsWidget (TQWidget *detailsWidget) |
void | updateBackground () |
void | cancel () |
![]() | |
virtual void | polish () |
virtual void | setCaption (const TQString &caption) |
virtual void | setPlainCaption (const TQString &caption) |
![]() | |
void | helpClicked () |
void | defaultClicked () |
void | user3Clicked () |
void | user2Clicked () |
void | user1Clicked () |
void | applyClicked () |
void | tryClicked () |
void | okClicked () |
void | yesClicked () |
void | noClicked () |
void | cancelClicked () |
void | closeClicked () |
void | apply () |
void | backgroundChanged () |
void | hidden () |
void | finished () |
void | aboutToShowDetails () |
void | aboutToShowPage (TQWidget *page) |
![]() | |
void | layoutHintChanged () |
Detailed Description
A password input dialog.
This dialog asks the user to enter a password. The functions you're probably interested in are the static methods, getPassword() and getNewPassword().
Usage example
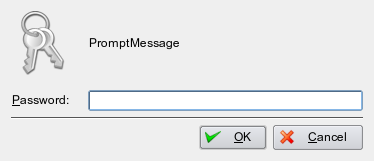
Security notes:
Keeping passwords in memory can be a potential security hole. You should handle this situation with care.
- You may want to use disableCoreDump() to disable core dumps. Core dumps are dangerous because they are an image of the process memory, and thus include any passwords that were in memory.
Definition at line 150 of file kpassdlg.h.
Member Enumeration Documentation
◆ Types
This enum distinguishes the two operation modes of this dialog:
Enumerator | |
---|---|
Password | The user is asked to enter a password. |
NewPassword | The user is asked to enter a password and to confirm it a second time. This is usually used when the user changes his password. |
Definition at line 159 of file kpassdlg.h.
Constructor & Destructor Documentation
◆ KPasswordDialog() [1/3]
KPasswordDialog::KPasswordDialog | ( | Types | type, |
bool | enableKeep, | ||
int | extraBttn, | ||
TQWidget * | parent = 0 , |
||
const char * | name = 0 |
||
) |
Constructs a password dialog.
- Parameters
-
type if NewPassword is given here, the dialog contains two input fields, so that the user must confirm his password and possible typos are detected immediately. enableKeep if true, a check box is shown in the dialog which allows the user to keep his password input for later. extraBttn allows to show additional buttons, KDialogBase. parent Passed to lower level constructor. name Passed to lower level constructor
- Since
- 3.0
Definition at line 179 of file kpassdlg.cpp.
◆ KPasswordDialog() [2/3]
KPasswordDialog::KPasswordDialog | ( | int | type, |
TQString | prompt, | ||
bool | enableKeep = false , |
||
int | extraBttn = 0 |
||
) |
- Deprecated:
- Variant of the previous constructor without the possibility to specify a parent. Will be removed in KDE 4.0
Definition at line 200 of file kpassdlg.cpp.
◆ KPasswordDialog() [3/3]
KPasswordDialog::KPasswordDialog | ( | Types | type, |
bool | enableKeep, | ||
int | extraBttn, | ||
const TQString & | iconName, | ||
TQWidget * | parent = 0 , |
||
const char * | name = 0 |
||
) |
Construct a password dialog.
Essentially the same as above but allows the icon in the password dialog to be set via iconName
.
- Parameters
-
type if NewPassword is given here, the dialog contains two input fields, so that the user must confirm his password and possible typos are detected immediately enableKeep if true, a check box is shown in the dialog which allows the user to keep his password input for later. extraBttn allows to show additional buttons. iconName the name of the icon to be shown in the dialog. If empty, a default icon is used parent Passed to lower level constructor. name Passed to lower level constructor
- Since
- 3.3
Definition at line 188 of file kpassdlg.cpp.
◆ ~KPasswordDialog()
|
virtual |
Destructs the password dialog.
Definition at line 342 of file kpassdlg.cpp.
Member Function Documentation
◆ addLine()
void KPasswordDialog::addLine | ( | TQString | key, |
TQString | value | ||
) |
Adds a line of information to the dialog.
Definition at line 376 of file kpassdlg.cpp.
◆ allowEmptyPasswords()
bool KPasswordDialog::allowEmptyPasswords | ( | ) | const |
◆ checkPassword()
|
inlineprotectedvirtual |
Virtual function that can be overridden to provide password checking in derived classes.
It should return true
if the password is valid, false
otherwise.
Definition at line 380 of file kpassdlg.h.
◆ clearPassword()
void KPasswordDialog::clearPassword | ( | ) |
Clears the password input field.
You might want to use this after the user failed to enter the correct password.
- Since
- 3.3
Definition at line 348 of file kpassdlg.cpp.
◆ disableCoreDumps()
|
static |
Static helper function that disables core dumps.
Definition at line 490 of file kpassdlg.cpp.
◆ getNewPassword()
|
static |
Pops up the dialog, asks the user for a password and returns it.
The user has to enter the password twice to make sure it was entered correctly.
- Parameters
-
password The password is returned in this reference parameter. prompt A prompt for the password. This can be a few lines of information. The text is word broken to fit nicely in the dialog.
- Returns
- Result code: Accepted or Rejected.
Definition at line 478 of file kpassdlg.cpp.
◆ getPassword()
|
static |
Pops up the dialog, asks the user for a password, and returns it.
- Parameters
-
password The password is returned in this reference parameter. prompt A prompt for the password. This can be a few lines of information. The text is word broken to fit nicely in the dialog. keep Enable/disable a checkbox controlling password keeping. If you pass a null pointer, or a pointer to the value 0, the checkbox is not shown. If you pass a pointer to a nonzero value, the checkbox is shown and the result is stored in *keep.
- Returns
- Result code: Accepted or Rejected.
Definition at line 461 of file kpassdlg.cpp.
◆ keep()
|
inline |
Returns true if the user wants to keep the password.
Definition at line 334 of file kpassdlg.h.
◆ maximumPasswordLength()
int KPasswordDialog::maximumPasswordLength | ( | ) | const |
◆ minimumPasswordLength()
int KPasswordDialog::minimumPasswordLength | ( | ) | const |
◆ password()
|
inline |
Returns the password entered.
Definition at line 322 of file kpassdlg.h.
◆ passwordStrengthWarningLevel()
int KPasswordDialog::passwordStrengthWarningLevel | ( | ) | const |
Password strength level below which a warning is given.
- Since
- 3.4
Definition at line 623 of file kpassdlg.cpp.
◆ prompt()
TQString KPasswordDialog::prompt | ( | ) | const |
Returns the password prompt.
Definition at line 368 of file kpassdlg.cpp.
◆ reasonablePasswordLength()
int KPasswordDialog::reasonablePasswordLength | ( | ) | const |
Password length that is expected to be reasonably safe.
- Since
- 3.4
Definition at line 612 of file kpassdlg.cpp.
◆ setAllowEmptyPasswords()
void KPasswordDialog::setAllowEmptyPasswords | ( | bool | allowed | ) |
◆ setKeepWarning()
void KPasswordDialog::setKeepWarning | ( | TQString | warn | ) |
Sets the text to be dynamically displayed when the keep checkbox is checked.
Definition at line 360 of file kpassdlg.cpp.
◆ setMaximumPasswordLength()
void KPasswordDialog::setMaximumPasswordLength | ( | int | maxLength | ) |
Maximum acceptable password length.
Limited to 199. Default: No limit, i.e. -1
- Parameters
-
maxLength The new maximum password length.
- Since
- 3.4
Definition at line 585 of file kpassdlg.cpp.
◆ setMinimumPasswordLength()
void KPasswordDialog::setMinimumPasswordLength | ( | int | minLength | ) |
Minimum acceptable password length.
Default: If empty passwords are forbidden, 1; Otherwise, 0.
- Parameters
-
minLength The new minimum password length
- Since
- 3.4
Definition at line 576 of file kpassdlg.cpp.
◆ setPasswordStrengthWarningLevel()
void KPasswordDialog::setPasswordStrengthWarningLevel | ( | int | warningLevel | ) |
Set the password strength level below which a warning is given Value is in the range 0 to 99.
Empty passwords score 0; non-empty passwords score up to 100, depending on their length and whether they contain numbers, mixed case letters and punctuation.
Default: 1 - warn if the password has no discernable strength whatsoever
- Parameters
-
warningLevel The level below which a warning should be given.
- Since
- 3.4
Definition at line 617 of file kpassdlg.cpp.
◆ setPrompt()
void KPasswordDialog::setPrompt | ( | TQString | prompt | ) |
Sets the password prompt.
Definition at line 354 of file kpassdlg.cpp.
◆ setReasonablePasswordLength()
void KPasswordDialog::setReasonablePasswordLength | ( | int | reasonableLength | ) |
Password length that is expected to be reasonably safe.
Default: 8 - the standard UNIX password length
- Parameters
-
reasonableLength The new reasonable password length.
- Since
- 3.4
Definition at line 603 of file kpassdlg.cpp.
◆ slotCancel
|
protectedslot |
Definition at line 434 of file kpassdlg.cpp.
◆ slotKeep
|
protectedslot |
Definition at line 440 of file kpassdlg.cpp.
◆ slotLayout
|
protectedslot |
Definition at line 455 of file kpassdlg.cpp.
◆ slotOk
|
protectedslot |
Definition at line 403 of file kpassdlg.cpp.
◆ virtual_hook()
|
protectedvirtual |
Reimplemented from KDialogBase.
Definition at line 497 of file kpassdlg.cpp.
The documentation for this class was generated from the following files: