#include <tdeactionclasses.h>
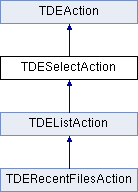
Public Slots | |
virtual void | setCurrentItem (int index) |
virtual void | setItems (const TQStringList &lst) |
virtual void | clear () |
virtual void | setEditable (bool) |
virtual void | setComboWidth (int width) |
![]() | |
virtual void | setText (const TQString &text) |
virtual bool | setShortcut (const TDEShortcut &) |
virtual void | setGroup (const TQString &) |
virtual void | setWhatsThis (const TQString &text) |
virtual void | setToolTip (const TQString &) |
virtual void | setIconSet (const TQIconSet &iconSet) |
virtual void | setIcon (const TQString &icon) |
virtual void | setEnabled (bool enable) |
void | setDisabled (bool disable) |
virtual void | setShortcutConfigurable (bool) |
virtual void | activate () |
Signals | |
void | activated (int index) |
void | activated (const TQString &text) |
![]() | |
void | activated () |
void | activated (TDEAction::ActivationReason reason, TQt::ButtonState state) |
void | enabled (bool) |
Public Member Functions | |
TDESelectAction (const TQString &text, const TDEShortcut &cut=TDEShortcut(), TQObject *parent=0, const char *name=0) | |
TDESelectAction (const TQString &text, const TDEShortcut &cut, const TQObject *receiver, const char *slot, TQObject *parent, const char *name=0) | |
TDESelectAction (const TQString &text, const TQIconSet &pix, const TDEShortcut &cut=TDEShortcut(), TQObject *parent=0, const char *name=0) | |
TDESelectAction (const TQString &text, const TQString &pix, const TDEShortcut &cut=TDEShortcut(), TQObject *parent=0, const char *name=0) | |
TDESelectAction (const TQString &text, const TQIconSet &pix, const TDEShortcut &cut, const TQObject *receiver, const char *slot, TQObject *parent, const char *name=0) | |
TDESelectAction (const TQString &text, const TQString &pix, const TDEShortcut &cut, const TQObject *receiver, const char *slot, TQObject *parent, const char *name=0) | |
TDESelectAction (TQObject *parent=0, const char *name=0) | |
virtual | ~TDESelectAction () |
virtual int | plug (TQWidget *widget, int index=-1) |
virtual bool | isEditable () const |
virtual TQStringList | items () const |
virtual void | changeItem (int index, const TQString &text) |
virtual TQString | currentText () const |
virtual int | currentItem () const |
virtual int | comboWidth () const |
void | setMaxComboViewCount (int n) |
TQPopupMenu * | popupMenu () const |
void | setRemoveAmpersandsInCombo (bool b) TDE_DEPRECATED |
bool | removeAmpersandsInCombo () const |
void | setMenuAccelsEnabled (bool b) |
bool | menuAccelsEnabled () const |
virtual bool | isShortcutConfigurable () const |
![]() | |
TDEAction (const TQString &text, const TDEShortcut &cut, const TQObject *receiver, const char *slot, TDEActionCollection *parent, const char *name) | |
TDEAction (const TQString &text, const TQIconSet &pix, const TDEShortcut &cut, const TQObject *receiver, const char *slot, TDEActionCollection *parent, const char *name) | |
TDEAction (const TQString &text, const TQString &pix, const TDEShortcut &cut, const TQObject *receiver, const char *slot, TDEActionCollection *parent, const char *name) | |
TDEAction (const KGuiItem &item, const TDEShortcut &cut, const TQObject *receiver, const char *slot, TDEActionCollection *parent, const char *name) | |
TDEAction (const TQString &text, const TDEShortcut &cut=TDEShortcut(), TQObject *parent=0, const char *name=0) | |
TDEAction (const TQString &text, const TDEShortcut &cut, const TQObject *receiver, const char *slot, TQObject *parent, const char *name=0) | |
TDEAction (const TQString &text, const TQIconSet &pix, const TDEShortcut &cut=TDEShortcut(), TQObject *parent=0, const char *name=0) | |
TDEAction (const TQString &text, const TQString &pix, const TDEShortcut &cut=TDEShortcut(), TQObject *parent=0, const char *name=0) | |
TDEAction (const TQString &text, const TQIconSet &pix, const TDEShortcut &cut, const TQObject *receiver, const char *slot, TQObject *parent, const char *name=0) | |
TDEAction (const TQString &text, const TQString &pix, const TDEShortcut &cut, const TQObject *receiver, const char *slot, TQObject *parent, const char *name=0) | |
TDEAction (TQObject *parent=0, const char *name=0) | |
virtual | ~TDEAction () |
virtual int | plug (TQWidget *widget, int index=-1) |
virtual void | plugAccel (TDEAccel *accel, bool configurable=true) TDE_DEPRECATED |
virtual void | unplug (TQWidget *w) |
virtual void | unplugAccel () TDE_DEPRECATED |
virtual bool | isPlugged () const |
bool | isPlugged (const TQWidget *container) const |
virtual bool | isPlugged (const TQWidget *container, int id) const |
virtual bool | isPlugged (const TQWidget *container, const TQWidget *_representative) const |
TQWidget * | container (int index) const |
int | itemId (int index) const |
TQWidget * | representative (int index) const |
int | containerCount () const |
uint | tdeaccelCount () const |
virtual bool | hasIcon () const |
bool | hasIconSet () const |
virtual TQString | plainText () const |
virtual TQString | text () const |
virtual const TDEShortcut & | shortcut () const |
virtual const TDEShortcut & | shortcutDefault () const |
TQString | shortcutText () const |
void | setShortcutText (const TQString &) |
virtual bool | isEnabled () const |
virtual bool | isShortcutConfigurable () const |
virtual TQString | group () const |
virtual TQString | whatsThis () const |
virtual TQString | toolTip () const |
virtual TQIconSet | iconSet (TDEIcon::Group group, int size=0) const |
TQIconSet | iconSet () const |
virtual TQString | icon () const |
TDEActionCollection * | parentCollection () const |
void | unplugAll () |
int | accel () const TDE_DEPRECATED |
TQString | statusText () const |
void | setAccel (int key) TDE_DEPRECATED |
void | setStatusText (const TQString &text) |
int | menuId (int i) |
Protected Slots | |
virtual void | slotActivated (int id) |
virtual void | slotActivated (const TQString &text) |
virtual void | slotActivated () |
![]() | |
virtual void | slotDestroyed () |
virtual void | slotKeycodeChanged () |
virtual void | slotActivated () |
void | slotPopupActivated () |
void | slotButtonClicked (int, TQt::ButtonState state) |
Protected Member Functions | |
virtual void | changeItem (int id, int index, const TQString &text) |
TQStringList | comboItems () const |
virtual void | updateCurrentItem (int id) |
virtual void | updateComboWidth (int id) |
virtual void | updateItems (int id) |
virtual void | updateClear (int id) |
virtual void | virtual_hook (int id, void *data) |
![]() | |
TDEToolBar * | toolBar (int index) const |
TQPopupMenu * | popupMenu (int index) const |
void | removeContainer (int index) |
int | findContainer (const TQWidget *widget) const |
int | findContainer (int id) const |
void | plugMainWindowAccel (TQWidget *w) |
void | addContainer (TQWidget *parent, int id) |
void | addContainer (TQWidget *parent, TQWidget *representative) |
virtual void | updateShortcut (int i) |
virtual void | updateShortcut (TQPopupMenu *menu, int id) |
virtual void | updateGroup (int id) |
virtual void | updateText (int i) |
virtual void | updateEnabled (int i) |
virtual void | updateIconSet (int i) |
virtual void | updateIcon (int i) |
virtual void | updateToolTip (int id) |
virtual void | updateWhatsThis (int i) |
TQString | whatsThisWithIcon () const |
const KGuiItem & | guiItem () const |
virtual void | virtual_hook (int id, void *data) |
Additional Inherited Members | |
![]() | |
enum | ActivationReason { UnknownActivation , EmulatedActivation , AccelActivation , PopupMenuActivation , ToolBarActivation } |
![]() | |
static int | getToolButtonID () |
![]() | |
TDEActionCollection * | m_parentCollection |
Detailed Description
Action for selecting one of several items.
Action for selecting one of several items.
This action shows up a submenu with a list of items. One of them can be checked. If the user clicks on an item this item will automatically be checked, the formerly checked item becomes unchecked. There can be only one item checked at a time.
Definition at line 331 of file tdeactionclasses.h.
Constructor & Destructor Documentation
◆ TDESelectAction() [1/7]
TDESelectAction::TDESelectAction | ( | const TQString & | text, |
const TDEShortcut & | cut = TDEShortcut() , |
||
TQObject * | parent = 0 , |
||
const char * | name = 0 |
||
) |
Constructs a select action with text and potential keyboard accelerator but nothing else.
Use this only if you really know what you are doing.
- Parameters
-
text The text that will be displayed. cut The corresponding keyboard accelerator (shortcut). parent This action's parent. name An internal name for this action.
Definition at line 376 of file tdeactionclasses.cpp.
◆ TDESelectAction() [2/7]
TDESelectAction::TDESelectAction | ( | const TQString & | text, |
const TDEShortcut & | cut, | ||
const TQObject * | receiver, | ||
const char * | slot, | ||
TQObject * | parent, | ||
const char * | name = 0 |
||
) |
- Parameters
-
text The text that will be displayed. cut The corresponding keyboard accelerator (shortcut). receiver The slot's parent. slot The slot to invoke to execute this action. parent This action's parent. name An internal name for this action.
Definition at line 383 of file tdeactionclasses.cpp.
◆ TDESelectAction() [3/7]
TDESelectAction::TDESelectAction | ( | const TQString & | text, |
const TQIconSet & | pix, | ||
const TDEShortcut & | cut = TDEShortcut() , |
||
TQObject * | parent = 0 , |
||
const char * | name = 0 |
||
) |
- Parameters
-
text The text that will be displayed. pix The icons that go with this action. cut The corresponding keyboard accelerator (shortcut). parent This action's parent. name An internal name for this action.
Definition at line 391 of file tdeactionclasses.cpp.
◆ TDESelectAction() [4/7]
TDESelectAction::TDESelectAction | ( | const TQString & | text, |
const TQString & | pix, | ||
const TDEShortcut & | cut = TDEShortcut() , |
||
TQObject * | parent = 0 , |
||
const char * | name = 0 |
||
) |
- Parameters
-
text The text that will be displayed. pix The dynamically loaded icon that goes with this action. cut The corresponding keyboard accelerator (shortcut). parent This action's parent. name An internal name for this action.
Definition at line 399 of file tdeactionclasses.cpp.
◆ TDESelectAction() [5/7]
TDESelectAction::TDESelectAction | ( | const TQString & | text, |
const TQIconSet & | pix, | ||
const TDEShortcut & | cut, | ||
const TQObject * | receiver, | ||
const char * | slot, | ||
TQObject * | parent, | ||
const char * | name = 0 |
||
) |
- Parameters
-
text The text that will be displayed. pix The icons that go with this action. cut The corresponding keyboard accelerator (shortcut). receiver The slot's parent. slot The slot to invoke to execute this action. parent This action's parent. name An internal name for this action.
Definition at line 407 of file tdeactionclasses.cpp.
◆ TDESelectAction() [6/7]
TDESelectAction::TDESelectAction | ( | const TQString & | text, |
const TQString & | pix, | ||
const TDEShortcut & | cut, | ||
const TQObject * | receiver, | ||
const char * | slot, | ||
TQObject * | parent, | ||
const char * | name = 0 |
||
) |
- Parameters
-
text The text that will be displayed. pix The dynamically loaded icon that goes with this action. cut The corresponding keyboard accelerator (shortcut). receiver The slot's parent. slot The slot to invoke to execute this action. parent This action's parent. name An internal name for this action.
Definition at line 417 of file tdeactionclasses.cpp.
◆ TDESelectAction() [7/7]
TDESelectAction::TDESelectAction | ( | TQObject * | parent = 0 , |
const char * | name = 0 |
||
) |
- Parameters
-
parent This action's parent. name An internal name for this action.
Definition at line 427 of file tdeactionclasses.cpp.
◆ ~TDESelectAction()
|
virtual |
Destructor.
Definition at line 433 of file tdeactionclasses.cpp.
Member Function Documentation
◆ activated [1/2]
|
signal |
This signal is emitted when an item is selected;.
- Parameters
-
text indicates the item selected.
◆ activated [2/2]
|
signal |
This signal is emitted when an item is selected;.
- Parameters
-
index indicated the item selected.
◆ changeItem() [1/2]
|
protectedvirtual |
Definition at line 534 of file tdeactionclasses.cpp.
◆ changeItem() [2/2]
|
virtual |
Changes the text of item.
- Parameters
-
index to text .
Definition at line 516 of file tdeactionclasses.cpp.
◆ clear
|
virtualslot |
Clears up all the items in this action.
Definition at line 744 of file tdeactionclasses.cpp.
◆ comboItems()
|
protected |
Depending on the menuAccelsEnabled property this method will return the actions items in a way for inclusion in a combobox with the ampersand character removed from all items or not.
- Since
- 3.1
Definition at line 725 of file tdeactionclasses.cpp.
◆ comboWidth()
|
virtual |
When this action is plugged into a toolbar, it creates a combobox.
This returns the maximum width set by setComboWidth
Definition at line 599 of file tdeactionclasses.cpp.
◆ currentItem()
|
virtual |
Returns the index of the current item.
- See also
- setCurrentItem
Reimplemented in TDEListAction.
Definition at line 579 of file tdeactionclasses.cpp.
◆ currentText()
|
virtual |
Returns the text of the currently selected item.
Reimplemented in TDEListAction.
Definition at line 571 of file tdeactionclasses.cpp.
◆ isEditable()
|
virtual |
When this action is plugged into a toolbar, it creates a combobox.
- Returns
- true if the combo editable.
Definition at line 812 of file tdeactionclasses.cpp.
◆ isShortcutConfigurable()
|
inlinevirtual |
Returns true if this action's shortcut is configurable.
Reimplemented from TDEAction.
Definition at line 500 of file tdeactionclasses.h.
◆ items()
|
virtual |
- Returns
- the items that can be selected with this action. Use setItems to set them.
Reimplemented in TDERecentFilesAction.
Definition at line 566 of file tdeactionclasses.cpp.
◆ menuAccelsEnabled()
bool TDESelectAction::menuAccelsEnabled | ( | ) | const |
- Since
- 3.1
Definition at line 832 of file tdeactionclasses.cpp.
◆ plug()
|
virtual |
"Plug" or insert this action into a given widget.
This will typically be a menu or a toolbar. From this point on, you will never need to directly manipulate the item in the menu or toolbar. You do all enabling/disabling/manipulation directly with your TDESelectAction object.
- Parameters
-
widget The GUI element to display this action. index The index of the item.
Reimplemented from TDEAction.
Reimplemented in TDERecentFilesAction.
Definition at line 639 of file tdeactionclasses.cpp.
◆ popupMenu()
TQPopupMenu * TDESelectAction::popupMenu | ( | ) | const |
Returns a pointer to the popup menu used by this action.
Definition at line 486 of file tdeactionclasses.cpp.
◆ removeAmpersandsInCombo()
bool TDESelectAction::removeAmpersandsInCombo | ( | ) | const |
- Since
- 3.1
Definition at line 822 of file tdeactionclasses.cpp.
◆ setComboWidth
|
virtualslot |
When this action is plugged into a toolbar, it creates a combobox.
This gives a maximum size to the combobox. The minimum size is automatically given by the contents (the items).
Definition at line 467 of file tdeactionclasses.cpp.
◆ setCurrentItem
|
virtualslot |
Sets the currently checked item.
- Parameters
-
index Index of the item (remember the first item is zero).
Definition at line 440 of file tdeactionclasses.cpp.
◆ setEditable
|
virtualslot |
When this action is plugged into a toolbar, it creates a combobox.
This makes the combo editable or read-only.
Definition at line 807 of file tdeactionclasses.cpp.
◆ setItems
|
virtualslot |
Sets the items to be displayed in this action You need to call this.
Definition at line 551 of file tdeactionclasses.cpp.
◆ setMaxComboViewCount()
void TDESelectAction::setMaxComboViewCount | ( | int | n | ) |
Sets the maximum items that are visible at once if the action is a combobox, that is the number of items in the combobox's viewport Only works before the action is plugged.
- Since
- 3.5
Definition at line 481 of file tdeactionclasses.cpp.
◆ setMenuAccelsEnabled()
void TDESelectAction::setMenuAccelsEnabled | ( | bool | b | ) |
Sets whether any occurrence of the ampersand character ( & ) in items should be interpreted as keyboard accelerator for items displayed in a menu or not.
- Since
- 3.1
Definition at line 827 of file tdeactionclasses.cpp.
◆ setRemoveAmpersandsInCombo()
void TDESelectAction::setRemoveAmpersandsInCombo | ( | bool | b | ) |
◆ slotActivated [1/3]
|
protectedvirtualslot |
Definition at line 799 of file tdeactionclasses.cpp.
◆ slotActivated [2/3]
|
protectedvirtualslot |
Definition at line 777 of file tdeactionclasses.cpp.
◆ slotActivated [3/3]
|
protectedvirtualslot |
Definition at line 766 of file tdeactionclasses.cpp.
◆ updateClear()
|
protectedvirtual |
Definition at line 754 of file tdeactionclasses.cpp.
◆ updateComboWidth()
|
protectedvirtual |
Definition at line 604 of file tdeactionclasses.cpp.
◆ updateCurrentItem()
|
protectedvirtual |
Definition at line 584 of file tdeactionclasses.cpp.
◆ updateItems()
|
protectedvirtual |
Definition at line 617 of file tdeactionclasses.cpp.
◆ virtual_hook()
|
protectedvirtual |
Reimplemented from TDEAction.
Definition at line 2383 of file tdeactionclasses.cpp.
The documentation for this class was generated from the following files: