#include <kreplacedialog.h>
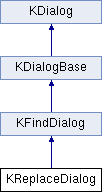
Public Types | |
enum | Options { PromptOnReplace = 256 , BackReference = 512 } |
![]() | |
enum | Options { WholeWordsOnly = 1 , FromCursor = 2 , SelectedText = 4 , CaseSensitive = 8 , FindBackwards = 16 , RegularExpression = 32 , FindIncremental = 64 , MinimumUserOption = 65536 } |
![]() | |
enum | ButtonCode |
enum | DialogType |
Public Member Functions | |
KReplaceDialog (TQWidget *parent=0, const char *name=0, long options=0, const TQStringList &findStrings=TQStringList(), const TQStringList &replaceStrings=TQStringList(), bool hasSelection=true) | |
virtual | ~KReplaceDialog () |
void | setReplacementHistory (const TQStringList &history) |
TQStringList | replacementHistory () const |
void | setOptions (long options) |
long | options () const |
TQString | replacement () const |
TQWidget * | replaceExtension () |
![]() | |
KFindDialog (TQWidget *parent=0, const char *name=0, long options=0, const TQStringList &findStrings=TQStringList(), bool hasSelection=false) | |
KFindDialog (bool modal, TQWidget *parent=0, const char *name=0, long options=0, const TQStringList &findStrings=TQStringList(), bool hasSelection=false) | |
virtual | ~KFindDialog () |
void | setFindHistory (const TQStringList &history) |
TQStringList | findHistory () const |
void | setHasSelection (bool hasSelection) |
void | setHasCursor (bool hasCursor) |
void | setSupportsBackwardsFind (bool supports) |
void | setSupportsCaseSensitiveFind (bool supports) |
void | setSupportsWholeWordsFind (bool supports) |
void | setSupportsRegularExpressionFind (bool supports) |
void | setOptions (long options) |
long | options () const |
TQString | pattern () const |
void | setPattern (const TQString &pattern) |
TQWidget * | findExtension () |
![]() | |
KDialogBase (TQWidget *parent=0, const char *name=0, bool modal=true, const TQString &caption=TQString::null, int buttonMask=Ok|Apply|Cancel, ButtonCode defaultButton=Ok, bool separator=false, const KGuiItem &user1=KGuiItem(), const KGuiItem &user2=KGuiItem(), const KGuiItem &user3=KGuiItem()) | |
KDialogBase (int dialogFace, const TQString &caption, int buttonMask, ButtonCode defaultButton, TQWidget *parent=0, const char *name=0, bool modal=true, bool separator=false, const KGuiItem &user1=KGuiItem(), const KGuiItem &user2=KGuiItem(), const KGuiItem &user3=KGuiItem()) | |
KDialogBase (KDialogBase::DialogType dialogFace, WFlags f, TQWidget *parent=0, const char *name=0, bool modal=true, const TQString &caption=TQString::null, int buttonMask=Ok|Apply|Cancel, ButtonCode defaultButton=Ok, bool separator=false, const KGuiItem &user1=KGuiItem(), const KGuiItem &user2=KGuiItem(), const KGuiItem &user3=KGuiItem()) | |
KDialogBase (const TQString &caption, int buttonMask=Yes|No|Cancel, ButtonCode defaultButton=Yes, ButtonCode escapeButton=Cancel, TQWidget *parent=0, const char *name=0, bool modal=true, bool separator=false, const KGuiItem &yes=KStdGuiItem::yes(), const KGuiItem &no=KStdGuiItem::no(), const KGuiItem &cancel=KStdGuiItem::cancel()) | |
void | setButtonBoxOrientation (int orientation) |
void | setEscapeButton (ButtonCode id) |
virtual void | adjustSize () |
TQFrame * | plainPage () |
TQFrame * | addPage (const TQString &itemName, const TQString &header=TQString::null, const TQPixmap &pixmap=TQPixmap()) |
TQFrame * | addPage (const TQStringList &items, const TQString &header=TQString::null, const TQPixmap &pixmap=TQPixmap()) |
TQVBox * | addVBoxPage (const TQString &itemName, const TQString &header=TQString::null, const TQPixmap &pixmap=TQPixmap()) |
TQVBox * | addVBoxPage (const TQStringList &items, const TQString &header=TQString::null, const TQPixmap &pixmap=TQPixmap()) |
TQHBox * | addHBoxPage (const TQString &itemName, const TQString &header=TQString::null, const TQPixmap &pixmap=TQPixmap()) |
TQHBox * | addHBoxPage (const TQStringList &items, const TQString &header=TQString::null, const TQPixmap &pixmap=TQPixmap()) |
TQGrid * | addGridPage (int n, Orientation dir, const TQString &itemName, const TQString &header=TQString::null, const TQPixmap &pixmap=TQPixmap()) |
TQGrid * | addGridPage (int n, Orientation dir, const TQStringList &items, const TQString &header=TQString::null, const TQPixmap &pixmap=TQPixmap()) |
void | setFolderIcon (const TQStringList &path, const TQPixmap &pixmap) |
TQFrame * | makeMainWidget () |
TQVBox * | makeVBoxMainWidget () |
TQHBox * | makeHBoxMainWidget () |
TQGrid * | makeGridMainWidget (int n, Orientation dir) |
void | enableButtonSeparator (bool state) |
void | showButton (ButtonCode id, bool state) |
void | showButtonOK (bool state) |
void | showButtonApply (bool state) |
void | showButtonCancel (bool state) |
bool | showPage (int index) |
int | activePageIndex () const |
int | pageIndex (TQWidget *widget) const |
void | setMainWidget (TQWidget *widget) |
TQWidget * | mainWidget () |
void | disableResize () |
void | setInitialSize (const TQSize &s, bool noResize=false) |
void | incInitialSize (const TQSize &s, bool noResize=false) |
TQSize | configDialogSize (const TQString &groupName) const |
TQSize | configDialogSize (TDEConfig &config, const TQString &groupName) const |
void | saveDialogSize (const TQString &groupName, bool global=false) |
void | saveDialogSize (TDEConfig &config, const TQString &groupName, bool global=false) const |
void | setButtonOK (const KGuiItem &item=KStdGuiItem::ok()) |
void | setButtonOKText (const TQString &text=TQString::null, const TQString &tooltip=TQString::null, const TQString &quickhelp=TQString::null) TDE_DEPRECATED |
void | setButtonApply (const KGuiItem &item=KStdGuiItem::apply()) |
void | setButtonApplyText (const TQString &text=TQString::null, const TQString &tooltip=TQString::null, const TQString &quickhelp=TQString::null) TDE_DEPRECATED |
void | setButtonCancel (const KGuiItem &item=KStdGuiItem::cancel()) |
void | setButtonCancelText (const TQString &text=TQString::null, const TQString &tooltip=TQString::null, const TQString &quickhelp=TQString::null) TDE_DEPRECATED |
void | setButtonText (ButtonCode id, const TQString &text) |
void | setButtonTip (ButtonCode id, const TQString &text) |
void | setButtonWhatsThis (ButtonCode id, const TQString &text) |
void | setButtonGuiItem (ButtonCode id, const KGuiItem &item) |
void | setTreeListAutoResize (bool state) |
void | setShowIconsInTreeList (bool state) |
void | setRootIsDecorated (bool state) |
void | unfoldTreeList (bool persist=false) |
void | addWidgetBelowList (TQWidget *widget) |
void | addButtonBelowList (const TQString &text, TQObject *recv, const char *slot) |
void | addButtonBelowList (const KGuiItem &guiitem, TQObject *recv, const char *slot) |
void | setIconListAllVisible (bool state) |
void | showTile (bool state) |
void | getBorderWidths (int &ulx, int &uly, int &lrx, int &lry) const TDE_DEPRECATED |
TQRect | getContentsRect () const TDE_DEPRECATED |
TQSize | calculateSize (int w, int h) const |
TQString | helpLinkText () const |
TQPushButton * | actionButton (ButtonCode id) |
![]() | |
KDialog (TQWidget *parent=0, const char *name=0, bool modal=false, WFlags f=0) | |
Protected Slots | |
void | slotOk () |
virtual void | showEvent (TQShowEvent *) |
![]() | |
void | slotOk () |
void | slotSelectedTextToggled (bool) |
void | showPatterns () |
void | showPlaceholders () |
void | textSearchChanged (const TQString &) |
void | slotRegexCheckBoxToggled (bool checked) |
void | slotPlaceholdersCheckBoxToggled (bool checked) |
![]() | |
virtual void | slotHelp () |
virtual void | slotDefault () |
virtual void | slotDetails () |
virtual void | slotUser3 () |
virtual void | slotUser2 () |
virtual void | slotUser1 () |
virtual void | slotOk () |
virtual void | slotApply () |
virtual void | slotTry () |
virtual void | slotYes () |
virtual void | slotNo () |
virtual void | slotCancel () |
virtual void | slotClose () |
virtual void | applyPressed () |
void | updateGeometry () |
void | slotDelayedDestruct () |
Additional Inherited Members | |
![]() | |
void | enableButton (ButtonCode id, bool state) |
void | enableButtonOK (bool state) |
void | enableButtonApply (bool state) |
void | enableButtonCancel (bool state) |
void | enableLinkedHelp (bool state) |
void | delayedDestruct () |
void | setHelpLinkText (const TQString &text) |
void | setHelp (const TQString &anchor, const TQString &appname=TQString::null) |
void | helpClickedSlot (const TQString &) |
void | setDetails (bool showDetails) |
void | setDetailsWidget (TQWidget *detailsWidget) |
void | updateBackground () |
void | cancel () |
![]() | |
virtual void | polish () |
virtual void | setCaption (const TQString &caption) |
virtual void | setPlainCaption (const TQString &caption) |
![]() | |
void | helpClicked () |
void | defaultClicked () |
void | user3Clicked () |
void | user2Clicked () |
void | user1Clicked () |
void | applyClicked () |
void | tryClicked () |
void | okClicked () |
void | yesClicked () |
void | noClicked () |
void | cancelClicked () |
void | closeClicked () |
void | apply () |
void | backgroundChanged () |
void | hidden () |
void | finished () |
void | aboutToShowDetails () |
void | aboutToShowPage (TQWidget *page) |
![]() | |
void | layoutHintChanged () |
![]() | |
static bool | haveBackgroundTile () |
static const TQPixmap * | backgroundTile () |
static const TQPixmap * | getBackgroundTile () TDE_DEPRECATED |
static void | setBackgroundTile (const TQPixmap *pix) |
![]() | |
static int | marginHint () |
static int | spacingHint () |
static void | resizeLayout (TQWidget *widget, int margin, int spacing) |
static void | resizeLayout (TQLayoutItem *lay, int margin, int spacing) |
static void | centerOnScreen (TQWidget *widget, int screen=-1) |
static bool | avoidArea (TQWidget *widget, const TQRect &area, int screen=-1) |
![]() | |
Help | |
Default | |
Ok | |
Apply | |
Try | |
Cancel | |
Close | |
User1 | |
User2 | |
User3 | |
No | |
Yes | |
Details | |
Filler | |
Stretch | |
NoDefault | |
![]() | |
virtual void | showEvent (TQShowEvent *) |
![]() | |
virtual void | keyPressEvent (TQKeyEvent *e) |
virtual void | hideEvent (TQHideEvent *) |
virtual void | closeEvent (TQCloseEvent *e) |
virtual void | keyPressEvent (TQKeyEvent *) |
Detailed Description
A generic "replace" dialog.
Detail:
This widget inherits from KFindDialog and implements the following additional functionalities: a replacement string object and an area for a user-defined widget to extend the dialog.
Example:
To use the basic replace dialog:
To use your own extensions:
Definition at line 59 of file kreplacedialog.h.
Member Enumeration Documentation
◆ Options
Options.
Enumerator | |
---|---|
PromptOnReplace | Should the user be prompted before the replace operation? |
Definition at line 69 of file kreplacedialog.h.
Constructor & Destructor Documentation
◆ KReplaceDialog()
KReplaceDialog::KReplaceDialog | ( | TQWidget * | parent = 0 , |
const char * | name = 0 , |
||
long | options = 0 , |
||
const TQStringList & | findStrings = TQStringList() , |
||
const TQStringList & | replaceStrings = TQStringList() , |
||
bool | hasSelection = true |
||
) |
Construct a replace dialog.read-only or rather select-only combo box with a parent object and a name.
- Parameters
-
parent The parent object of this widget name The name of this widget options A bitfield of the Options to be enabled. findStrings A TQStringList to insert in the combo box of text to find replaceStrings A TQStringList to insert in the combo box of text to replace with hasSelection Whether a selection exists
Definition at line 45 of file kreplacedialog.cpp.
◆ ~KReplaceDialog()
|
virtual |
Destructor.
Definition at line 54 of file kreplacedialog.cpp.
Member Function Documentation
◆ options()
long KReplaceDialog::options | ( | ) | const |
Returns the state of the options.
Disabled options may be returned in an indeterminate state.
- Returns
- The options.
- See also
- setOptions, Options, KFindDialog::Options
Definition at line 75 of file kreplacedialog.cpp.
◆ replaceExtension()
TQWidget * KReplaceDialog::replaceExtension | ( | ) |
Returns an empty widget which the user may fill with additional UI elements as required.
The widget occupies the width of the dialog, and is positioned immediately the regular expression support widgets for the replacement string.
- Returns
- An extensible TQWidget.
Definition at line 87 of file kreplacedialog.cpp.
◆ replacement()
TQString KReplaceDialog::replacement | ( | ) | const |
Returns the replacement string.
- Returns
- The replacement string.
Definition at line 98 of file kreplacedialog.cpp.
◆ replacementHistory()
TQStringList KReplaceDialog::replacementHistory | ( | ) | const |
Returns the list of history items.
- Returns
- The replacement history.
- See also
- setReplacementHistory
Definition at line 103 of file kreplacedialog.cpp.
◆ setOptions()
void KReplaceDialog::setOptions | ( | long | options | ) |
Set the options which are enabled.
- Parameters
-
options The setting of the Options.
- See also
- Options, KFindDialog::Options
Definition at line 112 of file kreplacedialog.cpp.
◆ setReplacementHistory()
void KReplaceDialog::setReplacementHistory | ( | const TQStringList & | history | ) |
Provide the list of strings
to be displayed as the history of replacement strings.
strings
might get truncated if it is too long.
- Parameters
-
history The replacement history.
- See also
- replacementHistory
Definition at line 119 of file kreplacedialog.cpp.
◆ showEvent
|
protectedvirtualslot |
Definition at line 59 of file kreplacedialog.cpp.
◆ slotOk
|
protectedslot |
Definition at line 127 of file kreplacedialog.cpp.
The documentation for this class was generated from the following files: