#include <KDGanttView.h>
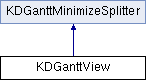
Public Types | |
enum | Scale { Minute , Hour , Day , Week , Month , Auto } |
enum | YearFormat { FourDigit , TwoDigit , TwoDigitApostrophe , NoDate } |
enum | HourFormat { Hour_24 , Hour_12 , Hour_24_FourDigit } |
enum | RepaintMode { No , Medium , Always } |
![]() | |
enum | ResizeMode { Stretch , KeepSize , FollowSizeHint } |
enum | Direction { Left , Right , Up , Down } |
Public Slots | |
void | editItem (KDGanttViewItem *) |
void | zoomToSelection (const TQDateTime &start, const TQDateTime &end) |
Public Member Functions | |
KDGanttView (TQWidget *parent=0, const char *name=0) | |
virtual void | show () |
virtual bool | close (bool alsoDelete) |
void | setRepaintMode (RepaintMode mode) |
void | setUpdateEnabled (bool enable) |
bool | getUpdateEnabled () const |
void | setGanttMaximumWidth (int w) |
int | ganttMaximumWidth () const |
void | setShowLegend (bool show) |
bool | showLegend () const |
void | setLegendIsDoctwindow (bool dock) |
bool | legendIsDoctwindow () const |
TQDockWindow * | legendDoctwindow () const |
void | setShowListView (bool show) |
bool | showListView () const |
void | setEditorEnabled (bool enable) |
bool | editorEnabled () const |
void | setListViewWidth (int) |
int | listViewWidth () |
void | setEditable (bool editable) |
bool | editable () const |
void | setCalendarMode (bool mode) |
bool | calendarMode () const |
void | setDisplaySubitemsAsGroup (bool show) |
bool | displaySubitemsAsGroup () const |
void | setDisplayEmptyTasksAsLine (bool show) |
bool | displayEmptyTasksAsLine () const |
void | setHorBackgroundLines (int count=2, TQBrush brush=TQBrush(TQColor(200, 200, 200), TQt::Dense6Pattern)) |
int | horBackgroundLines (TQBrush &brush) |
bool | saveProject (TQIODevice *) |
bool | loadProject (TQIODevice *) |
void | print (TQPrinter *printer=0, bool printListView=true, bool printTimeLine=true, bool printLegend=false) |
TQSize | drawContents (TQPainter *p=0, bool drawListView=true, bool drawTimeLine=true, bool drawLegend=false) |
void | setZoomFactor (double factor, bool absolute) |
double | zoomFactor () const |
void | zoomToFit () |
void | ensureVisible (KDGanttViewItem *) |
void | center (KDGanttViewItem *) |
void | centerTimeline (const TQDateTime ¢er) |
void | centerTimelineAfterShow (const TQDateTime ¢er) |
void | setTimelineToStart () |
void | setTimelineToEnd () |
void | addTicksLeft (int num=1) |
void | addTicksRight (int num=1) |
void | setShowTaskLinks (bool show) |
bool | showTaskLinks () const |
void | setFont (const TQFont &f) |
void | setShowHeaderPopupMenu (bool show=true, bool showZoom=true, bool showScale=true, bool showTime=true, bool showYear=true, bool showGrid=true, bool showPrint=false) |
bool | showHeaderPopupMenu () const |
void | setShowTimeTablePopupMenu (bool) |
bool | showTimeTablePopupMenu () const |
void | setShapes (KDGanttViewItem::Type type, KDGanttViewItem::Shape start, KDGanttViewItem::Shape middle, KDGanttViewItem::Shape end, bool overwriteExisting=true) |
bool | shapes (KDGanttViewItem::Type type, KDGanttViewItem::Shape &start, KDGanttViewItem::Shape &middle, KDGanttViewItem::Shape &end) const |
void | setColors (KDGanttViewItem::Type type, const TQColor &start, const TQColor &middle, const TQColor &end, bool overwriteExisting=true) |
bool | colors (KDGanttViewItem::Type type, TQColor &start, TQColor &middle, TQColor &end) const |
void | setDefaultColor (KDGanttViewItem::Type type, const TQColor &, bool overwriteExisting=true) |
TQColor | defaultColor (KDGanttViewItem::Type type) const |
void | setHighlightColors (KDGanttViewItem::Type type, const TQColor &start, const TQColor &middle, const TQColor &end, bool overwriteExisting=true) |
bool | highlightColors (KDGanttViewItem::Type type, TQColor &start, TQColor &middle, TQColor &end) const |
void | setDefaultHighlightColor (KDGanttViewItem::Type type, const TQColor &, bool overwriteExisting=true) |
TQColor | defaultHighlightColor (KDGanttViewItem::Type type) const |
void | setTextColor (const TQColor &color) |
TQColor | textColor () const |
void | setNoInformationBrush (const TQBrush &brush) |
TQBrush | noInformationBrush () const |
TQPtrList< KDGanttViewTaskLink > | taskLinks () const |
TQPtrList< KDGanttViewTaskLinkGroup > | taskLinkGroups () const |
void | addLegendItem (KDGanttViewItem::Shape shape, const TQColor &shapeColor, const TQString &text) |
void | clearLegend () |
void | setHorizonStart (const TQDateTime &start) |
TQDateTime | horizonStart () const |
void | setHorizonEnd (const TQDateTime &start) |
TQDateTime | horizonEnd () const |
void | setScale (Scale) |
Scale | scale () const |
void | setMaximumScale (Scale) |
Scale | maximumScale () const |
void | setMinimumScale (Scale) |
Scale | minimumScale () const |
void | setAutoScaleMinorTickCount (int count) |
int | autoScaleMinorTickCount () const |
void | setMajorScaleCount (int count) |
int | majorScaleCount () const |
void | setMinorScaleCount (int count) |
int | minorScaleCount () const |
void | setMinimumColumnWidth (int width) |
int | minimumColumnWidth () const |
void | setYearFormat (YearFormat format) |
YearFormat | yearFormat () const |
void | setHourFormat (HourFormat format) |
HourFormat | hourFormat () const |
void | setShowMajorTicks (bool) |
bool | showMajorTicks () const |
void | setShowMinorTicks (bool) |
bool | showMinorTicks () const |
void | setColumnBackgroundColor (const TQDateTime &column, const TQColor &color, Scale mini=KDGanttView::Minute, Scale maxi=KDGanttView::Month) |
void | addIntervalBackgroundColor (KDIntervalColorRectangle *newItem) |
void | clearBackgroundColor () |
TQColor | columnBackgroundColor (const TQDateTime &column) const |
void | setWeekendBackgroundColor (const TQColor &color) |
TQColor | weekendBackgroundColor () const |
void | setWeekdayBackgroundColor (const TQColor &color, int weekday) |
TQColor | weekdayBackgroundColor (int weekday) const |
void | setPaletteBackgroundColor (const TQColor &col) |
void | setGvBackgroundColor (const TQColor &) |
void | setLvBackgroundColor (const TQColor &) |
void | setTimeHeaderBackgroundColor (const TQColor &) |
void | setLegendHeaderBackgroundColor (const TQColor &) |
TQColor | gvBackgroundColor () const |
TQColor | lvBackgroundColor () const |
TQColor | timeHeaderBackgroundColor () const |
TQColor | legendHeaderBackgroundColor () const |
void | addUserdefinedLegendHeaderWidget (TQWidget *w) |
void | setWeekendDays (int start, int end) |
void | weekendDays (int &start, int &end) const |
void | setHeaderVisible (bool) |
bool | headerVisible () const |
void | setShowLegendButton (bool show) |
bool | showLegendButton () const |
virtual int | addColumn (const TQString &label, int width=-1) |
virtual int | addColumn (const TQIconSet &iconset, const TQString &label, int width=-1) |
virtual void | removeColumn (int index) |
KDGanttViewItem * | selectedItem () const |
void | setSelected (KDGanttViewItem *, bool) |
KDGanttViewItem * | firstChild () const |
KDGanttViewItem * | lastItem () const |
int | childCount () const |
void | clear () |
void | setDragEnabled (bool b) |
void | setDropEnabled (bool b) |
void | setDragDropEnabled (bool b) |
bool | dragEnabled () const |
bool | dropEnabled () const |
bool | isDragEnabled () const |
bool | isDropEnabled () const |
virtual bool | lvDropEvent (TQDropEvent *e, KDGanttViewItem *, KDGanttViewItem *) |
virtual void | lvStartDrag (KDGanttViewItem *) |
virtual bool | lvDragMoveEvent (TQDragMoveEvent *e, KDGanttViewItem *, KDGanttViewItem *) |
virtual void | lvDragEnterEvent (TQDragEnterEvent *e) |
virtual TQSize | sizeHint () const |
KDGanttViewItem * | getItemByName (const TQString &name) const |
TQDateTime | getDateTimeForCoordX (int coordX, bool global=true) const |
KDGanttViewItem * | getItemByListViewPos (const TQPoint &pos) const |
KDGanttViewItem * | getItemByGanttViewPos (const TQPoint &pos) const |
KDGanttViewItem * | getItemAt (const TQPoint &pos, bool global=true) const |
void | setLvVScrollBarMode (TQScrollView::ScrollBarMode) |
void | setGvVScrollBarMode (TQScrollView::ScrollBarMode) |
void | setLinkItemsEnabled (bool on) |
bool | isLinkItemsEnabled () const |
KDTimeTableWidget * | timeTableWidget () |
KDTimeHeaderWidget * | timeHeaderWidget () |
void | setFixedHorizon (bool f) |
![]() | |
KDGanttMinimizeSplitter (TQWidget *parent=0, const char *name=0) | |
KDGanttMinimizeSplitter (TQt::Orientation, TQWidget *parent=0, const char *name=0) | |
~KDGanttMinimizeSplitter () | |
virtual void | setOrientation (TQt::Orientation) |
TQt::Orientation | orientation () const |
void | setMinimizeDirection (Direction) |
Direction | minimizeDirection () const |
virtual void | setResizeMode (TQWidget *w, ResizeMode) |
virtual void | setOpaqueResize (bool=TRUE) |
bool | opaqueResize () const |
void | moveToFirst (TQWidget *) |
void | moveToLast (TQWidget *) |
void | refresh () |
virtual TQSize | sizeHint () const |
virtual TQSize | minimumSizeHint () const |
TQValueList< int > | sizes () const |
void | setSizes (TQValueList< int >) |
void | expandPos (int id, int *min, int *max) |
Static Public Member Functions | |
static TQPixmap | getPixmap (KDGanttViewItem::Shape shape, const TQColor &shapeColor, const TQColor &backgroundColor, int itemSize) |
Protected Member Functions | |
virtual TQDragObject * | dragObject () |
virtual void | startDrag () |
![]() | |
void | childEvent (TQChildEvent *) |
bool | event (TQEvent *) |
void | resizeEvent (TQResizeEvent *) |
int | idAfter (TQWidget *) const |
void | moveSplitter (TQCOORD pos, int id) |
virtual void | drawSplitter (TQPainter *, TQCOORD x, TQCOORD y, TQCOORD w, TQCOORD h) |
void | styleChange (TQStyle &) |
int | adjustPos (int, int) |
virtual void | setRubberband (int) |
void | getRange (int id, int *, int *) |
Friends | |
class | KDGanttCanvasView |
class | KDGanttViewEventItem |
class | KDGanttViewItem |
class | KDGanttViewTaskItem |
class | KDGanttViewSummaryItem |
class | KDGanttViewTaskLink |
class | KDGanttViewCalendarItem |
class | KDTimeTableWidget |
class | KDTimeHeaderWidget |
class | KDListView |
class | KDGanttViewTaskLinkGroup |
class | KDLegendWidget |
Detailed Description
This class represents a Gantt view with the Gantt chart, the header, an optional listview and an optional legend.
In order to set up a Gantt view, create an object of this class, and populate it with a number of KDGanttViewItem objects.
If you experience problems with the repainting of the content of the Gantt View after scrolling, call setRepaintMode().
Definition at line 70 of file KDGanttView.h.
Member Enumeration Documentation
◆ HourFormat
This enum is used to specify the hour format used in the header.
Definition at line 119 of file KDGanttView.h.
◆ RepaintMode
Please see setRepaintMode() for a description of the values of this enumeration.
Definition at line 120 of file KDGanttView.h.
◆ Scale
enum KDGanttView::Scale |
This enum is used to specify the units of the scales in the header.
Definition at line 117 of file KDGanttView.h.
◆ YearFormat
This enum is used to specify the year format used in the header.
Definition at line 118 of file KDGanttView.h.
Constructor & Destructor Documentation
◆ KDGanttView()
KDGanttView::KDGanttView | ( | TQWidget * | parent = 0 , |
const char * | name = 0 |
||
) |
Constructs an empty KDGanttView.
- Parameters
-
parent the widget parent name the internal debugging name
Definition at line 83 of file KDGanttView.cpp.
◆ ~KDGanttView()
KDGanttView::~KDGanttView | ( | ) |
Definition at line 200 of file KDGanttView.cpp.
Member Function Documentation
◆ addColumn() [1/2]
|
virtual |
Calls to this method are passed through to the underlying TQListView.
Definition at line 3892 of file KDGanttView.cpp.
◆ addColumn() [2/2]
|
virtual |
Calls to this method are passed through to the underlying TQListView.
Definition at line 3882 of file KDGanttView.cpp.
◆ addIntervalBackgroundColor()
void KDGanttView::addIntervalBackgroundColor | ( | KDIntervalColorRectangle * | newItem | ) |
Adds a filled rectangle for a time interval given by start and end, across all tasks. start may be later than end.
- See also
- KDIntervalColorRectangle
Definition at line 1978 of file KDGanttView.cpp.
◆ addLegendItem()
void KDGanttView::addLegendItem | ( | KDGanttViewItem::Shape | shape, |
const TQColor & | shapeColor, | ||
const TQString & | text | ||
) |
Adds an item to the legend.
- Parameters
-
shape the shape to display shapeColor the color in which to display the shape text the text to display
- See also
- clearLegend()
Definition at line 1570 of file KDGanttView.cpp.
◆ addTicksLeft()
void KDGanttView::addTicksLeft | ( | int | num = 1 | ) |
Add num minor ticks of the current scale of the timeline to the start of the timeline. The timeline is not set automatically at the start. Call setTimelineToStart() to ensure that the timeline is at the start after calling this method.
- Parameters
-
num the number of minor ticks which should be added
Definition at line 1143 of file KDGanttView.cpp.
◆ addTicksRight()
void KDGanttView::addTicksRight | ( | int | num = 1 | ) |
Add num minor ticks of the current scale of the timeline to the end of the timeline. The timeline is not set automatically at the end. Call setTimelineToEnd() to ensure that the timeline is at the end after calling this method.
- Parameters
-
num the number of minor ticks which should be added
Definition at line 1158 of file KDGanttView.cpp.
◆ addUserdefinedLegendHeaderWidget()
void KDGanttView::addUserdefinedLegendHeaderWidget | ( | TQWidget * | w | ) |
Adds a widget to the spacer widget above the list view part and below the ShowLegendButton. To assign all the space above the Listview to the spacer widget, hide the ShowLegendButton by calling setShowLegendButton( false ). The spacer widget is a TQHBox. You may add as many widgets as you want. They are ordered horizontally from left to right. To remove a widget from the spacer widget, call widget->reparent(newParent,...) or delete the widget. Since the spacer is a TQHBox, the layout of the added widgets is managed by this TQHBox.
- Parameters
-
w A pointer to the widget to be added.
- See also
- setShowLegendButton( )
Definition at line 4248 of file KDGanttView.cpp.
◆ autoScaleMinorTickCount()
int KDGanttView::autoScaleMinorTickCount | ( | ) | const |
Returns the absolut number of minor ticks, if scaling is set to Auto
- Returns
- the absolut number of minor ticks
Definition at line 1728 of file KDGanttView.cpp.
◆ calendarMode()
bool KDGanttView::calendarMode | ( | ) | const |
Returns true, if the Gantt view is in calendar mode. See setCalendarMode() for the meaning of calendar mode.
- Returns
- returns true, if the Gantt view is in calendermode
- See also
- setCalendarMode()
Definition at line 2371 of file KDGanttView.cpp.
◆ center()
void KDGanttView::center | ( | KDGanttViewItem * | item | ) |
Makes sure that the specified Gantt item is in the center of the visible Gantt chart (if possible).
Definition at line 1168 of file KDGanttView.cpp.
◆ centerTimeline()
void KDGanttView::centerTimeline | ( | const TQDateTime & | center | ) |
Makes sure that the specified TQDateTime is in the center of the visible Gantt chart (if possible). If you want to center the timeline when the KDGanttView is hidden, calling centerTimelineAfterShow() is the better alternative.
- See also
- center(), centerTimelineAfterShow()
Definition at line 1086 of file KDGanttView.cpp.
◆ centerTimelineAfterShow()
void KDGanttView::centerTimelineAfterShow | ( | const TQDateTime & | center | ) |
Makes sure that the specified TQDateTime is in the center of the visible Gantt chart (if possible). If the KDGanttView is currently hidden, this method resets the center once again after the next show() call. Use this method if you want to center the timeline when the KDGanttView is hidden. After calling KDGanttView::show(), there may be computations of the sizes of the widgets and subwidgets and of the automatically computed start datetime. This method ensures that the center of the timeline is to be properly reset after show().
- See also
- center(), centerTimeline()
Definition at line 1105 of file KDGanttView.cpp.
◆ childCount()
int KDGanttView::childCount | ( | ) | const |
Returns the number of items in the Gantt view.
- Returns
- the number of items in the Gantt view.
Definition at line 4079 of file KDGanttView.cpp.
◆ clear()
void KDGanttView::clear | ( | ) |
Removes all items from the Gantt view.
Definition at line 4088 of file KDGanttView.cpp.
◆ clearBackgroundColor()
void KDGanttView::clearBackgroundColor | ( | ) |
Removes all background color settings set with setColumnBackgroundColor() and setIntervalBackgroundColor(). Does not affect the settings of setWeekendBackgroundColor().
- See also
- setColumnBackgroundColor(), setWeekendBackgroundColor(), weekendBackgroundColor(), setIntervalBackgroundColor()
Definition at line 1991 of file KDGanttView.cpp.
◆ clearLegend()
void KDGanttView::clearLegend | ( | ) |
Removes all items from the legend.
- See also
- addLegendItem()
Definition at line 1553 of file KDGanttView.cpp.
◆ close()
|
virtual |
Closes the widget. The closing is rejected, if a repainting is currently being done.
- Parameters
-
alsoDelete if true, the widget is deleted
- Returns
- true, if the widget was closed
Definition at line 304 of file KDGanttView.cpp.
◆ colors()
bool KDGanttView::colors | ( | KDGanttViewItem::Type | type, |
TQColor & | start, | ||
TQColor & | middle, | ||
TQColor & | end | ||
) | const |
Queries the colors for a particular type of Gantt item.
- Parameters
-
type the type of Gantt items for which to query the colors start the start color is returned in this parameter middle the middle color is returned in this parameter end the end color is returned in this parameter
- Returns
- true if there was a general color set for the specified type. If the return value is false, the values of the three color parameters are undefined.
- See also
- setColors(), setDefaultColor(), defaultColor()
Definition at line 1413 of file KDGanttView.cpp.
◆ columnBackgroundColor()
TQColor KDGanttView::columnBackgroundColor | ( | const TQDateTime & | column | ) | const |
Returns the background color for the column closest to column.
- Parameters
-
column the column to query the background color for
- Returns
- the background color of the specified color
Definition at line 2005 of file KDGanttView.cpp.
◆ defaultColor()
TQColor KDGanttView::defaultColor | ( | KDGanttViewItem::Type | type | ) | const |
Returns the default color for a particular type of Gantt item that is used for the item if no specific start, middle, or end colors are set.
- Parameters
-
type the type of Gantt items for which to query the highlight colors
- Returns
- color the default color used
- See also
- setDefaultColor(), setColors(), colors()
Definition at line 2278 of file KDGanttView.cpp.
◆ defaultHighlightColor()
TQColor KDGanttView::defaultHighlightColor | ( | KDGanttViewItem::Type | type | ) | const |
Returns the default highlighting color for a particular type of Gantt item that is used for the item if no specific start, middle, or end colors are set.
- Parameters
-
type the type of Gantt items for which to query the highlight colors
- Returns
- color the default highlighting color used
Definition at line 2324 of file KDGanttView.cpp.
◆ displayEmptyTasksAsLine()
bool KDGanttView::displayEmptyTasksAsLine | ( | ) | const |
Returns, whether tasks where the start time and the end time are the same are displayed as a line over the full height of the Gantt view.
- Returns
- true, if empty tasks are displayed as line.
Definition at line 2431 of file KDGanttView.cpp.
◆ displaySubitemsAsGroup()
bool KDGanttView::displaySubitemsAsGroup | ( | ) | const |
Returns, whether new items are created with the displayHiddenSubitems property.
- Returns
- true, if hidden subitems should be displayed on newly created items.
- See also
- setDisplaySubitemsAsGroup(), KDGanttViewItem::setDisplaySubitemsAsGroup(), KDGanttViewItem::displaySubitemsAsGroup()
Definition at line 2406 of file KDGanttView.cpp.
◆ dragEnabled()
bool KDGanttView::dragEnabled | ( | ) | const |
- Deprecated:
- Use isDragEnabled() instead
Definition at line 4337 of file KDGanttView.cpp.
◆ dragObject()
|
protectedvirtual |
Implements a pass-through to the list view.
Definition at line 4110 of file KDGanttView.cpp.
◆ drawContents()
TQSize KDGanttView::drawContents | ( | TQPainter * | p = 0 , |
bool | drawListView = true , |
||
bool | drawTimeLine = true , |
||
bool | drawLegend = false |
||
) |
Paints a Gantt view on a TQPainter. You can specify, whether the list view, the time line, or the legend is painted. All combinations of these three widgets are allowed. Returns the size of the painted area. Paints the list view in the top-left corner, the time line to the right of the list view, and the legend below the list view. If called with p = 0, nothing is painted and only the size of the painted area is computed. This is useful for determining only the painted area and setting the scale of the painter, before calling this method with a painter. In order to get the output fitted to your paper and your printer, call first TQSize size = drawContents( 0, printListView, printTimeLine, printLegend ); then compute the scale dx = paper.width() / size.width(); dy = paper.height() / size.height(); then make float scale to fit the width or height of the paper if ( dx < dy ) scale = dx; else scale = dy; then set the scale p.scale( scale, scale ); and now call drawContents with painter p drawContents( &p, printListView, printTimeLine, printLegend );
For a detailed example, please see the commented source code in KDGanttView::print(...)
- Parameters
-
p a pointer to the painter to paint on. If p is 0, nothing is painted and only the size of the painted area is computed drawListView if true, the list view is painted drawTimeLine if true, the time line is painted drawLegend if true, the legend is painted
- Returns
- the size of the painted area
- See also
- print()
Definition at line 939 of file KDGanttView.cpp.
◆ dropEnabled()
bool KDGanttView::dropEnabled | ( | ) | const |
- Deprecated:
- Use isDropEnabled() instead
Definition at line 4346 of file KDGanttView.cpp.
◆ dropped
|
signal |
This signal is emitted whenever a Gantt item is dropped onto the Gantt view. droppedItem is 0, if this is a drag operation from another KDGanttView instance. If this drag is an internal drag (i.e. within the KDGanttView), this parameter points to the dropped item. itemBelowMouse is a pointer to the item below the dragged item (i.e., below the mouse). The dragged item may be inserted in the KDGanttView as a child of this item. If The value is 0, if there is no item below the dragged item, and the dragged item will be inserted as a root item.
In order to get user-defined behavior for drop events, reimplement KDGanttView::lvDropEvent()
◆ editable()
bool KDGanttView::editable | ( | ) | const |
Returns whether the Gantt chart is user-editable
- Returns
- true if the Gantt chart is user-editable
- See also
- setEditable()
Definition at line 762 of file KDGanttView.cpp.
◆ editItem
|
slot |
This slot is called when a new item has been added to the Gantt view. It will show the item attribute dialog in case the item is editable. item is a pointer to the item that has been created.
Definition at line 3746 of file KDGanttView.cpp.
◆ editorEnabled()
bool KDGanttView::editorEnabled | ( | ) | const |
Returns whether it is possible to edit the appearance of a Gantt item visually in a dialog by double-clicking the item.
- Returns
- true if visual editing is enabled, false otherwise
- See also
- setEditorEnabled()
Definition at line 735 of file KDGanttView.cpp.
◆ ensureVisible()
void KDGanttView::ensureVisible | ( | KDGanttViewItem * | item | ) |
Makes sure that the specified Gantt item is visible without scrolling.
- See also
- center(), centerTimelineAfterShow()
Definition at line 1072 of file KDGanttView.cpp.
◆ firstChild()
KDGanttViewItem * KDGanttView::firstChild | ( | ) | const |
Returns the first item in the Gantt view.
- Returns
- the first item in the Gantt view, 0 if there are no items
Definition at line 2336 of file KDGanttView.cpp.
◆ ganttMaximumWidth()
int KDGanttView::ganttMaximumWidth | ( | ) | const |
Returns the maximum width of the Gantt view part widget in pixels. The default maximum width is 32767 pixels.
- Returns
- the maximum width of the Gantt view part widget in pixels.
Definition at line 271 of file KDGanttView.cpp.
◆ getDateTimeForCoordX()
TQDateTime KDGanttView::getDateTimeForCoordX | ( | int | coordX, |
bool | global = true |
||
) | const |
Returns the corresponding date and time of the coordinate X in the Gantt view.
- Parameters
-
coordX the coordinate to search for global true if coordX is a global position, false otherwise
- Returns
- the date and time at coordinate X in the Gantt view.
Definition at line 421 of file KDGanttView.cpp.
◆ getItemAt()
KDGanttViewItem * KDGanttView::getItemAt | ( | const TQPoint & | pos, |
bool | global = true |
||
) | const |
Returns the pointer to the Gantt item at the position pos in the list view part of the Gantt view. The position pos is a global position if parameter global is true. If the vertical part (y coordinate) of pos (mapped to local coordinates) is less than 0 or larger than the height of the listview, 0 is returned. The horizontal part (x coordinate) of pos is ignored.
- Parameters
-
pos the position of the Gantt item global if true, pos is assumed to be global
- Returns
- the pointer to the item with position pos. O, if there is no item in the Gantt view at this position.
Definition at line 4013 of file KDGanttView.cpp.
◆ getItemByGanttViewPos()
KDGanttViewItem * KDGanttView::getItemByGanttViewPos | ( | const TQPoint & | pos | ) | const |
Returns the pointer to the Gantt item at the position pos in the Gantt view. The position pos is a global position. If no items are found, or the item is disabled, 0 is returned. If there is more than one item with the same position in the Gantt view, the first item found will be returned. This is not necessarily the first item in the listview.
- Parameters
-
pos the (global) position of the Gantt item
- Returns
- the pointer to the item with position pos. O, if there is no item in the Gantt view at this position.
Definition at line 3981 of file KDGanttView.cpp.
◆ getItemByListViewPos()
KDGanttViewItem * KDGanttView::getItemByListViewPos | ( | const TQPoint & | pos | ) | const |
Returns the pointer to the Gantt item at the position pos in the list view. The position pos is a global position. If no item is found, 0 is returned.
- Parameters
-
pos the (global) position of the Gantt item
- Returns
- the pointer to the item with position pos. O, if there is no item in the list view at this position.
Definition at line 3961 of file KDGanttView.cpp.
◆ getItemByName()
KDGanttViewItem * KDGanttView::getItemByName | ( | const TQString & | name | ) | const |
Returns the pointer to the Gantt item with the name name. If no item is found, the return value is 0. If there is more than one item with the same name in the Gantt view, the first item found will be returned. This may not necessarily be the first item in the listview.
- Parameters
-
name the name of the Gantt item
- Returns
- the pointer to the item with name name. O, if there is no item in the Gantt view with this name.
Definition at line 3938 of file KDGanttView.cpp.
◆ getPixmap()
|
static |
This method returns the pixmap used for a certain shape, in the selected color and size.
- Parameters
-
shape the shape to generate shapeColor the foreground color of the shape backgroundColor the background color of the shape itemSize the size of the shape
- Returns
- the generated shape pixmap
Definition at line 3769 of file KDGanttView.cpp.
◆ getUpdateEnabled()
bool KDGanttView::getUpdateEnabled | ( | ) | const |
Returns whether updating is enabled or not.
- Returns
- true, if updating is enabled
- See also
- setUpdateEnabled()
Definition at line 246 of file KDGanttView.cpp.
◆ gvBackgroundColor()
TQColor KDGanttView::gvBackgroundColor | ( | ) | const |
Returns the background color of the Gantt view.
- Returns
- the background color of the Gantt view
- See also
- setGvBackgroundColor()
Definition at line 4204 of file KDGanttView.cpp.
◆ gvContextMenuRequested
|
signal |
This signal is emitted when the user requests a context menu in the Gantt view. Notice that pos is the absolute mouse position.
◆ gvCurrentChanged
|
signal |
This signal is emitted whenever the user clicks on the Gantt view item parameter is 0, if no item was clicked
◆ gvItemDoubleClicked
|
signal |
This signal is emitted whenever the user double-clicks into the Gantt view.
◆ gvItemLeftClicked
|
signal |
This signal is emitted whenever the user clicks into the Gantt view with the left mouse button.
◆ gvItemMidClicked
|
signal |
This signal is emitted whenever the user clicks into the Gantt view with the middle mouse button.
◆ gvItemRightClicked
|
signal |
This signal is emitted whenever the user clicks into the Gantt view with the right mouse button.
◆ gvMouseButtonClicked
|
signal |
This signal is emitted when the user clicks into the Gantt view with any mouse button. Notice that pos is the absolute mouse position.
◆ headerVisible()
bool KDGanttView::headerVisible | ( | ) | const |
Returns whether the listview header is visible.
- Returns
- whether the header is visible
Definition at line 407 of file KDGanttView.cpp.
◆ highlightColors()
bool KDGanttView::highlightColors | ( | KDGanttViewItem::Type | type, |
TQColor & | start, | ||
TQColor & | middle, | ||
TQColor & | end | ||
) | const |
Queries the highlight colors for a particular type of Gantt item.
- Parameters
-
type the type of Gantt items for which to query the highlight colors start the start highlight color is returned in this parameter middle the middle highlight color is returned in this parameter end the end highlight color is returned in this parameter
- Returns
- true if there was a general highlight color set for the specified type. If the return value is false, the values of the three highlight color parameters are undefined.
Definition at line 1479 of file KDGanttView.cpp.
◆ horBackgroundLines()
int KDGanttView::horBackgroundLines | ( | TQBrush & | brush | ) |
Returns the definition of the horizontal background lines of the Gantt chart.
- Parameters
-
brush the brush of the lines
- Returns
- every nth line gets a background specified by brush if 0 is returned, no backgroud lines are drawn
Definition at line 2466 of file KDGanttView.cpp.
◆ horizonEnd()
TQDateTime KDGanttView::horizonEnd | ( | ) | const |
Returns the end of the horizon of the Gantt chart.
- Returns
- the end of the horizon of the Gantt chart
- See also
- setHorizonEnd()
Definition at line 1628 of file KDGanttView.cpp.
◆ horizonStart()
TQDateTime KDGanttView::horizonStart | ( | ) | const |
Returns the start of the horizon of the Gantt chart.
- Returns
- the start of the horizon of the Gantt chart
- See also
- setHorizonStart()
Definition at line 1602 of file KDGanttView.cpp.
◆ hourFormat()
KDGanttView::HourFormat KDGanttView::hourFormat | ( | ) | const |
Returns the format in which to display hours.
- Returns
- the hour format
- See also
- setHourFormat(), setYearFormat(), yearFormat()
Definition at line 1806 of file KDGanttView.cpp.
◆ isDragEnabled()
bool KDGanttView::isDragEnabled | ( | ) | const |
Returns whether dragging is enabled for this Gantt view.
- Returns
- true if dragging is enabled
- See also
- setDragEnabled(), setDragDropEnabled()
Definition at line 4316 of file KDGanttView.cpp.
◆ isDropEnabled()
bool KDGanttView::isDropEnabled | ( | ) | const |
Returns whether dropping is enabled for this Gantt view.
- Returns
- true if dropping is enabled
- See also
- setDropEnabled(), setDragDropEnabled()
Definition at line 4328 of file KDGanttView.cpp.
◆ isLinkItemsEnabled()
bool KDGanttView::isLinkItemsEnabled | ( | ) | const |
Returns if the linking functionallity is enabled or disabled.
Definition at line 4647 of file KDGanttView.cpp.
◆ itemConfigured
|
signal |
This signal is emitted when the user has configured an item visually.
◆ itemDoubleClicked
|
signal |
This signal is emitted when the user double-clicks an item.
◆ itemLeftClicked
|
signal |
This signal is emitted when the user clicks on an item with the left mouse button.
◆ itemMidClicked
|
signal |
This signal is emitted when the user clicks on an item with the middle mouse button.
◆ itemRightClicked
|
signal |
This signal is emitted when the user clicks on an item with the right mouse button.
◆ lastItem()
KDGanttViewItem * KDGanttView::lastItem | ( | ) | const |
Returns the last item in the Gantt view
- Returns
- the last item in the Gantt view, 0 if there are no items
Definition at line 2477 of file KDGanttView.cpp.
◆ legendDoctwindow()
TQDockWindow * KDGanttView::legendDoctwindow | ( | ) | const |
Returns the pointer to the legend dock window. DO NOT DELETE THIS POINTER! If the legend is not a dock window, 0 is returned To set the legend as a dock window, call KDGanttView::setLegendIsDoctwindow( true );
- Returns
- the pointer to the legend dock window 0 is returned, if the legend is no dock window DO NOT DELETE THIS POINTER!
Definition at line 647 of file KDGanttView.cpp.
◆ legendHeaderBackgroundColor()
TQColor KDGanttView::legendHeaderBackgroundColor | ( | ) | const |
Returns the background color of the legend header.
- Returns
- the background color of the legend header
- See also
- setLegendHeaderBackgroundColor()
Definition at line 4228 of file KDGanttView.cpp.
◆ legendIsDoctwindow()
bool KDGanttView::legendIsDoctwindow | ( | ) | const |
Returns whether the legend is shown as a dock window
- Returns
- true if the legend is shown as a dock window
Definition at line 629 of file KDGanttView.cpp.
◆ linkItems
|
signal |
This signal is emitted when the user wants to link two items in the Gantt view.
◆ listViewWidth()
int KDGanttView::listViewWidth | ( | ) |
Returns the width of the list view.
- Returns
- the width of the list view
- See also
- setListViewWidth()
Definition at line 4577 of file KDGanttView.cpp.
◆ loadProject()
bool KDGanttView::loadProject | ( | TQIODevice * | device | ) |
Loads a previously saved state of the Gantt view. All current settings and items are discarded before loading the data.
- Parameters
-
device a pointer to the IO device from which to load the Gantt view state.
- Returns
- true if the file could be read, false if an error occurred
- See also
- saveProject()
Definition at line 806 of file KDGanttView.cpp.
◆ lvBackgroundColor()
TQColor KDGanttView::lvBackgroundColor | ( | ) | const |
Returns the background color of the list view.
- Returns
- the background color of the list view
- See also
- setLvBackgroundColor()
Definition at line 4192 of file KDGanttView.cpp.
◆ lvContextMenuRequested
|
signal |
This signal is emitted when the user requests a context menu in the list view. Notice that pos is the absolute mouse position.
◆ lvCurrentChanged
|
signal |
This signal is emitted whenever the user clicks on the list view item parameter is 0, if no item was clicked
◆ lvDragEnterEvent()
|
virtual |
This virtual method specifies whether a drag enter event may be accepted or not. To accept a drag enter event, call e->accept( true ); To not accept a drag enter evente, call e->accept( false ); This method does nothing but accepting the drag enter event, in case decoding is possible. In order to define accepting drops for particular items yourself, subclass KDGanttView and reimplement this method.
- Parameters
-
e The TQDragMoveEvent Note: e->source() is a pointer to the KDGanttView, the drag started from. I.e., if e->source() == this, this drag is an internal drag.
- See also
- lvDropEvent(), lvStartDrag(), lvDragMoveEvent()
Definition at line 4442 of file KDGanttView.cpp.
◆ lvDragMoveEvent()
|
virtual |
This virtual method specifies whether a drop event may be accepted or not. To accept a drop event, call e->accept( true ); To not accept a drop event, call e->accept( false ); This method does nothing but allowing to execute the internal drag move event handling.
In order to specify user-defined drop acceptance for particular items, subclass KDGanttView and reimplement this method.
- Parameters
-
e The TQDragMoveEvent Note: e->source() is a pointer to the KDGanttView, the drag started from. I.e. if e->source() == this, this drag is an internal drag. draggedItem 0, if this is a drag operation from another KDGanttView instance. If this drag is an internal drag (i.e., within the KDGanttView), this parameter points to the dragged item. itemBelowMouse a pointer to the item below the dragged item (i.e., below the mouse). If you accept the drop, the dragged item will be inserted in the KDGanttView as a child of this item. The value is 0 if there is no item below the dragged item, and the dragged item will be inserted as a root item.
- Returns
- false, when the internal drag move event handling should be executed true, when the internal drag move event handling should not be executed; usually you should return true, if you have called e->accept( true ) before.
- See also
- lvDropEvent(), lvStartDrag()
Definition at line 4496 of file KDGanttView.cpp.
◆ lvDropEvent()
|
virtual |
This virtual method makes it possible to specify user-defined drop handling. The method is called directly before the internal drop handling is executed. Return false to execute internal drop handling. Return true to not execute internal drop handling. In order to specify user-defined drop handling, subclass KDGanttView and reimplement this method.
- Parameters
-
e The TQDropEvent Note: e->source() is a pointer to the KDGanttView from which the drag started. I.e., if e->source() == this, this drag is an internal drag. droppedItem 0, if this is a drag operation from another KDGanttView instance. If this drag is an internal drag (i.e., within the KDGanttView), this parameter points to the dropped item. itemBelowMouse a pointer to the item below the dragged item (i.e., below the mouse). If you accept, the dragged item may be inserted in the KDGanttView as a child of this item. The value is 0 if there is no item below the dragged item, and the dragged item will be inserted as a root item.
- Returns
- false, when the internal drop handling should be executed true, when the internal drop handling should not be executed
- See also
- lvDropEvent(), lvStartDrag()
Definition at line 4378 of file KDGanttView.cpp.
◆ lvItemDoubleClicked
|
signal |
This signal is emitted whenever the user double-clicks into the list view.
◆ lvItemLeftClicked
|
signal |
This signal is emitted whenever the user clicks into the list view with the left mouse button.
◆ lvItemMidClicked
|
signal |
This signal is emitted whenever the user clicks into the list view with the middle mouse button.
◆ lvItemRenamed
|
signal |
This signal is emitted whenever the user changes the name of an item in the list view using in-place editing. text contains the new text in the list view.
◆ lvItemRightClicked
|
signal |
This signal is emitted whenever the user clicks into the list view with the right mouse button.
◆ lvMouseButtonClicked
|
signal |
This signal is emitted when the user clicks into the list view with any mouse button . Notice that pos is the absolute mouse position.
◆ lvMouseButtonPressed
|
signal |
This signal is emitted when the user presses any mouse button in the list view. Notice that pos is the absolute mouse position.
◆ lvSelectionChanged
|
signal |
This signal is emitted whenever the user changes the selection in the list view.
◆ lvStartDrag()
|
virtual |
This virtual method creates a TQDragObject and starts a drag for a KDGanttViewItem. In order to prevent drags of particular items, subclass from KDGanttView and reimplement this method.
- Parameters
-
item the KDGanttViewItem, which should be dragged
- See also
- lvDropEvent(), lvDragMoveEvent()
Definition at line 4536 of file KDGanttView.cpp.
◆ majorScaleCount()
int KDGanttView::majorScaleCount | ( | ) | const |
Returns the number of ticks per unit in the major scale.
- Returns
- the number of ticks in the major scale
Definition at line 2208 of file KDGanttView.cpp.
◆ maximumScale()
KDGanttView::Scale KDGanttView::maximumScale | ( | ) | const |
Returns the maximum allowed time scale of the lower scale of the header.
- Returns
- the unit of the lower scale of the header.
- See also
- setScale()
Definition at line 1677 of file KDGanttView.cpp.
◆ minimumColumnWidth()
int KDGanttView::minimumColumnWidth | ( | ) | const |
Returns the minimum width a column needs to have.
- Returns
- the column minimum width
- See also
- setMinimumColumnWidth()
Definition at line 1754 of file KDGanttView.cpp.
◆ minimumScale()
KDGanttView::Scale KDGanttView::minimumScale | ( | ) | const |
Returns the minimum allowed time scale of the lower scale of the header.
- Returns
- the unit of the lower scale of the header.
- See also
- setScale()
Definition at line 1701 of file KDGanttView.cpp.
◆ minorScaleCount()
int KDGanttView::minorScaleCount | ( | ) | const |
Returns the number of ticks per unit in the minor scale.
- Returns
- the number of ticks in the minor scale
Definition at line 2232 of file KDGanttView.cpp.
◆ noInformationBrush()
TQBrush KDGanttView::noInformationBrush | ( | ) | const |
Returns the brush of the 'showNoInformation' lines
- Returns
- the brush of the 'showNoInformation' lines
- See also
- KDGanttViewItem::showNoInformation(), KDGanttViewItem::setShowNoInformation(), setNoInformationBrush()
Definition at line 1542 of file KDGanttView.cpp.
◆ print()
void KDGanttView::print | ( | TQPrinter * | printer = 0 , |
bool | printListView = true , |
||
bool | printTimeLine = true , |
||
bool | printLegend = false |
||
) |
Sends a Gantt view to a printer. The printer should already be set up for printing (by calling TQPrinter::setup()). If the printer is not set up, TQPrinter::setup() is called before printing
You can specify, whether the ListView, TimeLine, or Legend will be printed. All combinations of these three widgets are allowed.
- Parameters
-
printer a pointer to the printer to print on. If printer is 0, the method creates a temporary printer and discards it when it is done printing. printListView if true, the list view is printed printTimeLine if true, the time line is printed printLegend if true, the legend is printed
- See also
- drawContents()
Definition at line 847 of file KDGanttView.cpp.
◆ removeColumn()
|
virtual |
Calls to this method are passed through to the underlying TQListView.
Definition at line 3902 of file KDGanttView.cpp.
◆ rescaling
|
signal |
This signal is emitted if another scale is choosen than the specified one: i.e. if the horizon has a very wide range from start to end and as scale is choosen minute it may be that the size of the Gantt widget would become more than 32000 pixels. In this case the scale is automatically changed to Hour and rescaling( Hour ) is emitted. If the widget size would be still more than 32000 pixels, the scale is automatically changed to day and rescaling( Day ) is emitted. In the new scale, the minortickcount is increased such that the horizon will fit in the maximum size of 32000 pixels.
◆ saveProject()
bool KDGanttView::saveProject | ( | TQIODevice * | device | ) |
Saves the state of the Gantt view in an IO device in XML format. The saved data can be reloaded with loadProject().
- Parameters
-
device a pointer to the IO device in which to store the Gantt view state.
- Returns
- true if the data could be written, false if an error occurred
- See also
- loadProject()
Definition at line 779 of file KDGanttView.cpp.
◆ scale()
KDGanttView::Scale KDGanttView::scale | ( | ) | const |
Returns the unit of the lower scale of the header.
- Returns
- the unit of the lower scale of the header.
- See also
- setScale()
Definition at line 1653 of file KDGanttView.cpp.
◆ selectedItem()
KDGanttViewItem * KDGanttView::selectedItem | ( | ) | const |
Calls to this method are passed through to the underlying TQListView.
Definition at line 3911 of file KDGanttView.cpp.
◆ setAutoScaleMinorTickCount()
void KDGanttView::setAutoScaleMinorTickCount | ( | int | count | ) |
Sets the absolute number of minor ticks, if scaling is set to Auto. If the scale mode is set to Auto, then the actual scale and the minorScaleCount is automatically computed, such that there are count minor ticks
- Parameters
-
count the number of minor ticks
Definition at line 1716 of file KDGanttView.cpp.
◆ setCalendarMode()
void KDGanttView::setCalendarMode | ( | bool | mode | ) |
This method turns calendar mode on and off. In calendar mode, only those items can be opened which have subitems which have subitems. I.e., if an item contains multiple calendars, it can be opened, but not a calendar item itself. If you want to use this GanttView as a calendar view, you have to call setDisplaySubitemsAsGroup( true ); to use the root items as calendar items. To create new calendar entries for these root items, create a new KDGanttViewTaskItem with this root item as a parent. If you want an item with subitems to behave like a calendar (which is possibly empty at startup), please call setIsCalendar( true ); for this item.
- Parameters
-
mode if true, the calendar view mode is turned on if false, the calendar view mode is turned off
Definition at line 2358 of file KDGanttView.cpp.
◆ setColors()
void KDGanttView::setColors | ( | KDGanttViewItem::Type | type, |
const TQColor & | start, | ||
const TQColor & | middle, | ||
const TQColor & | end, | ||
bool | overwriteExisting = true |
||
) |
Sets the colors for a certain type of Gantt item. Not all items use all three colors (e.g., only summary items use the middle color).
This setting overrides any color settings made on individual items. These settings will be taken as initial values of any newly created item of this certain type. See also the description of the KDGanttViewItem class.
- Parameters
-
type the type of Gantt items for which to set the colors start the color to use for the beginning of the item middle the color to use for the middle of the item end the color to use for the end of the item overwriteExisting if true, overwrites existing color settings on invididual items
- See also
- colors(), setDefaultColors(), defaultColors()
Definition at line 1381 of file KDGanttView.cpp.
◆ setColumnBackgroundColor()
void KDGanttView::setColumnBackgroundColor | ( | const TQDateTime & | column, |
const TQColor & | color, | ||
Scale | mini = KDGanttView::Minute , |
||
Scale | maxi = KDGanttView::Month |
||
) |
Sets the background color for the column closest to column. It can be specified whether the color should be shown in all scales or only in specific scales. If you want to define the color only for the daily view, specify mini and maxi as Day. If there is no value specified for mini and maxi, the color for the column is shown on all scales. Note that it is possible that there are two values for a column in a scale. In this case, the shown color is unspecified.
- Parameters
-
column the column to set the background color for color the background color mini show the colour only in scales greater than this maxi show the colour only in scales lesser than this
Definition at line 1887 of file KDGanttView.cpp.
◆ setDefaultColor()
void KDGanttView::setDefaultColor | ( | KDGanttViewItem::Type | type, |
const TQColor & | color, | ||
bool | overwriteExisting = true |
||
) |
Sets the default color for a particular type of Gantt item that is used for the item if no specific start, middle, or end colors are set.
- Parameters
-
type the type of Gantt items for which to query the highlight colors color the default color to use overwriteExisting if true, existing settings for individual items are overwritten
- See also
- defaultColor(), setColors(), colors()
Definition at line 2251 of file KDGanttView.cpp.
◆ setDefaultHighlightColor()
void KDGanttView::setDefaultHighlightColor | ( | KDGanttViewItem::Type | type, |
const TQColor & | color, | ||
bool | overwriteExisting = true |
||
) |
Sets the default highlighting color for a particular type of Gantt item that is used for the item if no specific start, middle, or end colors are set.
- Parameters
-
type the type of Gantt items for which to query the highlight colors color the default highlighting color to use overwriteExisting if true, existing color settings in individual items are overwritten
Definition at line 2297 of file KDGanttView.cpp.
◆ setDisplayEmptyTasksAsLine()
void KDGanttView::setDisplayEmptyTasksAsLine | ( | bool | show | ) |
This method specifies whether tasks where the start time and the end time are the same are displayed as a line over the full height of the Gantt view.
- Parameters
-
show if true, tasks with starttime == endtime are displayed as a line
Definition at line 2419 of file KDGanttView.cpp.
◆ setDisplaySubitemsAsGroup()
void KDGanttView::setDisplaySubitemsAsGroup | ( | bool | show | ) |
This method specifies whether hidden subitems should be displayed. It iterates over all KDGanttViewItems in this Gantt view and sets their displaySubitemsAsGroup() property. All newly created items will have this setting by default.
- Parameters
-
show if true, the hidden subitems are displayed in all items of this Gantt view.
Definition at line 2387 of file KDGanttView.cpp.
◆ setDragDropEnabled()
void KDGanttView::setDragDropEnabled | ( | bool | b | ) |
Combines setDragEnabled() and setDropEnabled() in one convenient method.
- Parameters
-
b true if dragging and dropping are enabled, false if dragging and dropping are disabled
- See also
- setDragEnabled(), setDropEnabled()
Definition at line 4303 of file KDGanttView.cpp.
◆ setDragEnabled()
void KDGanttView::setDragEnabled | ( | bool | b | ) |
Specifies whether drag operations are allowed in the Gantt view. Recurses over all items contained in the Gantt view and enables or disabled them for dragging.
- Parameters
-
b true if dragging is enabled, false if dragging is disabled
Definition at line 4264 of file KDGanttView.cpp.
◆ setDropEnabled()
void KDGanttView::setDropEnabled | ( | bool | b | ) |
Specifies whether drop operations are allowed in the Gantt view. Recurses over all items contained in the Gantt view and enables or disabled them for dropping.
- Parameters
-
b true if dragging is enabled, false if dragging is disabled
Definition at line 4283 of file KDGanttView.cpp.
◆ setEditable()
void KDGanttView::setEditable | ( | bool | editable | ) |
Specifies whether the Gantt chart is user-editable.
- Parameters
-
editable pass true in order to get a user-editable Gantt chart, pass false in order to get a read-only chart
- See also
- editable()
Definition at line 749 of file KDGanttView.cpp.
◆ setEditorEnabled()
void KDGanttView::setEditorEnabled | ( | bool | enable | ) |
Specifies whether it should be possible to edit the appearance of a Gantt item visually in a dialog by double-clicking the item.
- Parameters
-
enable pass true in order to enable the visual editor and false in order to turn it off
- See also
- editorEnabled()
Definition at line 721 of file KDGanttView.cpp.
◆ setFixedHorizon()
|
inline |
Definition at line 351 of file KDGanttView.h.
◆ setFont()
void KDGanttView::setFont | ( | const TQFont & | font | ) |
Sets the font in the left list view widget and in the right time header widget. The settings of the fonts in the time table widget are not effected.
- Parameters
-
font the new font of the widget
Definition at line 1209 of file KDGanttView.cpp.
◆ setGanttMaximumWidth()
void KDGanttView::setGanttMaximumWidth | ( | int | w | ) |
Sets the maximum width of the Gantt view part widget in pixels. The largest allowed width is 32767.
- Parameters
-
w the maximum width
Definition at line 260 of file KDGanttView.cpp.
◆ setGvBackgroundColor()
void KDGanttView::setGvBackgroundColor | ( | const TQColor & | c | ) |
Sets the background color of the Gantt view.
- Parameters
-
c the background color of the Gantt view.
- See also
- gvBackgroundColor()
Definition at line 4141 of file KDGanttView.cpp.
◆ setGvVScrollBarMode()
void KDGanttView::setGvVScrollBarMode | ( | TQScrollView::ScrollBarMode | m | ) |
Sets the scrollbar mode of the time table. The default is always on. Possible values are always on and always off. It only makes sense to set this to always off if setLvVScrollBarMode() is set to always on or auto.
- Parameters
-
m The scrollbar mode.
- See also
- setLvVScrollBarMode( )
Definition at line 4607 of file KDGanttView.cpp.
◆ setHeaderVisible()
void KDGanttView::setHeaderVisible | ( | bool | visible | ) |
Specifies whether the listview header should be visible. By default, it is not visible.
- Parameters
-
visible true to make the header visible, false to make it invisible
Definition at line 391 of file KDGanttView.cpp.
◆ setHighlightColors()
void KDGanttView::setHighlightColors | ( | KDGanttViewItem::Type | type, |
const TQColor & | start, | ||
const TQColor & | middle, | ||
const TQColor & | end, | ||
bool | overwriteExisting = true |
||
) |
Sets the highlight colors for a certain type of Gantt item. Not all items use all three highlight colors (e.g., only summary items use the middle highlight color).
This setting overrides any highlight color settings made on individual items. These settings will be taken as initial values of any newly created item of this certain type. See also the description of the KDGanttViewItem class.
- Parameters
-
type the type of Gantt items for which to set the highlight colors start the highlight color to use for the beginning of the item middle the highlight color to use for the middle of the item end the highlight color to use for the end of the item overwriteExisting if true, overwrites existing color settings in the individual items
Definition at line 1443 of file KDGanttView.cpp.
◆ setHorBackgroundLines()
void KDGanttView::setHorBackgroundLines | ( | int | count = 2 , |
TQBrush | brush = TQBrush( TQColor ( 200,200,200 ), TQt::Dense6Pattern ) |
||
) |
Defines the horizontal background lines of the Gantt chart. Call setHorBackgroundLines() (equivalent to setHorBackgroundLines( 2, TQBrush( TQColor ( 240,240,240 )) ) ) to draw a light grey horizontal background line for every second Gantt item. Call setHorBackgroundLines(0) in order to not show horizontal background lines. You may specify the number of lines and the brush of the lines.
- Parameters
-
count for count >= 2, every count line gets a backgroud specified by brush for count < 2, no background lines are drawn brush the brush of the lines
Definition at line 2451 of file KDGanttView.cpp.
◆ setHorizonEnd()
void KDGanttView::setHorizonEnd | ( | const TQDateTime & | end | ) |
Sets the end of the horizon of the Gantt chart. If end is null, the horizon end is computed automatically.
- Parameters
-
end the end of the horizon
- See also
- setHorizonEnd()
Definition at line 1615 of file KDGanttView.cpp.
◆ setHorizonStart()
void KDGanttView::setHorizonStart | ( | const TQDateTime & | start | ) |
Sets the start of the horizon of the Gantt chart. If start is null, the horizon start is computed automatically.
- Parameters
-
start the start of the horizon
- See also
- horizonStart()
Definition at line 1590 of file KDGanttView.cpp.
◆ setHourFormat()
void KDGanttView::setHourFormat | ( | HourFormat | format | ) |
Specifies the format in which to display hours. If no hours are shown, this method has no effect.
- Parameters
-
format the hour format
- See also
- hourFormat(), setYearFormat(), yearFormat()
Definition at line 1793 of file KDGanttView.cpp.
◆ setLegendHeaderBackgroundColor()
void KDGanttView::setLegendHeaderBackgroundColor | ( | const TQColor & | c | ) |
Sets the background color of the legend header.
- Parameters
-
c the background color of the legend header
- See also
- legendHeaderBackgroundColor()
Definition at line 4167 of file KDGanttView.cpp.
◆ setLegendIsDoctwindow()
void KDGanttView::setLegendIsDoctwindow | ( | bool | show | ) |
Specifies whether the legend should be shown as a dock window or not.
- Parameters
-
show if true, show legend as a dock window
Definition at line 610 of file KDGanttView.cpp.
◆ setLinkItemsEnabled()
void KDGanttView::setLinkItemsEnabled | ( | bool | on | ) |
This enables/disables the linking ui of KDGanttViewItems in KDGanttView. A signal linkItems() is emitted when two items shall be linked and can be used to create the actual link.
Definition at line 4636 of file KDGanttView.cpp.
◆ setListViewWidth()
void KDGanttView::setListViewWidth | ( | int | w | ) |
Sets the width of the list view. Space will be taken from or given to the Gantt view.
- Parameters
-
w the width of the list view
- See also
- listViewWidth()
Definition at line 4561 of file KDGanttView.cpp.
◆ setLvBackgroundColor()
void KDGanttView::setLvBackgroundColor | ( | const TQColor & | c | ) |
Sets the background color of the list view.
- Parameters
-
c the background color of the list view
- See also
- lvBackgroundColor()
Definition at line 4180 of file KDGanttView.cpp.
◆ setLvVScrollBarMode()
void KDGanttView::setLvVScrollBarMode | ( | TQScrollView::ScrollBarMode | m | ) |
Sets the scrollbar mode of the listview. The default is always off. Possible values are always on, always off and auto. It only makes sense to set this to always off if setGvVScrollBarMode() is set to always on.
- Parameters
-
m the scrollbar mode.
- See also
- setGvVScrollBarMode( )
Definition at line 4592 of file KDGanttView.cpp.
◆ setMajorScaleCount()
void KDGanttView::setMajorScaleCount | ( | int | count | ) |
Sets the number of ticks in the major scale.
- Parameters
-
count the number of ticks in the major scale
Definition at line 2196 of file KDGanttView.cpp.
◆ setMaximumScale()
void KDGanttView::setMaximumScale | ( | Scale | unit | ) |
Sets the maximum allowed time scale of the lower scale of the header.
- Parameters
-
unit the unit of the lower scale of the header.
- See also
- scale()
Definition at line 1665 of file KDGanttView.cpp.
◆ setMinimumColumnWidth()
void KDGanttView::setMinimumColumnWidth | ( | int | width | ) |
Sets the minimum width that a column needs to have. If the size of the Gantt chart and the scale would make it necessary to go below this limit otherwise, the chart will automatically be made less exact.
- Parameters
-
width the minimum column width
- See also
- minimumColumnWidth()
Definition at line 1742 of file KDGanttView.cpp.
◆ setMinimumScale()
void KDGanttView::setMinimumScale | ( | Scale | unit | ) |
Sets the minimum allowed time scale of the lower scale of the header.
- Parameters
-
unit the unit of the lower scale of the header.
- See also
- scale()
Definition at line 1689 of file KDGanttView.cpp.
◆ setMinorScaleCount()
void KDGanttView::setMinorScaleCount | ( | int | count | ) |
Sets the number of ticks in the minor scale.
- Parameters
-
count the number of ticks in the minor scale
Definition at line 2220 of file KDGanttView.cpp.
◆ setNoInformationBrush()
void KDGanttView::setNoInformationBrush | ( | const TQBrush & | brush | ) |
Specifies the brush in which the 'showNoInformation' line of items should be drawn.
- Parameters
-
brush the brush of the 'showNoInformation' lines
- See also
- KDGanttViewItem::showNoInformation(), KDGanttViewItem::setShowNoInformation(), KDGanttView::noInformationBrush()
Definition at line 1530 of file KDGanttView.cpp.
◆ setPaletteBackgroundColor()
void KDGanttView::setPaletteBackgroundColor | ( | const TQColor & | col | ) |
This method is overridden for internal purposes.
Definition at line 4128 of file KDGanttView.cpp.
◆ setRepaintMode()
void KDGanttView::setRepaintMode | ( | RepaintMode | mode | ) |
Specifies whether the content should be repainted after scrolling or not.
- Parameters
-
mode If No, there is no repainting after scrolling. This is the fastest mode. If Medium, there is extra repainting after releasing the scrollbar. This provides fast scrolling with updated content after scrolling. Recommended, when repaint problems occur. This is the default value after startup. If Always, there is an extra update after every move of the scrollbar. This entails slow scrolling with updated content at all time.
Definition at line 541 of file KDGanttView.cpp.
◆ setScale()
void KDGanttView::setScale | ( | Scale | unit | ) |
Configures the unit of the lower scale of the header. The higher unit is computed automatically.
- Parameters
-
unit the unit of the lower scale of the header.
- See also
- scale()
Definition at line 1641 of file KDGanttView.cpp.
◆ setSelected()
void KDGanttView::setSelected | ( | KDGanttViewItem * | item, |
bool | selected | ||
) |
Calls to this method are passed through to the underlying TQListView.
Definition at line 3920 of file KDGanttView.cpp.
◆ setShapes()
void KDGanttView::setShapes | ( | KDGanttViewItem::Type | type, |
KDGanttViewItem::Shape | start, | ||
KDGanttViewItem::Shape | middle, | ||
KDGanttViewItem::Shape | end, | ||
bool | overwriteExisting = true |
||
) |
Sets the shapes for a certain type of Gantt item. Not all items use all three shapes (e.g., only summary items use the middle shape).
This setting overrides any shape settings made on individual items. These settings will be taken as initial values of any newly created item of this certain type. See also the documentation of the KDGanttViewItem class.
- Parameters
-
type the type of Gantt items for which to set the shapes start the shape to use for the beginning of the item middle the shape to use for the middle of the item end the shape to use for the end of the item overwriteExisting if true, overwrites existing shape settings in the individual items
- See also
- shapes()
Definition at line 1318 of file KDGanttView.cpp.
◆ setShowHeaderPopupMenu()
void KDGanttView::setShowHeaderPopupMenu | ( | bool | show = true , |
bool | showZoom = true , |
||
bool | showScale = true , |
||
bool | showTime = true , |
||
bool | showYear = true , |
||
bool | showGrid = true , |
||
bool | showPrint = false |
||
) |
Specifies whether the configure popup menu should be shown on right click on the time header widget. This menu lets the user quickly change the zoom factor, the scale mode (minute, hour, day, week, month, auto) , the time format, the year format, the grid format, and printing. The default setting is not to show the popup menu. This functionality must be enabled explicitly by the application developer. You can disable each submenu of the popmenu.
- Parameters
-
show true in order to show the popup menu, false in order not to. The default is true. showZoom show the zoom submenu, default: true showScale show the scale submenu, default: true showTime show the time format submenu, default: true showYear show the year format submenu, default: true showGrid show the grid submenu, default: true showPrint show the print submenu, default: false
Definition at line 1244 of file KDGanttView.cpp.
◆ setShowLegend()
void KDGanttView::setShowLegend | ( | bool | show | ) |
Specifies whether the legend should be shown or not. Besides setting this programmatically, the user can also show/hide the legend by using the button provided for this purpose.
- Parameters
-
show force legend to be shown
- See also
- showLegend()
Definition at line 661 of file KDGanttView.cpp.
◆ setShowLegendButton()
void KDGanttView::setShowLegendButton | ( | bool | show | ) |
Specifies whether the legend button should be visible. By default, it is visible.
- Parameters
-
show true to show the legend button, false to hide it
- See also
- showLegendButton()
Definition at line 362 of file KDGanttView.cpp.
◆ setShowListView()
void KDGanttView::setShowListView | ( | bool | show | ) |
Specifies whether the listview of the Gantt view should be shown or not.
- Parameters
-
show pass true in order to show the listview and false in order to hide it.
- See also
- showListView()
Definition at line 689 of file KDGanttView.cpp.
◆ setShowMajorTicks()
void KDGanttView::setShowMajorTicks | ( | bool | show | ) |
Hides/shows the grid for the major ticks of the time header in the gantt view.
- Parameters
-
show true in order to show ticks, false in order to hide them. If show is true, setShowMinorTicks( false ) is performed automatically to hide the grid of the minor ticks. In order to show now grid, call setShowMinorTicks( false ) and setShowMajorTicks( false ).
Definition at line 1822 of file KDGanttView.cpp.
◆ setShowMinorTicks()
void KDGanttView::setShowMinorTicks | ( | bool | show | ) |
Hides/shows the grid for the minor ticks of the time header in the gantt view.
- Parameters
-
show true in order to show ticks, false in order to hide them. If show is true, setShowMajorTicks( false ) is performed automatically to hide the grid of the major ticks. In order to show now grid, call setShowMinorTicks( false ) and setShowMajorTicks( false ).
Definition at line 1852 of file KDGanttView.cpp.
◆ setShowTaskLinks()
void KDGanttView::setShowTaskLinks | ( | bool | show | ) |
Specifies whether task links should be shown.
- Parameters
-
show true for showing task links, false for not showing them
- See also
- showTaskLinks(), KDGanttViewTaskLink
Definition at line 1183 of file KDGanttView.cpp.
◆ setShowTimeTablePopupMenu()
void KDGanttView::setShowTimeTablePopupMenu | ( | bool | show | ) |
Specifies whether the add item popup menu should be shown on right click on the time table widget. This menu lets the user quickly add new items to the Gantt view (as root, as child or after an item). It also offers cutting and pasting of items.
The default setting is that the popup menu is not shown. It must be enabled by the program.
- Parameters
-
show true in order to show popup menu, false in order not to
Definition at line 1283 of file KDGanttView.cpp.
◆ setTextColor()
void KDGanttView::setTextColor | ( | const TQColor & | color | ) |
Sets the color used for texts in the Gantt chart. Overrides all individual settings of the Gantt items.
- Parameters
-
color the text color to use
- See also
- textColor()
Definition at line 1498 of file KDGanttView.cpp.
◆ setTimeHeaderBackgroundColor()
void KDGanttView::setTimeHeaderBackgroundColor | ( | const TQColor & | c | ) |
Sets the background color of the time header.
- Parameters
-
c the background color of the time header.
- See also
- timeHeaderBackgroundColor()
Definition at line 4153 of file KDGanttView.cpp.
◆ setTimelineToEnd()
void KDGanttView::setTimelineToEnd | ( | ) |
Sets the timeline to the horizon end.
Definition at line 1127 of file KDGanttView.cpp.
◆ setTimelineToStart()
void KDGanttView::setTimelineToStart | ( | ) |
Sets the timeline to the horizon start.
Definition at line 1118 of file KDGanttView.cpp.
◆ setUpdateEnabled()
void KDGanttView::setUpdateEnabled | ( | bool | enable | ) |
Enables or disables updating of the content of the Gantt view. To avoid flickering in the Gantt view while inserting large amounts of Gantt items, you should call
bool upd = KDGanttView::getUpdateEnabled(); KDGanttView::settUpdateEnabled( false ); ... insert items here ... KDGanttView::settUpdateEnabled( upd );
With this code, you avoid unwanted side effects with other parts in your code, where you disable (and re-enable) the update.
When calling setUpdateEnabled( true ), all the content is recomputed, resized, and updated.
Before calling show() for the first time, updating is disabled. When calling show(), updating is automatically enabled.
- Parameters
-
enable if true, the content of the Gantt view is updated after every insertion of a new item.
- See also
- getUpdateEnabled()
Definition at line 230 of file KDGanttView.cpp.
◆ setWeekdayBackgroundColor()
void KDGanttView::setWeekdayBackgroundColor | ( | const TQColor & | color, |
int | weekday | ||
) |
Specifies the background color for weekday days. If no individual days are visible on the Gantt chart, this method has no visible effect. The days are specified as an intervals of integer values where 1 means Monday and 7 means Sunday.
- Parameters
-
color the background color to use for weekend days. weekday the day of the week (Monday = 1, Sunday = 7)
Definition at line 2047 of file KDGanttView.cpp.
◆ setWeekendBackgroundColor()
void KDGanttView::setWeekendBackgroundColor | ( | const TQColor & | color | ) |
Specifies the background color for weekend days. If no individual days are visible on the Gantt chart, this method has no visible effect.
- Parameters
-
color the background color to use for weekend days.
Definition at line 2019 of file KDGanttView.cpp.
◆ setWeekendDays()
void KDGanttView::setWeekendDays | ( | int | start, |
int | end | ||
) |
Defines which days are considered weekends. The days are specified as an interval of integer values where 1 means Monday and 7 means Sunday. In order to define a weekend from Sunday to Monday, specify (7,1).
- Parameters
-
start the first day of the weekend end the last day of the weekend
Definition at line 2077 of file KDGanttView.cpp.
◆ setYearFormat()
void KDGanttView::setYearFormat | ( | YearFormat | format | ) |
Specifies the format in which to display years. If no years are shown, this method has no effect.
- Parameters
-
format the year format
- See also
- yearFormat(), setHourFormat(), hourFormat()
Definition at line 1767 of file KDGanttView.cpp.
◆ setZoomFactor()
void KDGanttView::setZoomFactor | ( | double | factor, |
bool | absolute | ||
) |
Zooms into the Gantt chart. Values less than 1 mean zooming in, values greater than 1 mean zooming out. A zooming factor of exactly 1.0 means original size.
- Parameters
-
factor the zoom factor absolute if true, factor is interpreted absolutely, if false, factor is interpreted relatively to the current zoom factor
- See also
- zoomToFit()
- zoomToSelection()
- zoomFactor()
Definition at line 1014 of file KDGanttView.cpp.
◆ shapes()
bool KDGanttView::shapes | ( | KDGanttViewItem::Type | type, |
KDGanttViewItem::Shape & | start, | ||
KDGanttViewItem::Shape & | middle, | ||
KDGanttViewItem::Shape & | end | ||
) | const |
Queries the shapes for a particular type of Gantt item.
- Parameters
-
type the type of Gantt items for which to query the shapes start the start shape is returned in this parameter middle the middle shape is returned in this parameter end the end shape is returned in this parameter
- Returns
- true if there was a general shape set for the specified type. If the return value is false, the values of the three shape parameters are undefined.
- See also
- setShapes()
Definition at line 1351 of file KDGanttView.cpp.
◆ show()
|
virtual |
Updates the content of the GanttView and shows it. Automatically sets setUpdateEnabled( true ).
- See also
- setUpdateEnabled()
Definition at line 282 of file KDGanttView.cpp.
◆ showHeaderPopupMenu()
bool KDGanttView::showHeaderPopupMenu | ( | ) | const |
Returns whether the configure popup menu should be shown on right click on the time header widget.
- Returns
- true if the popup menu should be shown
Definition at line 1263 of file KDGanttView.cpp.
◆ showLegend()
bool KDGanttView::showLegend | ( | ) | const |
Returns whether the legend is currently shown. The visibility of the legend can be changed both by setShowLegend(), and interactively by the user.
- Returns
- true if the legend is currently visible
- See also
- setShowLegend()
Definition at line 675 of file KDGanttView.cpp.
◆ showLegendButton()
bool KDGanttView::showLegendButton | ( | ) | const |
Returns whether the legend button is visible.
- Returns
- whether the legend button is visible
- See also
- setShowLegendButton()
Definition at line 379 of file KDGanttView.cpp.
◆ showListView()
bool KDGanttView::showListView | ( | ) | const |
Returns whether the listview of the Gantt view is shown or not.
- Returns
- true if the listview is shown
- See also
- setShowListView()
Definition at line 706 of file KDGanttView.cpp.
◆ showMajorTicks()
bool KDGanttView::showMajorTicks | ( | ) | const |
Returns whether the grid is shown on the major scale.
- Returns
- true if ticks are shown on the major scale
Definition at line 1834 of file KDGanttView.cpp.
◆ showMinorTicks()
bool KDGanttView::showMinorTicks | ( | ) | const |
Returns whether ticks are shown on the minor scale.
- Returns
- true if ticks are shown on the minor scale
Definition at line 1864 of file KDGanttView.cpp.
◆ showTaskLinks()
bool KDGanttView::showTaskLinks | ( | ) | const |
Returns whether task links should be shown.
- Returns
- true if task links are shown, false otherwise
- See also
- setShowTaskLinks(), KDGanttViewTaskLink
Definition at line 1196 of file KDGanttView.cpp.
◆ showTimeTablePopupMenu()
bool KDGanttView::showTimeTablePopupMenu | ( | ) | const |
Returns whether the add item popup menu should be shown on right click on the time table widget.
- Returns
- true if the popup menu should be shown
Definition at line 1295 of file KDGanttView.cpp.
◆ sizeHint()
|
virtual |
Returns a useful size for the view. Returned width: sizeHint().width() of the list view + width of TimeTable Returned height: height() of TimeHeader + height() of TimeTable + height() of Legend (if shown)
Reimplemented from KDGanttMinimizeSplitter.
Definition at line 321 of file KDGanttView.cpp.
◆ startDrag()
|
protectedvirtual |
Implements a pass-through to the list view.
Definition at line 4119 of file KDGanttView.cpp.
◆ taskLinkDoubleClicked
|
signal |
This signal is emitted when the user double-clicks a task link.
◆ taskLinkGroups()
TQPtrList< KDGanttViewTaskLinkGroup > KDGanttView::taskLinkGroups | ( | ) | const |
Returns the list of task link groups in the Gantt view.
- Returns
- the list of task link groups in the Gantt view
Definition at line 2500 of file KDGanttView.cpp.
◆ taskLinkLeftClicked
|
signal |
This signal is emitted when the user clicks on a task link with the left mouse button.
◆ taskLinkMidClicked
|
signal |
This signal is emitted when the user clicks on a task link with the middle mouse button.
◆ taskLinkRightClicked
|
signal |
This signal is emitted when the user clicks on a task link with the right mouse button.
◆ taskLinks()
TQPtrList< KDGanttViewTaskLink > KDGanttView::taskLinks | ( | ) | const |
Returns the list of task links in the Gantt view.
- Returns
- the list of task links in the Gantt view
Definition at line 2488 of file KDGanttView.cpp.
◆ textColor()
TQColor KDGanttView::textColor | ( | ) | const |
Returns the color used for texts in the Gantt chart.
- Returns
- the color used for texts in the Gantt chart.
- See also
- setTextColor()
Definition at line 1514 of file KDGanttView.cpp.
◆ timeHeaderBackgroundColor()
TQColor KDGanttView::timeHeaderBackgroundColor | ( | ) | const |
Returns the background color of the time header.
- Returns
- the background color of the time header
- See also
- setTimeHeaderBackgroundColor()
Definition at line 4216 of file KDGanttView.cpp.
◆ timeHeaderWidget()
|
inline |
Definition at line 349 of file KDGanttView.h.
◆ timeIntervallSelected
|
signal |
- Deprecated:
- This signal is deprecated, do not use it in new code; use timeIntervalSelected() instead. timeIntervallSelected() will be removed in future versions.
◆ timeIntervalSelected
|
signal |
This signal is emitted when the user selects a time interval with the mouse on the time header connect this signal to the slot void zoomToSelection( const TQDateTime& start, const TQDateTime& end) to obtain automatic zooming.
◆ timeTableWidget()
|
inline |
Definition at line 348 of file KDGanttView.h.
◆ weekdayBackgroundColor()
TQColor KDGanttView::weekdayBackgroundColor | ( | int | weekday | ) | const |
Returns the background color for weekday days.
- Parameters
-
weekday the day of the week (Monday = 1, Sunday = 7)
- Returns
- the background color for weekend days
Definition at line 2060 of file KDGanttView.cpp.
◆ weekendBackgroundColor()
TQColor KDGanttView::weekendBackgroundColor | ( | ) | const |
Returns the background color for weekend days.
- Returns
- the background color for weekend days
Definition at line 2031 of file KDGanttView.cpp.
◆ weekendDays()
void KDGanttView::weekendDays | ( | int & | start, |
int & | end | ||
) | const |
Returns which days are considered weekends.
- Parameters
-
start in this parameter, the first day of the weekend is returned end in this parameter, the end day of the weekend is returned
Definition at line 2090 of file KDGanttView.cpp.
◆ yearFormat()
KDGanttView::YearFormat KDGanttView::yearFormat | ( | ) | const |
Returns the format in which to display years.
- Returns
- the year format
- See also
- setYearFormat(), setHourFormat(), hourFormat()
Definition at line 1779 of file KDGanttView.cpp.
◆ zoomFactor()
double KDGanttView::zoomFactor | ( | ) | const |
Returns the current zoom factor.
- Returns
- the current zoom factor
- See also
- zoomToFit(), zoomToSelection(), setZoomFactor()
Definition at line 1027 of file KDGanttView.cpp.
◆ zoomToFit()
void KDGanttView::zoomToFit | ( | ) |
Zooms such that the Gantt chart is less than the available space of the widget.
Definition at line 1041 of file KDGanttView.cpp.
◆ zoomToSelection
|
slot |
Zooms so that at least the selected time period is visible after the zoom.
- Parameters
-
start the new font of the widget end the new font of the widget
- See also
- setZoomFactor()
- zoomFactor()
- zoomToFit()
Definition at line 1058 of file KDGanttView.cpp.
Friends And Related Function Documentation
◆ KDGanttCanvasView
|
friend |
Definition at line 442 of file KDGanttView.h.
◆ KDGanttViewCalendarItem
|
friend |
Definition at line 448 of file KDGanttView.h.
◆ KDGanttViewEventItem
|
friend |
Definition at line 443 of file KDGanttView.h.
◆ KDGanttViewItem
|
friend |
Definition at line 444 of file KDGanttView.h.
◆ KDGanttViewSummaryItem
|
friend |
Definition at line 446 of file KDGanttView.h.
◆ KDGanttViewTaskItem
|
friend |
Definition at line 445 of file KDGanttView.h.
◆ KDGanttViewTaskLink
|
friend |
Definition at line 447 of file KDGanttView.h.
◆ KDGanttViewTaskLinkGroup
|
friend |
Definition at line 452 of file KDGanttView.h.
◆ KDLegendWidget
|
friend |
Definition at line 453 of file KDGanttView.h.
◆ KDListView
|
friend |
Definition at line 451 of file KDGanttView.h.
◆ KDTimeHeaderWidget
|
friend |
Definition at line 450 of file KDGanttView.h.
◆ KDTimeTableWidget
|
friend |
Definition at line 449 of file KDGanttView.h.
The documentation for this class was generated from the following files: