#include <KDGanttViewTaskItem.h>
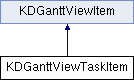
Public Member Functions | |
KDGanttViewTaskItem (KDGanttView *view, const TQString &lvtext=TQString(), const TQString &name=TQString()) | |
KDGanttViewTaskItem (KDGanttViewItem *parent, const TQString &lvtext=TQString(), const TQString &name=TQString()) | |
KDGanttViewTaskItem (KDGanttView *view, KDGanttViewItem *after, const TQString &lvtext=TQString(), const TQString &name=TQString()) | |
KDGanttViewTaskItem (KDGanttViewItem *parent, KDGanttViewItem *after, const TQString &lvtext=TQString(), const TQString &name=TQString()) | |
virtual | ~KDGanttViewTaskItem () |
void | setStartTime (const TQDateTime &start) |
void | setEndTime (const TQDateTime &end) |
![]() | |
virtual | ~KDGanttViewItem () |
Type | type () const |
void | setEnabled (bool on) |
bool | enabled () const |
virtual void | setOpen (bool o) |
void | setItemVisible (bool on) |
bool | itemVisible () const |
void | setEditable (bool editable) |
bool | editable () const |
void | setShowNoInformation (bool show) |
bool | showNoInformation () |
void | setDisplaySubitemsAsGroup (bool show) |
bool | displaySubitemsAsGroup () const |
void | setPriority (int prio) |
int | priority () |
virtual void | setStartTime (const TQDateTime &start) |
TQDateTime | startTime () const |
virtual void | setEndTime (const TQDateTime &end) |
TQDateTime | endTime () const |
void | setText (const TQString &text) |
TQString | text () const |
void | setListViewText (const TQString &text, int column=0) |
void | setListViewText (int column, const TQString &text) |
TQString | listViewText (int column=0) const |
void | setFont (const TQFont &font) |
TQFont | font () const |
void | setTooltipText (const TQString &text) |
TQString | tooltipText () const |
void | setWhatsThisText (const TQString &text) |
TQString | whatsThisText () const |
void | setPixmap (int column, const TQPixmap &pixmap) |
void | setPixmap (const TQPixmap &pixmap) |
const TQPixmap * | pixmap (int column=0) const |
void | setHighlight (bool) |
bool | highlight () const |
bool | subitemIsCalendar () const |
void | setShapes (Shape start, Shape middle, Shape end) |
void | shapes (Shape &start, Shape &middle, Shape &end) const |
void | setDefaultColor (const TQColor &) |
TQColor | defaultColor () const |
void | setColors (const TQColor &start, const TQColor &middle, const TQColor &end) |
void | colors (TQColor &start, TQColor &middle, TQColor &end) const |
void | setDefaultHighlightColor (const TQColor &) |
TQColor | defaultHighlightColor () const |
void | setHighlightColors (const TQColor &start, const TQColor &middle, const TQColor &end) |
void | highlightColors (TQColor &start, TQColor &middle, TQColor &end) const |
void | setTextColor (const TQColor &color) |
TQColor | textColor () const |
void | setProgress (int percent) |
void | setFloatStartTime (const TQDateTime &start) |
void | setFloatEndTime (const TQDateTime &end) |
KDGanttViewItem * | firstChild () const |
KDGanttViewItem * | nextSibling () const |
KDGanttViewItem * | parent () const |
KDGanttViewItem * | itemAbove () |
KDGanttViewItem * | itemBelow (bool includeDisabled=true) |
KDGanttViewItem * | getChildByName (const TQString &name) |
TQString | name () const |
void | createNode (TQDomDocument &doc, TQDomElement &parentElement) |
void | setMoveable (bool m) |
bool | isMoveable () const |
void | setResizeable (bool r) |
bool | isResizeable () const |
Protected Member Functions | |
void | showItem (bool show=true, int coordY=0) |
![]() | |
KDGanttViewItem (Type type, KDGanttView *view, const TQString &lvtext=TQString(), const TQString &name=TQString()) | |
KDGanttViewItem (Type type, KDGanttViewItem *parent, const TQString &lvtext=TQString(), const TQString &name=TQString()) | |
KDGanttViewItem (Type type, KDGanttView *view, KDGanttViewItem *after, const TQString &lvtext=TQString(), const TQString &name=TQString()) | |
KDGanttViewItem (Type type, KDGanttViewItem *parent, KDGanttViewItem *after, const TQString &lvtext=TQString(), const TQString &name=TQString()) | |
void | updateCanvasItems () |
int | getCoordY () |
TQDateTime | myChildStartTime () |
TQDateTime | myChildEndTime () |
void | generateAndInsertName (const TQString &name) |
void | moveTextCanvas (int x, int y) |
Additional Inherited Members | |
![]() | |
enum | Type { Event , Task , Summary } |
enum | Shape { TriangleDown , TriangleUp , Diamond , Square , Circle } |
![]() | |
static KDGanttViewItem * | find (const TQString &name) |
static KDGanttViewItem * | createFromDomElement (KDGanttView *view, TQDomElement &element) |
static KDGanttViewItem * | createFromDomElement (KDGanttView *view, KDGanttViewItem *previous, TQDomElement &element) |
static KDGanttViewItem * | createFromDomElement (KDGanttViewItem *parent, TQDomElement &element) |
static KDGanttViewItem * | createFromDomElement (KDGanttViewItem *parent, KDGanttViewItem *previous, TQDomElement &element) |
![]() | |
bool | isVisibleInGanttView |
KDCanvasLine * | startLine |
KDCanvasLine * | endLine |
KDCanvasLine * | startLineBack |
KDCanvasLine * | endLineBack |
KDCanvasLine * | actualEnd |
KDCanvasPolygonItem * | startShape |
KDCanvasPolygonItem * | midShape |
KDCanvasPolygonItem * | endShape |
KDCanvasPolygonItem * | progressShape |
KDCanvasPolygonItem * | startShapeBack |
KDCanvasPolygonItem * | midShapeBack |
KDCanvasPolygonItem * | endShapeBack |
KDCanvasPolygonItem * | floatStartShape |
KDCanvasPolygonItem * | floatEndShape |
KDGanttView * | myGanttView |
KDCanvasText * | textCanvas |
TQString | textCanvasText |
TQDateTime | myStartTime |
TQDateTime | myEndTime |
bool | isHighlighted |
bool | isEditable |
int | myItemSize |
bool | blockUpdating |
int | myProgress |
TQDateTime | myFloatStartTime |
TQDateTime | myFloatEndTime |
Detailed Description
This class represents calendar items in Gantt charts.
A calendar item in a Gantt chart has no start/end shape, it is displayed as a rectangle. You can set the colors as usual, where only the first argument of setColors( col, col, col ) is important. If the start time is equal to the end time, the item is displayed as ø, showing that there is no time interval set.
For a KDGanttViewTaskItem, the text, setted by setText(), is shown in the item itself and the text is truncated automatically to fit in the item. For all other item types, the text is shown right of the item.
Definition at line 43 of file KDGanttViewTaskItem.h.
Constructor & Destructor Documentation
◆ KDGanttViewTaskItem() [1/4]
KDGanttViewTaskItem::KDGanttViewTaskItem | ( | KDGanttView * | view, |
const TQString & | lvtext = TQString() , |
||
const TQString & | name = TQString() |
||
) |
Constructs an empty Gantt item of type event.
- Parameters
-
view the Gantt view to insert this item into lvtext the text to show in the listview name the name by which the item can be identified. If no name is specified, a unique name will be generated
Definition at line 69 of file KDGanttViewTaskItem.cpp.
◆ KDGanttViewTaskItem() [2/4]
KDGanttViewTaskItem::KDGanttViewTaskItem | ( | KDGanttViewItem * | parent, |
const TQString & | lvtext = TQString() , |
||
const TQString & | name = TQString() |
||
) |
Constructs an empty Gantt item of type event.
- Parameters
-
parent a parent item under which this one goes lvtext the text to show in the list view name the name by which the item can be identified. If no name is specified, a unique name will be generated
Definition at line 87 of file KDGanttViewTaskItem.cpp.
◆ KDGanttViewTaskItem() [3/4]
KDGanttViewTaskItem::KDGanttViewTaskItem | ( | KDGanttView * | view, |
KDGanttViewItem * | after, | ||
const TQString & | lvtext = TQString() , |
||
const TQString & | name = TQString() |
||
) |
Constructs an empty Gantt item of type event.
- Parameters
-
view the Gantt view to insert this item into after another item at the same level behind which this one should go lvtext the text to show in the list view name the name by which the item can be identified. If no name is specified, a unique name will be generated
Definition at line 105 of file KDGanttViewTaskItem.cpp.
◆ KDGanttViewTaskItem() [4/4]
KDGanttViewTaskItem::KDGanttViewTaskItem | ( | KDGanttViewItem * | parent, |
KDGanttViewItem * | after, | ||
const TQString & | lvtext = TQString() , |
||
const TQString & | name = TQString() |
||
) |
Constructs an empty Gantt item of type event.
- Parameters
-
parent a parent item under which this one goes after another item at the same level behind which this one should go lvtext the text to show in the listview name the name by which the item can be identified. If no name is specified, a unique name will be generated
Definition at line 124 of file KDGanttViewTaskItem.cpp.
◆ ~KDGanttViewTaskItem()
|
virtual |
The destructor.
Definition at line 137 of file KDGanttViewTaskItem.cpp.
Member Function Documentation
◆ setEndTime()
|
virtual |
Specifies the end time of this item. The parameter must be valid and non-null. If the parameter is invalid or null, no value is set. If the end time is less the start time, the start time is set to this end time automatically.
- Parameters
-
end the end time
- See also
- setStartTime(), startTime(), endTime()
Reimplemented from KDGanttViewItem.
Definition at line 152 of file KDGanttViewTaskItem.cpp.
◆ setStartTime()
|
virtual |
Specifies the start time of this item. The parameter must be valid and non-null. If the parameter is invalid or null, no value is set. If the start time is greater than the end time, the end time is set to this start time automatically.
- Parameters
-
start the start time
- See also
- startTime(), setEndTime(), endTime()
Reimplemented from KDGanttViewItem.
Definition at line 171 of file KDGanttViewTaskItem.cpp.
◆ showItem()
|
protectedvirtual |
Reimplemented from KDGanttViewItem.
Definition at line 199 of file KDGanttViewTaskItem.cpp.
The documentation for this class was generated from the following files: