#include <addressbook.h>
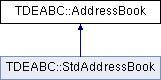
Classes | |
class | ConstIterator |
class | Iterator |
Signals | |
void | addressBookChanged (AddressBook *addressBook) |
void | addressBookLocked (AddressBook *addressBook) |
void | addressBookUnlocked (AddressBook *addressBook) |
void | loadingFinished (Resource *resource) |
void | savingFinished (Resource *resource) |
Public Member Functions | |
AddressBook () | |
AddressBook (const TQString &config) | |
virtual | ~AddressBook () |
Ticket * | requestSaveTicket (Resource *resource=0) |
void | releaseSaveTicket (Ticket *ticket) |
bool | load () |
bool | asyncLoad () |
bool | save (Ticket *ticket) |
bool | asyncSave (Ticket *ticket) |
ConstIterator | begin () const |
Iterator | begin () |
ConstIterator | end () const |
Iterator | end () |
void | clear () |
void | insertAddressee (const Addressee &addr) |
void | removeAddressee (const Addressee &addr) |
void | removeAddressee (const Iterator &it) |
Iterator | find (const Addressee &addr) |
Addressee | findByUid (const TQString &uid) |
Addressee::List | allAddressees () |
Addressee::List | findByName (const TQString &name) |
Addressee::List | findByEmail (const TQString &email) |
Addressee::List | findByCategory (const TQString &category) |
virtual TQString | identifier () |
Field::List | fields (int category=Field::All) |
bool | addCustomField (const TQString &label, int category=Field::All, const TQString &key=TQString::null, const TQString &app=TQString::null) |
bool | addResource (Resource *resource) |
bool | removeResource (Resource *resource) |
TQPtrList< Resource > | resources () |
void | setErrorHandler (ErrorHandler *errorHandler) |
void | error (const TQString &msg) |
void | cleanUp () TDE_DEPRECATED |
void | dump () const |
void | emitAddressBookLocked () |
void | emitAddressBookUnlocked () |
void | emitAddressBookChanged () |
bool | loadingHasFinished () const |
Protected Slots | |
void | resourceLoadingFinished (Resource *) |
void | resourceSavingFinished (Resource *) |
void | resourceLoadingError (Resource *, const TQString &) |
void | resourceSavingError (Resource *, const TQString &) |
Protected Member Functions | |
void | deleteRemovedAddressees () |
void | setStandardResource (Resource *) |
Resource * | standardResource () |
KRES::Manager< Resource > * | resourceManager () |
Friends | |
class | StdAddressBook |
TQ_OBJECT friend KABC_EXPORT TQDataStream & | operator<< (TQDataStream &, const AddressBook &) |
KABC_EXPORT TQDataStream & | operator>> (TQDataStream &, AddressBook &) |
Detailed Description
Address Book.
This class provides access to a collection of address book entries.
Definition at line 43 of file addressbook.h.
Constructor & Destructor Documentation
◆ AddressBook() [1/2]
AddressBook::AddressBook | ( | ) |
Constructs an address book object.
You have to add the resources manually before calling load().
Definition at line 304 of file addressbook.cpp.
◆ AddressBook() [2/2]
AddressBook::AddressBook | ( | const TQString & | config | ) |
Constructs an address book object.
The resources are loaded automatically.
- Parameters
-
config The config file which contains the resource settings.
Definition at line 314 of file addressbook.cpp.
◆ ~AddressBook()
|
virtual |
Destructor.
Definition at line 328 of file addressbook.cpp.
Member Function Documentation
◆ addCustomField()
bool AddressBook::addCustomField | ( | const TQString & | label, |
int | category = Field::All , |
||
const TQString & | key = TQString::null , |
||
const TQString & | app = TQString::null |
||
) |
Add custom field to address book.
- Parameters
-
label User visible label of the field. category Ored list of field categories. key Identifier used as key for reading and writing the field. app String used as application key for reading and writing the field.
Definition at line 669 of file addressbook.cpp.
◆ addResource()
bool AddressBook::addResource | ( | Resource * | resource | ) |
Adds a resource to the address book.
- Parameters
-
resource The resource you want to add.
- Returns
- Whether opening the resource was successfully.
Definition at line 704 of file addressbook.cpp.
◆ addressBookChanged
|
signal |
Emitted when one of the resources discovered a change in its backend or the asynchronous loading of all resources has finished.
You should connect to this signal to update the presentation of the contact data in your application.
- Parameters
-
addressBook The address book which emitted this signal.
◆ addressBookLocked
|
signal |
Emitted when one of the resources has been locked for writing.
- Parameters
-
addressBook The address book which emitted this signal.
◆ addressBookUnlocked
|
signal |
Emitted when one of the resources has been unlocked.
You should connect to this signal if you want to save your changes to a resource which is currently locked, and want to get notified when saving is possible again.
- Parameters
-
addressBook The address book which emitted this signal.
◆ allAddressees()
Addressee::List AddressBook::allAddressees | ( | ) |
Returns a list of all addressees in the address book.
Definition at line 581 of file addressbook.cpp.
◆ asyncLoad()
bool AddressBook::asyncLoad | ( | ) |
Loads all addressees asynchronously.
This function returns immediately and emits the addressBookChanged() signal as soon as the loading has finished.
- Returns
- Whether the synchronous part of loading was successfully.
Definition at line 354 of file addressbook.cpp.
◆ asyncSave()
bool AddressBook::asyncSave | ( | Ticket * | ticket | ) |
Saves all addressees of one resource asynchronously.
If the save is successfull the ticket is deleted.
- Parameters
-
ticket The ticket returned by requestSaveTicket().
- Returns
- Whether the synchronous part of saving was successfully.
Definition at line 387 of file addressbook.cpp.
◆ begin() [1/2]
AddressBook::Iterator AddressBook::begin | ( | ) |
This is an overloaded member function, provided for convenience.
It behaves essentially like the above function.
Definition at line 401 of file addressbook.cpp.
◆ begin() [2/2]
AddressBook::ConstIterator AddressBook::begin | ( | ) | const |
Returns an iterator pointing to the first addressee of address book.
This iterator equals end() if the address book is empty.
Definition at line 428 of file addressbook.cpp.
◆ cleanUp()
void AddressBook::cleanUp | ( | ) |
- Deprecated:
- There is no need to call this function anymore.
Definition at line 803 of file addressbook.cpp.
◆ clear()
void AddressBook::clear | ( | ) |
Removes all addressees from the address book.
Definition at line 481 of file addressbook.cpp.
◆ deleteRemovedAddressees()
|
protected |
Definition at line 783 of file addressbook.cpp.
◆ dump()
void AddressBook::dump | ( | ) | const |
Used for debug output.
This function prints out the list of all addressees to kdDebug(5700).
Definition at line 625 of file addressbook.cpp.
◆ emitAddressBookChanged()
|
inline |
Definition at line 354 of file addressbook.h.
◆ emitAddressBookLocked()
|
inline |
Definition at line 352 of file addressbook.h.
◆ emitAddressBookUnlocked()
|
inline |
Definition at line 353 of file addressbook.h.
◆ end() [1/2]
AddressBook::Iterator AddressBook::end | ( | ) |
This is an overloaded member function, provided for convenience.
It behaves essentially like the above function.
Definition at line 455 of file addressbook.cpp.
◆ end() [2/2]
AddressBook::ConstIterator AddressBook::end | ( | ) | const |
Returns an iterator pointing to the last addressee of address book.
This iterator equals begin() if the address book is empty.
Definition at line 468 of file addressbook.cpp.
◆ error()
void AddressBook::error | ( | const TQString & | msg | ) |
Shows GUI independent error messages.
- Parameters
-
msg The error message that shall be displayed.
Definition at line 772 of file addressbook.cpp.
◆ fields()
Field::List AddressBook::fields | ( | int | category = Field::All | ) |
Returns a list of all Fields known to the address book which are associated with the given field category.
Definition at line 651 of file addressbook.cpp.
◆ find()
AddressBook::Iterator AddressBook::find | ( | const Addressee & | addr | ) |
Returns an iterator pointing to the specified addressee.
It will return end() if no addressee matched.
- Parameters
-
addr The addresee you are looking for.
Definition at line 558 of file addressbook.cpp.
◆ findByCategory()
Addressee::List AddressBook::findByCategory | ( | const TQString & | category | ) |
Searches all addressees which belongs to the specified category.
- Parameters
-
category The category you are looking for.
- Returns
- A list of all matching addressees.
Definition at line 614 of file addressbook.cpp.
◆ findByEmail()
Addressee::List AddressBook::findByEmail | ( | const TQString & | ) |
Searches all addressees which match the specified email address.
- Parameters
-
email The email address you are looking for.
- Returns
- A list of all matching addressees.
Definition at line 603 of file addressbook.cpp.
◆ findByName()
Addressee::List AddressBook::findByName | ( | const TQString & | name | ) |
Searches all addressees which match the specified name.
- Parameters
-
name The name you are looking for.
- Returns
- A list of all matching addressees.
Definition at line 592 of file addressbook.cpp.
◆ findByUid()
Addressee AddressBook::findByUid | ( | const TQString & | uid | ) |
Searches an addressee with the specified unique identifier.
- Parameters
-
uid The unique identifier you are looking for.
- Returns
- The addressee with the specified unique identifier or an empty addressee.
Definition at line 569 of file addressbook.cpp.
◆ identifier()
|
virtual |
Returns a string identifying this addressbook.
The identifier is created by concatenation of the resource identifiers.
Definition at line 637 of file addressbook.cpp.
◆ insertAddressee()
void AddressBook::insertAddressee | ( | const Addressee & | addr | ) |
Insert an addressee into the address book.
If an addressee with the same unique id already exists, it is replaced by the new one, otherwise it is appended.
- Parameters
-
addr The addressee which shall be insert.
Definition at line 518 of file addressbook.cpp.
◆ load()
bool AddressBook::load | ( | ) |
Loads all addressees synchronously.
- Returns
- Whether the loading was successfully.
Definition at line 336 of file addressbook.cpp.
◆ loadingFinished
|
signal |
Emitted when the asynchronous loading of one resource has finished after calling asyncLoad().
- Parameters
-
resource The resource which emitted this signal.
◆ loadingHasFinished()
bool AddressBook::loadingHasFinished | ( | ) | const |
Returns true when the loading of the addressbook has finished, otherwise false.
- Since
- 3.5
Definition at line 807 of file addressbook.cpp.
◆ releaseSaveTicket()
void AddressBook::releaseSaveTicket | ( | Ticket * | ticket | ) |
Releases the ticket requested previously with requestSaveTicket().
Call this function, if you want to release a ticket without saving.
Definition at line 508 of file addressbook.cpp.
◆ removeAddressee() [1/2]
void AddressBook::removeAddressee | ( | const Addressee & | addr | ) |
Removes an addressee from the address book.
- Parameters
-
addr The addressee which shall be removed.
Definition at line 546 of file addressbook.cpp.
◆ removeAddressee() [2/2]
void AddressBook::removeAddressee | ( | const Iterator & | it | ) |
This is an overloaded member function, provided for convenience.
It behaves essentially like the above function.
- Parameters
-
it An iterator pointing to the addressee which shall be removed.
Definition at line 552 of file addressbook.cpp.
◆ removeResource()
bool AddressBook::removeResource | ( | Resource * | resource | ) |
Removes a resource from the address book.
- Parameters
-
resource The resource you want to remove.
- Returns
- Whether closing the resource was successfully.
Definition at line 727 of file addressbook.cpp.
◆ requestSaveTicket()
Ticket * AddressBook::requestSaveTicket | ( | Resource * | resource = 0 | ) |
Requests a ticket for saving the addressbook.
Calling this function locks the addressbook for all other processes. You need the returned ticket object for calling the save() function.
- Parameters
-
resource A pointer to the resource which shall be locked. If 0, the default resource is locked.
- Returns
- 0 if the resource is already locked or a valid save ticket otherwise.
- See also
- save()
Definition at line 488 of file addressbook.cpp.
◆ resourceLoadingError
|
protectedslot |
Definition at line 828 of file addressbook.cpp.
◆ resourceLoadingFinished
|
protectedslot |
Definition at line 812 of file addressbook.cpp.
◆ resourceManager()
|
protected |
Definition at line 798 of file addressbook.cpp.
◆ resources()
TQPtrList< Resource > AddressBook::resources | ( | ) |
Returns a list of all resources.
Definition at line 751 of file addressbook.cpp.
◆ resourceSavingError
|
protectedslot |
Definition at line 837 of file addressbook.cpp.
◆ resourceSavingFinished
|
protectedslot |
Definition at line 821 of file addressbook.cpp.
◆ save()
bool AddressBook::save | ( | Ticket * | ticket | ) |
Saves all addressees of one resource synchronously.
If the save is successfull the ticket is deleted.
- Parameters
-
ticket The ticket returned by requestSaveTicket().
- Returns
- Whether the saving was successfully.
Definition at line 373 of file addressbook.cpp.
◆ savingFinished
|
signal |
Emitted when the asynchronous saving of one resource has finished after calling asyncSave().
- Parameters
-
resource The resource which emitted this signal.
◆ setErrorHandler()
void AddressBook::setErrorHandler | ( | ErrorHandler * | errorHandler | ) |
Sets the ErrorHandler
, that is used by error() to provide GUI independent error messages.
- Parameters
-
errorHandler The error handler you want to use.
Definition at line 766 of file addressbook.cpp.
◆ setStandardResource()
|
protected |
Definition at line 788 of file addressbook.cpp.
◆ standardResource()
|
protected |
Definition at line 793 of file addressbook.cpp.
Friends And Related Function Documentation
◆ StdAddressBook
|
friend |
Definition at line 49 of file addressbook.h.
The documentation for this class was generated from the following files: