#include <folder.h>
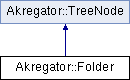
Public Slots | |
virtual void | slotDeleteExpiredArticles () |
virtual void | slotMarkAllArticlesAsRead () |
virtual void | slotChildChanged (TreeNode *node) |
virtual void | slotChildDestroyed (TreeNode *node) |
virtual void | slotAddToFetchQueue (FetchQueue *queue, bool intervalFetchesOnly=false) |
virtual TreeNode * | next () |
![]() | |
virtual void | slotDeleteExpiredArticles ()=0 |
virtual void | slotMarkAllArticlesAsRead ()=0 |
virtual void | slotAddToFetchQueue (FetchQueue *queue, bool intervalFetchesOnly=false)=0 |
Signals | |
void | signalChildAdded (TreeNode *) |
void | signalChildRemoved (Folder *, TreeNode *) |
![]() | |
void | signalDestroyed (TreeNode *) |
void | signalChanged (TreeNode *) |
void | signalArticlesAdded (TreeNode *node, const TQValueList< Article > &guids) |
void | signalArticlesUpdated (TreeNode *, const TQValueList< Article > &guids) |
void | signalArticlesRemoved (TreeNode *, const TQValueList< Article > &guids) |
Public Member Functions | |
Folder (const TQString &title=TQString()) | |
virtual bool | accept (TreeNodeVisitor *visitor) |
virtual TQValueList< Article > | articles (const TQString &tag=TQString()) |
virtual TQStringList | tags () const |
virtual int | unread () const |
virtual int | totalCount () const |
virtual bool | isGroup () const |
virtual TQDomElement | toOPML (TQDomElement parent, TQDomDocument document) const |
virtual TQValueList< TreeNode * > | children () const |
virtual void | insertChild (TreeNode *node, TreeNode *after) |
virtual void | prependChild (TreeNode *node) |
virtual void | appendChild (TreeNode *node) |
virtual void | removeChild (TreeNode *node) |
virtual TreeNode * | firstChild () |
virtual TreeNode * | lastChild () |
virtual bool | isOpen () const |
virtual void | setOpen (bool open) |
![]() | |
TreeNode () | |
virtual | ~TreeNode () |
virtual const TQString & | title () const |
virtual void | setTitle (const TQString &title) |
virtual TreeNode * | nextSibling () const |
virtual TreeNode * | prevSibling () const |
virtual Folder * | parent () const |
virtual void | setParent (Folder *parent) |
virtual void | setNotificationMode (bool doNotify, bool notifyOccurredChanges=true) |
virtual TreeNode * | next ()=0 |
virtual uint | id () const |
virtual void | setId (uint id) |
Static Public Member Functions | |
static Folder * | fromOPML (TQDomElement e) |
Protected Member Functions | |
virtual void | insertChild (uint index, TreeNode *node) |
virtual void | doArticleNotification () |
![]() | |
virtual void | nodeModified () |
virtual void | articlesModified () |
void | emitSignalDestroyed () |
Detailed Description
Constructor & Destructor Documentation
◆ Folder()
Akregator::Folder::Folder | ( | const TQString & | title = TQString() | ) |
Creates a new folder with a given title.
- Parameters
-
title The title of the feed group
Definition at line 71 of file folder.cpp.
Member Function Documentation
◆ appendChild()
|
virtual |
inserts node
as last child
- Parameters
-
node the tree node to insert
Definition at line 168 of file folder.cpp.
◆ articles()
|
virtual |
returns recursively concatenated articles of children
- Returns
- an article sequence containing articles of children
Implements Akregator::TreeNode.
Definition at line 108 of file folder.cpp.
◆ children()
|
virtual |
returns the (direct) children of this node.
- Returns
- a list of pointers to the child nodes
Definition at line 133 of file folder.cpp.
◆ doArticleNotification()
|
protectedvirtual |
reimplement this in subclasses to do the actual notification called by articlesModified
Reimplemented from Akregator::TreeNode.
Definition at line 305 of file folder.cpp.
◆ firstChild()
|
virtual |
returns the first child of the group, 0 if none exist
Definition at line 220 of file folder.cpp.
◆ fromOPML()
|
static |
creates a feed group parsed from a XML dom element.
Child nodes are not inserted or parsed.
- Parameters
-
e the element representing the feed group
- Returns
- a freshly created feed group
Definition at line 63 of file folder.cpp.
◆ insertChild() [1/2]
inserts node
as child after child node after
.
if after
is not a child of this group, node
will be inserted as first child
- Parameters
-
node the tree node to insert after the node after which node
will be inserted
Definition at line 138 of file folder.cpp.
◆ insertChild() [2/2]
|
protectedvirtual |
inserts node
as child on position index
- Parameters
-
index the position where to insert node the tree node to insert
Definition at line 148 of file folder.cpp.
◆ isGroup()
|
inlinevirtual |
Helps the rest of the app to decide if node should be handled as group or not.
Use only where necessary, use polymorphism where possible.
Implements Akregator::TreeNode.
◆ isOpen()
|
virtual |
returns whether the feed group is opened or not.
Use only in FolderItem.
Definition at line 230 of file folder.cpp.
◆ lastChild()
|
virtual |
returns the last child of the group, 0 if none exist
Definition at line 225 of file folder.cpp.
◆ next
|
virtualslot |
returns the next node in the tree.
Calling next() unless it returns 0 iterates through the tree in pre-order
Definition at line 327 of file folder.cpp.
◆ prependChild()
|
virtual |
inserts node
as first child
- Parameters
-
node the tree node to insert
Definition at line 185 of file folder.cpp.
◆ removeChild()
|
virtual |
remove node
from children.
Note that node
will not be deleted
- Parameters
-
node the child node to remove
Definition at line 202 of file folder.cpp.
◆ setOpen()
|
virtual |
open/close the feed group (display it as expanded/collapsed in the tree view).
Use only in FolderItem.
Definition at line 235 of file folder.cpp.
◆ signalChildAdded
|
signal |
emitted when a child was added
◆ signalChildRemoved
emitted when a child was removed
◆ slotAddToFetchQueue
|
virtualslot |
enqueues children recursively for fetching
- Parameters
-
queue a fetch queue internvalFetchesOnly
Definition at line 298 of file folder.cpp.
◆ slotChildChanged
|
virtualslot |
Called when a child was modified.
- Parameters
-
node the child that was changed
Definition at line 276 of file folder.cpp.
◆ slotChildDestroyed
|
virtualslot |
Called when a child was destroyed.
- Parameters
-
node the child that was destroyed
Definition at line 282 of file folder.cpp.
◆ slotDeleteExpiredArticles
|
virtualslot |
Delete expired articles recursively.
Definition at line 289 of file folder.cpp.
◆ slotMarkAllArticlesAsRead
|
virtualslot |
Mark articles of children recursively as read.
Definition at line 267 of file folder.cpp.
◆ tags()
|
virtual |
returns a list of all tags occurring in the subtree of this folder
Implements Akregator::TreeNode.
Definition at line 93 of file folder.cpp.
◆ toOPML()
|
virtual |
converts the feed group into OPML format for save and export and appends it to node parent
in document @document.
Children are processed and appended recursively.
- Parameters
-
parent The parent element document The DOM document
- Returns
- The newly created element representing this feed group
Implements Akregator::TreeNode.
Definition at line 118 of file folder.cpp.
◆ totalCount()
|
virtual |
returns the number of articles in all children
- Returns
- number of articles
Implements Akregator::TreeNode.
Definition at line 245 of file folder.cpp.
◆ unread()
|
virtual |
returns the number of unread articles in all children
- Returns
- number of unread articles
Implements Akregator::TreeNode.
Definition at line 240 of file folder.cpp.
The documentation for this class was generated from the following files: