#include <actionmanager.h>
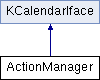
Public Slots | |
bool | addResource (const KURL &mUrl) |
bool | openURL (const KURL &url, bool merge=false) |
bool | saveURL () |
bool | saveAsURL (const KURL &kurl) |
bool | saveModifiedURL () |
void | exportHTML () |
void | exportHTML (HTMLExportSettings *) |
void | updateConfig () |
void | setDestinationPolicy () |
void | processIncidenceSelection (Incidence *incidence, const TQDate &date) |
void | keyBindings () |
void | readSettings () |
void | writeSettings () |
void | saveProperties (TDEConfig *) |
void | readProperties (TDEConfig *) |
void | loadParts () |
void | importCalendar (const KURL &url) |
Signals | |
void | actionNew (const KURL &url=KURL()) |
void | configChanged () |
void | closingDown () |
void | resourceAdded (ResourceCalendar *) |
Public Member Functions | |
ActionManager (KXMLGUIClient *client, CalendarView *widget, TQObject *parent, KOrg::MainWindow *mainWindow, bool isPart) | |
void | init () |
CalendarView * | view () const |
void | createCalendarLocal () |
void | createCalendarResources () |
void | saveCalendar () |
bool | saveResourceCalendar () |
void | loadResourceCalendar () |
KURL | url () const |
bool | openURL (const TQString &url) |
bool | mergeURL (const TQString &url) |
bool | saveAsURL (const TQString &url) |
void | closeURL () |
TQString | getCurrentURLasString () const |
virtual bool | deleteIncidence (const TQString &uid, bool force=false) |
bool | editIncidence (const TQString &uid) |
bool | editIncidence (const TQString &uid, const TQDate &date) |
bool | addIncidence (const TQString &ical) |
virtual ResourceRequestReply | resourceRequest (const TQValueList< TQPair< TQDateTime, TQDateTime > > &busy, const TQCString &resource, const TQString &vCalIn) |
void | openEventEditor (const TQString &) |
void | openEventEditor (const TQString &summary, const TQString &description, const TQString &attachment) |
void | openEventEditor (const TQString &summary, const TQString &description, const TQString &attachment, const TQStringList &attendees) |
void | openEventEditor (const TQString &summary, const TQString &description, const TQString &uri, const TQString &file, const TQStringList &attendees, const TQString &attachmentMimetype) |
void | openTodoEditor (const TQString &) |
void | openTodoEditor (const TQString &summary, const TQString &description, const TQString &attachment) |
void | openTodoEditor (const TQString &summary, const TQString &description, const TQString &attachment, const TQStringList &attendees) |
void | openTodoEditor (const TQString &summary, const TQString &description, const TQString &uri, const TQString &file, const TQStringList &attendees, const TQString &attachmentMimetype, bool isTask) |
void | openJournalEditor (const TQDate &date) |
void | openJournalEditor (const TQString &text, const TQDate &date) |
void | openJournalEditor (const TQString &text) |
void | showJournalView () |
void | showTodoView () |
void | showEventView () |
void | goDate (const TQDate &) |
void | goDate (const TQString &) |
void | showDate (const TQDate &date) |
TQString | localFileName () |
bool | queryClose () |
void | loadProfile (const TQString &path) |
void | saveToProfile (const TQString &path) const |
bool | handleCommandLine () |
Static Public Member Functions | |
static KOrg::MainWindow * | findInstance (const KURL &url) |
Protected Slots | |
void | file_new () |
void | file_open () |
void | file_open (const KURL &url) |
void | file_icalimport () |
void | file_merge () |
void | file_revert () |
void | file_archive () |
void | file_save () |
void | file_saveas () |
void | file_close () |
void | configureDateTime () |
void | showTip () |
void | showTipOnStart () |
void | downloadNewStuff () |
void | uploadNewStuff () |
void | toggleResourceButtons () |
void | toggleDateNavigator () |
void | toggleTodoView () |
void | toggleEventViewer () |
void | toggleResourceView () |
void | checkAutoSave () |
void | slotAutoArchivingSettingsModified () |
void | slotAutoArchive () |
void | configureDateTimeFinished (TDEProcess *) |
void | setTitle () |
void | updateUndoAction (const TQString &) |
void | updateRedoAction (const TQString &) |
void | slotPreviewDialogFinished (PreviewDialog *) |
Protected Member Functions | |
KURL | getSaveURL () |
void | showStatusMessageOpen (const KURL &url, bool merge) |
void | initCalendar (Calendar *cal) |
TQWidget * | dialogParent () |
Detailed Description
The ActionManager creates all the actions in KOrganizer.
This class is shared between the main application and the part so all common actions are in one location. It also provides DCOP interface[s].
Definition at line 73 of file actionmanager.h.
Member Function Documentation
◆ actionNew
|
signal |
Emitted when the "New" action is activated.
◆ addIncidence()
bool ActionManager::addIncidence | ( | const TQString & | ical | ) |
Add an incidence to the active calendar.
- Parameters
-
ical A calendar in iCalendar format containing the incidence.
Definition at line 1403 of file actionmanager.cpp.
◆ addResource
|
slot |
Add a new resource.
Definition at line 917 of file actionmanager.cpp.
◆ checkAutoSave
|
protectedslot |
called by the autoSaveTimer to automatically save the calendar
Definition at line 1238 of file actionmanager.cpp.
◆ closeURL()
void ActionManager::closeURL | ( | ) |
Close calendar file opened from URL.
Definition at line 974 of file actionmanager.cpp.
◆ closingDown
|
signal |
Emitted when the topwidget is closing down, so that any attached child windows can also close.
◆ configChanged
|
signal |
When change is made to options dialog, the topwidget will catch this and emit this signal which notifies all widgets which have registered for notification to update their settings.
◆ configureDateTime
|
protectedslot |
Open kcontrol module for configuring date and time formats.
Definition at line 1291 of file actionmanager.cpp.
◆ createCalendarLocal()
void ActionManager::createCalendarLocal | ( | ) |
Create Calendar object based on local file and set it on the view.
Definition at line 169 of file actionmanager.cpp.
◆ createCalendarResources()
void ActionManager::createCalendarResources | ( | ) |
Create Calendar object based on the resource framework and set it on the view.
Definition at line 178 of file actionmanager.cpp.
◆ deleteIncidence()
|
virtual |
Delete the incidence with the given unique id from current calendar.
- Parameters
-
uid UID of the incidence to delete. force If true, all recurrences and sub-todos (if applicable) will be deleted without prompting for confirmation.
Definition at line 1398 of file actionmanager.cpp.
◆ dialogParent()
|
protected |
Return widget used as parent for dialogs and message boxes.
Definition at line 2131 of file actionmanager.cpp.
◆ file_archive
|
protectedslot |
delete or archive old entries in your calendar for speed/space.
Definition at line 807 of file actionmanager.cpp.
◆ file_close
|
protectedslot |
close a file, prompt for save if changes made.
Definition at line 845 of file actionmanager.cpp.
◆ file_icalimport
|
protectedslot |
import a calendar from another program like ical.
Definition at line 740 of file actionmanager.cpp.
◆ file_merge
|
protectedslot |
open a calendar and add the contents to the current calendar.
Definition at line 798 of file actionmanager.cpp.
◆ file_new
|
protectedslot |
open new window
Definition at line 704 of file actionmanager.cpp.
◆ file_open [1/2]
|
protectedslot |
open a file, load it into the calendar.
Definition at line 709 of file actionmanager.cpp.
◆ file_open [2/2]
|
protectedslot |
open a file from the list of recent files.
Also called from file_open() after the URL is obtained from the user.
Definition at line 719 of file actionmanager.cpp.
◆ file_revert
|
protectedslot |
revert to saved
Definition at line 812 of file actionmanager.cpp.
◆ file_save
|
protectedslot |
save a file with the current fileName.
Definition at line 826 of file actionmanager.cpp.
◆ file_saveas
|
protectedslot |
save a file under a (possibly) different filename.
Definition at line 817 of file actionmanager.cpp.
◆ findInstance()
|
static |
Is there a instance with this URL?
Definition at line 1316 of file actionmanager.cpp.
◆ getCurrentURLasString()
TQString ActionManager::getCurrentURLasString | ( | ) | const |
Get current URL as TQString.
Definition at line 1383 of file actionmanager.cpp.
◆ getSaveURL()
|
protected |
◆ init()
void ActionManager::init | ( | ) |
Peform initialization that requires this* to be full constructed.
Definition at line 120 of file actionmanager.cpp.
◆ loadResourceCalendar()
void ActionManager::loadResourceCalendar | ( | ) |
Load the resource based calendar.
Definition at line 1994 of file actionmanager.cpp.
◆ mergeURL()
bool ActionManager::mergeURL | ( | const TQString & | url | ) |
Open calendar file from URL.
Definition at line 1373 of file actionmanager.cpp.
◆ openURL [1/2]
|
slot |
Open calendar file from URL.
Merge into current calendar, if merge is true.
Definition at line 857 of file actionmanager.cpp.
◆ openURL() [2/2]
bool ActionManager::openURL | ( | const TQString & | url | ) |
Open calendar file from URL.
Definition at line 1368 of file actionmanager.cpp.
◆ readSettings
|
slot |
Using the TDEConfig associated with the kapp variable, read in the settings from the config file.
Definition at line 656 of file actionmanager.cpp.
◆ resourceAdded
|
signal |
Indicates that a new resource was added.
◆ saveAsURL [1/2]
|
slot |
Save calendar file to URL.
Definition at line 1104 of file actionmanager.cpp.
◆ saveAsURL() [2/2]
bool ActionManager::saveAsURL | ( | const TQString & | url | ) |
Save calendar file to URL.
Definition at line 1378 of file actionmanager.cpp.
◆ saveCalendar()
void ActionManager::saveCalendar | ( | ) |
Save calendar to disk.
Definition at line 1957 of file actionmanager.cpp.
◆ saveModifiedURL
|
slot |
Save calendar if it is modified by the user.
Ask user what to do.
Definition at line 1150 of file actionmanager.cpp.
◆ saveResourceCalendar()
bool ActionManager::saveResourceCalendar | ( | ) |
Save the resource based calendar.
Return false if an error occured and the user decidec to not ignore the error. Otherwise it returns true.
Definition at line 1974 of file actionmanager.cpp.
◆ saveURL
|
slot |
Save calendar file to URL of current calendar.
Definition at line 981 of file actionmanager.cpp.
◆ showTip
|
protectedslot |
Show tip of the day.
Definition at line 1306 of file actionmanager.cpp.
◆ showTipOnStart
|
protectedslot |
Show tip of the day.
Definition at line 1311 of file actionmanager.cpp.
◆ slotAutoArchive
|
protectedslot |
called by the auto archive timer to automatically delete/archive events
Definition at line 2043 of file actionmanager.cpp.
◆ slotAutoArchivingSettingsModified
|
protectedslot |
connected to CalendarView's signal which comes from the ArchiveDialog
Definition at line 2035 of file actionmanager.cpp.
◆ updateConfig
|
slot |
Options dialog made a changed to the configuration.
we catch this and notify all widgets which need to update their configuration.
Definition at line 1255 of file actionmanager.cpp.
◆ url()
|
inline |
Get current URL.
Definition at line 133 of file actionmanager.h.
◆ writeSettings
|
slot |
Write current state to config file.
Definition at line 666 of file actionmanager.cpp.
The documentation for this class was generated from the following files: