#include <calendar.h>
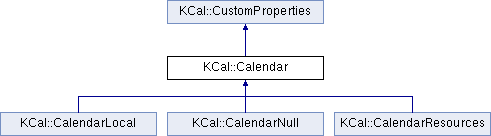
Classes | |
class | Observer |
Signals | |
void | calendarChanged () |
void | calendarSaved () |
void | calendarLoaded () |
void | batchAddingBegins () |
void | batchAddingEnds () |
Public Member Functions | |
Calendar (const TQString &timeZoneId) | |
virtual | ~Calendar () |
void | setProductId (const TQString &productId) |
TQString | productId () |
void | clearException () |
ErrorFormat * | exception () const |
void | setOwner (const Person &owner) |
const Person & | getOwner () const |
void | setTimeZoneId (const TQString &timeZoneId) |
virtual void | setTimeZoneIdViewOnly (const TQString &timeZoneId)=0 |
TQString | timeZoneId () const |
void | setLocalTime () |
bool | isLocalTime () const |
void | setModified (bool modified) |
bool | isModified () const |
virtual void | close ()=0 |
virtual void | closeEvents ()=0 |
virtual void | closeTodos ()=0 |
virtual void | closeJournals ()=0 |
virtual void | save ()=0 |
virtual bool | reload (const TQString &tz)=0 |
virtual bool | isSaving () |
TQStringList | categories () |
virtual bool | addIncidence (Incidence *incidence) |
virtual bool | deleteIncidence (Incidence *incidence) |
virtual Incidence::List | incidences () |
virtual Incidence::List | incidences (const TQDate &date) |
virtual Incidence::List | rawIncidences () |
Incidence * | incidence (const TQString &uid) |
Incidence * | incidenceFromSchedulingID (const TQString &sid) |
Incidence::List | incidencesFromSchedulingID (const TQString &UID) |
virtual bool | beginChange (Incidence *incidence) |
virtual bool | endChange (Incidence *incidence) |
Incidence * | dissociateOccurrence (Incidence *incidence, TQDate date, bool single=true) |
virtual bool | addEvent (Event *event)=0 |
virtual bool | deleteEvent (Event *event)=0 |
virtual Event::List | events (EventSortField sortField=EventSortUnsorted, SortDirection sortDirection=SortDirectionAscending) |
Event::List | events (const TQDateTime &qdt) |
Event::List | events (const TQDate &start, const TQDate &end, bool inclusive=false) |
Event::List | events (const TQDate &date, EventSortField sortField=EventSortUnsorted, SortDirection sortDirection=SortDirectionAscending) |
virtual Event::List | rawEvents (EventSortField sortField=EventSortUnsorted, SortDirection sortDirection=SortDirectionAscending)=0 |
virtual Event::List | rawEventsForDate (const TQDateTime &qdt)=0 |
virtual Event::List | rawEvents (const TQDate &start, const TQDate &end, bool inclusive=false)=0 |
virtual Event::List | rawEventsForDate (const TQDate &date, EventSortField sortField=EventSortUnsorted, SortDirection sortDirection=SortDirectionAscending)=0 |
virtual Event * | event (const TQString &uid)=0 |
virtual bool | addTodo (Todo *todo)=0 |
virtual bool | deleteTodo (Todo *todo)=0 |
virtual Todo::List | todos (TodoSortField sortField=TodoSortUnsorted, SortDirection sortDirection=SortDirectionAscending) |
virtual Todo::List | todos (const TQDate &date) |
virtual Todo::List | rawTodos (TodoSortField sortField=TodoSortUnsorted, SortDirection sortDirection=SortDirectionAscending)=0 |
virtual Todo::List | rawTodosForDate (const TQDate &date)=0 |
virtual Todo * | todo (const TQString &uid)=0 |
virtual bool | addJournal (Journal *journal)=0 |
virtual bool | deleteJournal (Journal *journal)=0 |
virtual Journal::List | journals (JournalSortField sortField=JournalSortUnsorted, SortDirection sortDirection=SortDirectionAscending) |
virtual Journal::List | journals (const TQDate &date) |
virtual Journal::List | rawJournals (JournalSortField sortField=JournalSortUnsorted, SortDirection sortDirection=SortDirectionAscending)=0 |
virtual Journal::List | rawJournalsForDate (const TQDate &date)=0 |
virtual Journal * | journal (const TQString &uid)=0 |
void | beginBatchAdding () |
void | endBatchAdding () |
virtual void | setupRelations (Incidence *incidence) |
virtual void | removeRelations (Incidence *incidence) |
void | setFilter (CalFilter *filter) |
CalFilter * | filter () |
virtual Alarm::List | alarms (const TQDateTime &from, const TQDateTime &to)=0 |
void | registerObserver (Observer *observer) |
void | unregisterObserver (Observer *observer) |
![]() | |
CustomProperties () | |
CustomProperties (const CustomProperties &) | |
bool | operator== (const CustomProperties &) const |
void | setCustomProperty (const TQCString &app, const TQCString &key, const TQString &value) |
void | removeCustomProperty (const TQCString &app, const TQCString &key) |
TQString | customProperty (const TQCString &app, const TQCString &key) const |
void | setNonKDECustomProperty (const TQCString &name, const TQString &value) |
void | removeNonKDECustomProperty (const TQCString &name) |
TQString | nonKDECustomProperty (const TQCString &name) const |
void | setCustomProperties (const TQMap< TQCString, TQString > &properties) |
TQMap< TQCString, TQString > | customProperties () const |
Static Public Member Functions | |
static Incidence::List | mergeIncidenceList (const Event::List &events, const Todo::List &todos, const Journal::List &journals) |
static Event::List | sortEvents (Event::List *eventList, EventSortField sortField, SortDirection sortDirection) |
static Event::List | sortEventsForDate (Event::List *eventList, const TQDate &date, EventSortField sortField, SortDirection sortDirection) |
static Todo::List | sortTodos (Todo::List *todoList, TodoSortField sortField, SortDirection sortDirection) |
static Journal::List | sortJournals (Journal::List *journalList, JournalSortField sortField, SortDirection sortDirection) |
Protected Member Functions | |
void | setException (ErrorFormat *e) |
void | incidenceUpdated (IncidenceBase *incidenceBase) |
virtual void | doSetTimeZoneId (const TQString &) |
void | notifyIncidenceAdded (Incidence *incidence) |
void | notifyIncidenceChanged (Incidence *incidence) |
void | notifyIncidenceDeleted (Incidence *incidence) |
virtual void | customPropertyUpdated () |
void | setObserversEnabled (bool enabled) |
virtual void | customPropertyUpdated () |
Detailed Description
This is the main "calendar" object class.
It holds information like Incidences(Events, To-dos, Journals), user information, etc. etc.
This is an abstract base class defining the interface to a calendar. It is implemented by subclasses like CalendarLocal, which use different methods to store and access the data.
Ownership of Incidences:
Incidence ownership is handled by the following policy: As soon as an Incidence (or any other subclass of IncidenceBase) object is added to the Calendar by an add...() method it is owned by the Calendar object. The Calendar takes care of deleting it. All Incidences returned by the query functions are returned as pointers so that changes to the returned Incidences are immediately visible in the Calendar. Do Not delete any Incidence object you get from Calendar.
Time Zone Handling:
- Incidence Storing:
- By default, (when LocalTime is unset) Incidence dates will have the "UTC" time zone when stored into a calendar file.
- To store Incidence dates without a time zone (i.e, "floating time zone") LocalTime must be set using the setLocalTime() method.
- Incidence Viewing:
- By default, Incidence dates will have the "UTC" time zone when read from a calendar.
- To view Incidence dates using another time zone TimeZoneId must be set using the setTimeZoneId() method, or the TimeZoneId can be passed to the Calendar constructor.
- It is permitted to switch viewing time zones using setTimeZoneId() as desired after the Calendar object has been constructed.
- Note that:
- The Calendar class doesn't do anything with TimeZoneId: it simply saves it for later use by the ICalFormat class.
- The ICalFormat class takes TimeZoneId and applies it to loaded Incidences before returning them in ICalFormat::load().
- Each Incidence can have its own time zone (or have a floating time zone).
- Once an Incidence is loaded it is adjusted to use the viewing time zone, TimeZoneId.
- Depending on the LocalTime setting, all loaded Incidences are stored either in UTC or without a time zone (floating time zone).
Definition at line 169 of file calendar.h.
Constructor & Destructor Documentation
◆ Calendar()
Calendar::Calendar | ( | const TQString & | timeZoneId | ) |
Construct Calendar object using a Time Zone.
- Parameters
-
timeZoneId is a string containing a Time Zone ID, which is assumed to be valid. The Time Zone Id is used to set the time zone for viewing Incidence dates.
On some systems, /usr/share/zoneinfo/zone.tab may be available for reference.
Example: "Europe/Berlin"
- Warning
- Do Not pass an empty timeZoneId string as this may cause unintended consequences when storing Incidences into the Calendar.
Definition at line 43 of file calendar.cpp.
◆ ~Calendar()
|
virtual |
Destructor.
Definition at line 68 of file calendar.cpp.
Member Function Documentation
◆ addEvent()
|
pure virtual |
Insert an Event into the Calendar.
- Parameters
-
event is a pointer to the Event to insert.
- Returns
- true if the Event was successfully inserted; false otherwise.
Implemented in KCal::CalendarNull, KCal::CalendarLocal, and KCal::CalendarResources.
◆ addIncidence()
|
virtual |
Insert an Incidence into the Calendar.
- Parameters
-
incidence is a pointer to the Incidence to insert.
- Returns
- true if the Incidence was successfully inserted; false otherwise.
Reimplemented in KCal::CalendarResources.
Definition at line 466 of file calendar.cpp.
◆ addJournal()
|
pure virtual |
Insert a Journal into the Calendar.
- Parameters
-
journal is a pointer to the Journal to insert.
- Returns
- true if the Journal was successfully inserted; false otherwise.
Implemented in KCal::CalendarLocal, KCal::CalendarNull, and KCal::CalendarResources.
◆ addTodo()
|
pure virtual |
Insert a Todo into the Calendar.
- Parameters
-
todo is a pointer to the Todo to insert.
- Returns
- true if the Todo was successfully inserted; false otherwise.
Implemented in KCal::CalendarNull, KCal::CalendarLocal, and KCal::CalendarResources.
◆ alarms()
|
pure virtual |
Return a list of Alarms within a time range for this Calendar.
- Parameters
-
from is the starting timestamp. to is the ending timestamp.
- Returns
- the list of Alarms for the for the specified time range.
Implemented in KCal::CalendarNull, KCal::CalendarLocal, and KCal::CalendarResources.
◆ batchAddingBegins
|
signal |
- See also
- beginBatchAdding()
- Since
- 4.4
◆ batchAddingEnds
|
signal |
- See also
- endBatchAdding()
- Since
- 4.4
◆ beginBatchAdding()
void Calendar::beginBatchAdding | ( | ) |
Emits the beginBatchAdding() signal.
This should be called before adding a batch of incidences with addIncidence( Incidence *), addTodo( Todo *), addEvent( Event *) or addJournal( Journal *). Some Calendars are connected to this signal, e.g: CalendarResources uses it to know a series of incidenceAdds are related so the user isn't prompted multiple times which resource to save the incidence to
- Since
- 4.4
Definition at line 144 of file calendar.cpp.
◆ beginChange()
|
virtual |
Flag that a change to a Calendar Incidence is starting.
- Parameters
-
incidence is a pointer to the Incidence that will be changing.
Reimplemented in KCal::CalendarResources.
Definition at line 1049 of file calendar.cpp.
◆ calendarChanged
|
signal |
Signal that the Calendar has been modified.
◆ calendarLoaded
|
signal |
Signal that the Calendar has been loaded into memory.
◆ calendarSaved
|
signal |
Signal that the Calendar has been saved.
◆ categories()
TQStringList Calendar::categories | ( | ) |
Return a list of all categories used by Incidences in this Calendar.
- Returns
- a TQStringList containing all the categories.
Definition at line 154 of file calendar.cpp.
◆ clearException()
void Calendar::clearException | ( | ) |
Clears the exception status.
Definition at line 74 of file calendar.cpp.
◆ close()
|
pure virtual |
Clears out the current Calendar, freeing all used memory etc.
Implemented in KCal::CalendarLocal, KCal::CalendarNull, and KCal::CalendarResources.
◆ closeEvents()
|
pure virtual |
Clears out the current Calendar, freeing all used memory etc.
Implemented in KCal::CalendarLocal, KCal::CalendarNull, and KCal::CalendarResources.
◆ closeJournals()
|
pure virtual |
Clears out the current Calendar, freeing all used memory etc.
Implemented in KCal::CalendarLocal, KCal::CalendarNull, and KCal::CalendarResources.
◆ closeTodos()
|
pure virtual |
Clears out the current Calendar, freeing all used memory etc.
Implemented in KCal::CalendarLocal, KCal::CalendarNull, and KCal::CalendarResources.
◆ customPropertyUpdated()
|
protectedvirtual |
CustomProperties::customPropertyUpdated()
Reimplemented from KCal::CustomProperties.
Definition at line 1013 of file calendar.cpp.
◆ deleteEvent()
|
pure virtual |
Remove an Event from the Calendar.
- Parameters
-
event is a pointer to the Event to remove.
- Returns
- true if the Event was successfully remove; false otherwise.
Implemented in KCal::CalendarNull, KCal::CalendarLocal, and KCal::CalendarResources.
◆ deleteIncidence()
|
virtual |
◆ deleteJournal()
|
pure virtual |
Remove a Journal from the Calendar.
- Parameters
-
journal is a pointer to the Journal to remove.
- Returns
- true if the Journal was successfully removed; false otherwise.
Implemented in KCal::CalendarLocal, KCal::CalendarNull, and KCal::CalendarResources.
◆ deleteTodo()
|
pure virtual |
Remove a Todo from the Calendar.
- Parameters
-
todo is a pointer to the Todo to remove.
- Returns
- true if the Todo was successfully removed; false otherwise.
Implemented in KCal::CalendarLocal, KCal::CalendarNull, and KCal::CalendarResources.
◆ dissociateOccurrence()
Incidence * Calendar::dissociateOccurrence | ( | Incidence * | incidence, |
TQDate | date, | ||
bool | single = true |
||
) |
Dissociate an Incidence from a recurring Incidence.
Dissociate a single occurrence or all future occurrences from a recurring sequence.
By default, only one single Incidence for the specified date will be dissociated and returned. If single is false, then the recurrence will be split at date, the old Incidence will have its recurrence ending at date and the new Incidence will have all recurrences past the date.
- Parameters
-
incidence is a pointer to a recurring Incidence. date is the TQDate within the recurring Incidence on which the dissociation will be performed. single is a flag meaning that a new Incidence should be created from the recurring Incidences after date.
- Returns
- a pointer to a new recurring Incidence if single is false.
The new incidence is returned, but not automatically inserted into the calendar, which is left to the calling application
Definition at line 508 of file calendar.cpp.
◆ doSetTimeZoneId()
|
inlineprotectedvirtual |
Let Calendar subclasses set the Time Zone ID.
First parameter is a string containing a Time Zone ID, which is assumed to be valid. On some systems, /usr/share/zoneinfo/zone.tab may be available for reference.
Example: "Europe/Berlin"
- Warning
- Do Not pass an empty timeZoneId string as this may cause unintended consequences when storing Incidences into the Calendar.
Reimplemented in KCal::CalendarResources.
Definition at line 974 of file calendar.h.
◆ endBatchAdding()
void Calendar::endBatchAdding | ( | ) |
Emits the endBatchAdding() signal.
Used with beginBatchAdding(). Should be called after adding all incidences.
- Since
- 4.4
Definition at line 149 of file calendar.cpp.
◆ endChange()
|
virtual |
Flag that a change to a Calendar Incidence has completed.
- Parameters
-
incidence is a pointer to the Incidence that was changed.
Reimplemented in KCal::CalendarResources.
Definition at line 1054 of file calendar.cpp.
◆ event()
|
pure virtual |
Returns the Event associated with the given unique identifier.
- Parameters
-
uid is a unique identifier string.
Implemented in KCal::CalendarNull, KCal::CalendarLocal, and KCal::CalendarResources.
◆ events() [1/4]
Event::List Calendar::events | ( | const TQDate & | date, |
EventSortField | sortField = EventSortUnsorted , |
||
SortDirection | sortDirection = SortDirectionAscending |
||
) |
Return a sorted, filtered list of all Events which occur on the given date.
The Events are sorted according to sortField and sortDirection.
- Parameters
-
date request filtered Event list for this TQDate only. sortField specifies the EventSortField. sortDirection specifies the SortDirection.
- Returns
- the list of sorted, filtered Events occurring on date.
Definition at line 434 of file calendar.cpp.
◆ events() [2/4]
Event::List Calendar::events | ( | const TQDate & | start, |
const TQDate & | end, | ||
bool | inclusive = false |
||
) |
Return a filtered list of all Events occurring within a date range.
- Parameters
-
start is the starting date. end is the ending date. inclusive if true only Events which are completely included within the date range are returned.
- Returns
- the list of filtered Events occurring within the specified date range.
Definition at line 450 of file calendar.cpp.
◆ events() [3/4]
Event::List Calendar::events | ( | const TQDateTime & | qdt | ) |
Return a filtered list of all Events which occur on the given timestamp.
- Parameters
-
qdt request filtered Event list for this TQDateTime only.
- Returns
- the list of filtered Events occurring on the specified timestamp.
Definition at line 443 of file calendar.cpp.
◆ events() [4/4]
|
virtual |
Return a sorted, filtered list of all Events for this Calendar.
- Parameters
-
sortField specifies the EventSortField. sortDirection specifies the SortDirection.
- Returns
- the list of all filtered Events sorted as specified.
Definition at line 458 of file calendar.cpp.
◆ exception()
ErrorFormat * Calendar::exception | ( | ) | const |
Returns an exception, if there is any, containing information about the last error that occurred.
Definition at line 80 of file calendar.cpp.
◆ filter()
CalFilter * Calendar::filter | ( | ) |
◆ getOwner()
const Person & Calendar::getOwner | ( | ) | const |
Get the owner of the Calendar.
- Returns
- the owner Person object.
Definition at line 91 of file calendar.cpp.
◆ incidence()
Incidence * Calendar::incidence | ( | const TQString & | uid | ) |
Returns the Incidence associated with the given unique identifier.
- Parameters
-
uid is a unique identifier string.
Definition at line 576 of file calendar.cpp.
◆ incidenceFromSchedulingID()
Incidence * Calendar::incidenceFromSchedulingID | ( | const TQString & | sid | ) |
Returns the Incidence associated with the given scheduling identifier.
- Parameters
-
sid is a unique scheduling identifier string.
Definition at line 599 of file calendar.cpp.
◆ incidences() [1/2]
|
virtual |
Return a filtered list of all Incidences for this Calendar.
- Returns
- the list of all filtered Incidences.
Definition at line 178 of file calendar.cpp.
◆ incidences() [2/2]
|
virtual |
Return a filtered list of all Incidences which occur on the given date.
- Parameters
-
date request filtered Incidence list for this TQDate only.
- Returns
- the list of filtered Incidences occurring on the specified date.
Definition at line 173 of file calendar.cpp.
◆ incidencesFromSchedulingID()
Incidence::List Calendar::incidencesFromSchedulingID | ( | const TQString & | UID | ) |
Searches all events and todos for (an incidence with this scheduling ID.
Returns a list of matching results.
Definition at line 588 of file calendar.cpp.
◆ incidenceUpdated()
|
protected |
The Observer interface.
So far not implemented.
- Parameters
-
incidenceBase is a pointer an IncidenceBase object.
Definition at line 963 of file calendar.cpp.
◆ isLocalTime()
bool Calendar::isLocalTime | ( | ) | const |
Determine if Calendar Incidences are to be written without a time zone.
- Returns
- true if the Calendar is set to write Incidences withoout a time zone; false otherwise.
Definition at line 125 of file calendar.cpp.
◆ isModified()
|
inline |
Determine the Calendar's modification status.
- Returns
- true if the Calendar has been modified since open or last save.
Definition at line 294 of file calendar.h.
◆ isSaving()
|
inlinevirtual |
Determine if the Calendar is currently being saved.
- Returns
- true if the Calendar is currently being saved; false otherwise.
Reimplemented in KCal::CalendarResources.
Definition at line 333 of file calendar.h.
◆ journal()
|
pure virtual |
Returns the Journal associated with the given unique identifier.
- Parameters
-
uid is a unique identifier string.
Implemented in KCal::CalendarNull, KCal::CalendarLocal, and KCal::CalendarResources.
◆ journals() [1/2]
|
virtual |
Return a filtered list of all Journals for on the specifed date.
- Parameters
-
date request filtered Journals for this TQDate only.
- Returns
- the list of filtered Journals for the specified date.
Definition at line 831 of file calendar.cpp.
◆ journals() [2/2]
|
virtual |
Return a sorted, filtered list of all Journals for this Calendar.
- Parameters
-
sortField specifies the JournalSortField. sortDirection specifies the SortDirection.
- Returns
- the list of all filtered Journals sorted as specified.
Definition at line 823 of file calendar.cpp.
◆ mergeIncidenceList()
|
static |
Create a merged list of Events, Todos, and Journals.
- Parameters
-
events is an Event list to merge. todos is a Todo list to merge. journals is a Journal list to merge.
- Returns
- a list of merged Incidences.
Definition at line 1028 of file calendar.cpp.
◆ notifyIncidenceAdded()
|
protected |
Let Calendar subclasses notify that they inserted an Incidence.
- Parameters
-
incidence is a pointer to the Incidence object that was inserted.
Definition at line 977 of file calendar.cpp.
◆ notifyIncidenceChanged()
|
protected |
Let Calendar subclasses notify that they modified an Incidence.
- Parameters
-
incidence is a pointer to the Incidence object that was modified.
Definition at line 989 of file calendar.cpp.
◆ notifyIncidenceDeleted()
|
protected |
Let Calendar subclasses notify that they removed an Incidence.
- Parameters
-
incidence is a pointer to the Incidence object that was removed.
Definition at line 1001 of file calendar.cpp.
◆ productId()
TQString Calendar::productId | ( | ) |
Get the Calendar's Product ID.
- Returns
- the string containing the Product ID
Definition at line 1023 of file calendar.cpp.
◆ rawEvents() [1/2]
|
pure virtual |
Return an unfiltered list of all Events occurring within a date range.
- Parameters
-
start is the starting date. end is the ending date. inclusive if true only Events which are completely included within the date range are returned.
- Returns
- the list of unfiltered Events occurring within the specified date range.
Implemented in KCal::CalendarNull, KCal::CalendarLocal, and KCal::CalendarResources.
◆ rawEvents() [2/2]
|
pure virtual |
Return a sorted, unfiltered list of all Events for this Calendar.
- Parameters
-
sortField specifies the EventSortField. sortDirection specifies the SortDirection.
- Returns
- the list of all unfiltered Events sorted as specified.
Implemented in KCal::CalendarLocal, KCal::CalendarResources, and KCal::CalendarNull.
◆ rawEventsForDate() [1/2]
|
pure virtual |
Return a sorted, unfiltered list of all Events which occur on the given date.
The Events are sorted according to sortField and sortDirection.
- Parameters
-
date request unfiltered Event list for this TQDate only. sortField specifies the EventSortField. sortDirection specifies the SortDirection.
- Returns
- the list of sorted, unfiltered Events occurring on date.
Implemented in KCal::CalendarNull, KCal::CalendarLocal, and KCal::CalendarResources.
◆ rawEventsForDate() [2/2]
|
pure virtual |
Return an unfiltered list of all Events which occur on the given timestamp.
- Parameters
-
qdt request unfiltered Event list for this TQDateTime only.
- Returns
- the list of unfiltered Events occurring on the specified timestamp.
Implemented in KCal::CalendarNull, KCal::CalendarLocal, and KCal::CalendarResources.
◆ rawIncidences()
|
virtual |
Return an unfiltered list of all Incidences for this Calendar.
- Returns
- the list of all unfiltered Incidences.
Definition at line 183 of file calendar.cpp.
◆ rawJournals()
|
pure virtual |
Return a sorted, unfiltered list of all Journals for this Calendar.
- Parameters
-
sortField specifies the JournalSortField. sortDirection specifies the SortDirection.
- Returns
- the list of all unfiltered Journals sorted as specified.
Implemented in KCal::CalendarLocal, KCal::CalendarResources, and KCal::CalendarNull.
◆ rawJournalsForDate()
|
pure virtual |
Return an unfiltered list of all Journals for on the specifed date.
- Parameters
-
date request unfiltered Journals for this TQDate only.
- Returns
- the list of unfiltered Journals for the specified date.
Implemented in KCal::CalendarNull, KCal::CalendarLocal, and KCal::CalendarResources.
◆ rawTodos()
|
pure virtual |
Return a sorted, unfiltered list of all Todos for this Calendar.
- Parameters
-
sortField specifies the TodoSortField. sortDirection specifies the SortDirection.
- Returns
- the list of all unfiltered Todos sorted as specified.
Implemented in KCal::CalendarLocal, KCal::CalendarResources, and KCal::CalendarNull.
◆ rawTodosForDate()
|
pure virtual |
Return an unfiltered list of all Todos which due on the specified date.
- Parameters
-
date request unfiltered Todos due on this TQDate.
- Returns
- the list of unfiltered Todos due on the specified date.
Implemented in KCal::CalendarNull, KCal::CalendarLocal, and KCal::CalendarResources.
◆ registerObserver()
void Calendar::registerObserver | ( | Observer * | observer | ) |
◆ reload()
|
pure virtual |
Load the calendar contents from storage.
This requires the calendar to have been loaded once before, in other words initialized.
- tz The timezone to use for loading.
Implemented in KCal::CalendarNull, KCal::CalendarLocal, and KCal::CalendarResources.
◆ removeRelations()
|
virtual |
Remove all Relations from an Incidence.
- Parameters
-
incidence is a pointer to the Incidence to have a Relation removed.
Definition at line 874 of file calendar.cpp.
◆ save()
|
pure virtual |
Sync changes in memory to persistant storage.
Implemented in KCal::CalendarLocal, KCal::CalendarNull, and KCal::CalendarResources.
◆ setException()
|
protected |
Sets information about the last error occurred.
The previous exception is freed.
Definition at line 85 of file calendar.cpp.
◆ setFilter()
void Calendar::setFilter | ( | CalFilter * | filter | ) |
Set the Calendar filter.
Definition at line 130 of file calendar.cpp.
◆ setLocalTime()
void Calendar::setLocalTime | ( | ) |
Set to store calendar Incidences without a time zone.
Definition at line 117 of file calendar.cpp.
◆ setModified()
void Calendar::setModified | ( | bool | modified | ) |
Set if the Calendar had been modified.
- Parameters
-
modified is true if the Calendar has been modified since open or last save.
Definition at line 950 of file calendar.cpp.
◆ setObserversEnabled()
|
protected |
◆ setOwner()
void Calendar::setOwner | ( | const Person & | owner | ) |
Set the owner of the Calendar.
- Parameters
-
owner is a Person object.
Definition at line 96 of file calendar.cpp.
◆ setProductId()
void Calendar::setProductId | ( | const TQString & | productId | ) |
Set the Calendar Product ID.
- Parameters
-
productId is a TQString containing the Product ID.
Definition at line 1018 of file calendar.cpp.
◆ setTimeZoneId()
void Calendar::setTimeZoneId | ( | const TQString & | timeZoneId | ) |
Set the Time Zone Id for the Calendar.
- Parameters
-
timeZoneId is a string containing a Time Zone ID, which is assumed to be valid. The Time Zone Id is used to set the time zone for viewing Incidence dates.
On some systems, /usr/share/zoneinfo/zone.tab may be available for reference.
Example: "Europe/Berlin"
- Warning
- Do Not pass an empty timeZoneId string as this may cause unintended consequences when storing Incidences into the Calendar.
Definition at line 103 of file calendar.cpp.
◆ setTimeZoneIdViewOnly()
|
pure virtual |
Set the timezone used for viewing the incidences in this calendar.
In case it differs from the current timezone, shift the events such that they retain their absolute time (in UTC). setTimeZoneId
Implemented in KCal::CalendarNull, KCal::CalendarLocal, and KCal::CalendarResources.
◆ setupRelations()
|
virtual |
Setup Relations for an Incidence.
- Parameters
-
incidence is a pointer to the Incidence to have a Relation setup.
Definition at line 840 of file calendar.cpp.
◆ sortEvents()
|
static |
Sort a list of Events.
- Parameters
-
eventList is a pointer to a list of Events. sortField specifies the EventSortField. sortDirection specifies the SortDirection.
- Returns
- a list of Events sorted as specified.
Definition at line 188 of file calendar.cpp.
◆ sortEventsForDate()
|
static |
Sort a list of Events that occur on a specified date.
- Parameters
-
eventList is a pointer to a list of Events occurring on date
.date is the date. sortField specifies the EventSortField. sortDirection specifies the SortDirection.
- Returns
- a list of Events sorted as specified.
Definition at line 291 of file calendar.cpp.
◆ sortJournals()
|
static |
Sort a list of Journals.
- Parameters
-
journalList is a pointer to a list of Journals. sortField specifies the JournalSortField. sortDirection specifies the SortDirection.
- Returns
- a list of Journals sorted as specified.
Definition at line 770 of file calendar.cpp.
◆ sortTodos()
|
static |
Sort a list of Todos.
- Parameters
-
todoList is a pointer to a list of Todos. sortField specifies the TodoSortField. sortDirection specifies the SortDirection.
- Returns
- a list of Todos sorted as specified.
Definition at line 611 of file calendar.cpp.
◆ timeZoneId()
TQString Calendar::timeZoneId | ( | ) | const |
Get the Time Zone ID for the Calendar.
- Returns
- the string containg the Time Zone ID.
Definition at line 112 of file calendar.cpp.
◆ todo()
|
pure virtual |
Returns the Todo associated with the given unique identifier.
- Parameters
-
uid is a unique identifier string.
Implemented in KCal::CalendarNull, KCal::CalendarLocal, and KCal::CalendarResources.
◆ todos() [1/2]
|
virtual |
Return a filtered list of all Todos which are due on the specified date.
- Parameters
-
date request filtered Todos due on this TQDate.
- Returns
- the list of filtered Todos due on the specified date.
Definition at line 763 of file calendar.cpp.
◆ todos() [2/2]
|
virtual |
Return a sorted, filtered list of all Todos for this Calendar.
- Parameters
-
sortField specifies the TodoSortField. sortDirection specifies the SortDirection.
- Returns
- the list of all filtered Todos sorted as specified.
Definition at line 755 of file calendar.cpp.
◆ unregisterObserver()
void Calendar::unregisterObserver | ( | Observer * | observer | ) |
The documentation for this class was generated from the following files: