#include <incidence.h>
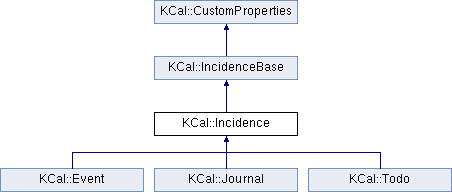
Classes | |
class | AddSubResourceVisitor |
class | AddVisitor |
class | DeleteVisitor |
Public Types | |
enum | Status { StatusNone , StatusTentative , StatusConfirmed , StatusCompleted , StatusNeedsAction , StatusCanceled , StatusInProcess , StatusDraft , StatusFinal , StatusX } |
enum | { SecrecyPublic = 0 , SecrecyPrivate = 1 , SecrecyConfidential = 2 } |
typedef ListBase< Incidence > | List |
![]() | |
enum | { SYNCNONE = 0 , SYNCMOD = 1 , SYNCDEL = 3 } |
Public Member Functions | |
Incidence (const Incidence &) | |
Incidence & | operator= (const Incidence &i) |
bool | operator== (const Incidence &) const |
virtual Incidence * | clone ()=0 |
void | setReadOnly (bool readonly) |
void | setFloats (bool f) |
void | recreate () |
void | setCreated (const TQDateTime &) |
TQDateTime | created () const |
void | setRevision (int rev) |
int | revision () const |
virtual void | setDtStart (const TQDateTime &dtStart) |
virtual TQDateTime | dtEnd () const |
void | setDescription (const TQString &description) |
TQString | description () const |
void | setSummary (const TQString &summary) |
TQString | summary () const |
void | setCategories (const TQStringList &categories) |
void | setCategories (const TQString &catStr) |
TQStringList | categories () const |
TQString | categoriesStr () const |
void | setRelatedToUid (const TQString &) |
TQString | relatedToUid () const |
void | setRelatedTo (Incidence *relatedTo) |
Incidence * | relatedTo () const |
Incidence::List | relations () const |
void | addRelation (Incidence *) |
void | removeRelation (Incidence *) |
Recurrence * | recurrence () const |
void | clearRecurrence () |
bool | doesRecur () const |
uint | recurrenceType () const |
virtual bool | recursOn (const TQDate &qd) const |
bool | recursAt (const TQDateTime &qdt) const |
virtual bool | recursOn (const TQDate &qd, Calendar *cal) const |
bool | recursAt (const TQDateTime &qdt, Calendar *cal) const |
virtual TQValueList< TQDateTime > | startDateTimesForDate (const TQDate &date) const |
virtual TQValueList< TQDateTime > | startDateTimesForDateTime (const TQDateTime &datetime) const |
virtual TQDateTime | endDateForStart (const TQDateTime &startDt) const |
void | addAttachment (Attachment *attachment) |
void | deleteAttachment (Attachment *attachment) |
void | deleteAttachments (const TQString &mime) |
Attachment::List | attachments () const |
Attachment::List | attachments (const TQString &mime) const |
void | clearAttachments () |
void | setSecrecy (int) |
int | secrecy () const |
TQString | secrecyStr () const |
void | setStatus (Status status) |
void | setCustomStatus (const TQString &status) |
Status | status () const |
TQString | statusStr () const |
void | setResources (const TQStringList &resources) |
TQStringList | resources () const |
void | setPriority (int priority) |
int | priority () const |
bool | hasRecurrenceID () const |
void | setHasRecurrenceID (bool hasRecurrenceID) |
void | setRecurrenceID (const TQDateTime &recurrenceID) |
TQDateTime | recurrenceID () const |
void | addChildIncidence (TQString childIncidence) |
void | deleteChildIncidence (TQString childIncidence) |
IncidenceList | childIncidences () const |
const Alarm::List & | alarms () const |
Alarm * | newAlarm () |
void | addAlarm (Alarm *) |
void | removeAlarm (Alarm *) |
void | clearAlarms () |
bool | isAlarmEnabled () const |
void | setLocation (const TQString &location) |
TQString | location () const |
void | setSchedulingID (const TQString &sid) |
TQString | schedulingID () const |
virtual void | recurrenceUpdated (Recurrence *) |
![]() | |
IncidenceBase (const IncidenceBase &) | |
IncidenceBase & | operator= (const IncidenceBase &i) |
bool | operator== (const IncidenceBase &) const |
virtual bool | accept (Visitor &) |
virtual TQCString | type () const =0 |
void | setUid (const TQString &) |
TQString | uid () const |
void | setLastModified (const TQDateTime &lm) |
TQDateTime | lastModified () const |
void | setOrganizer (const Person &o) |
void | setOrganizer (const TQString &o) |
Person | organizer () const |
virtual void | setReadOnly (bool) |
bool | isReadOnly () const |
virtual void | setDtStart (const TQDateTime &dtStart) |
virtual TQDateTime | dtStart () const |
virtual TDE_DEPRECATED TQString | dtStartTimeStr () const |
virtual TDE_DEPRECATED TQString | dtStartDateStr (bool shortfmt=true) const |
virtual TDE_DEPRECATED TQString | dtStartStr () const |
virtual void | setDuration (int seconds) |
int | duration () const |
void | setHasDuration (bool) |
bool | hasDuration () const |
bool | doesFloat () const |
void | setFloats (bool f) |
void | addComment (const TQString &comment) |
bool | removeComment (const TQString &comment) |
void | clearComments () |
TQStringList | comments () const |
void | addAttendee (Attendee *attendee, bool doUpdate=true) |
void | clearAttendees () |
const Attendee::List & | attendees () const |
int | attendeeCount () const |
Attendee * | attendeeByMail (const TQString &) const |
Attendee * | attendeeByMails (const TQStringList &, const TQString &email=TQString()) const |
Attendee * | attendeeByUid (const TQString &uid) const |
void | setSyncStatus (int status) |
void | setSyncStatusSilent (int status) |
int | syncStatus () const |
void | setPilotId (unsigned long id) |
unsigned long | pilotId () const |
void | registerObserver (Observer *) |
void | unRegisterObserver (Observer *) |
void | updated () |
void | updatedSilent () |
![]() | |
CustomProperties () | |
CustomProperties (const CustomProperties &) | |
bool | operator== (const CustomProperties &) const |
void | setCustomProperty (const TQCString &app, const TQCString &key, const TQString &value) |
void | removeCustomProperty (const TQCString &app, const TQCString &key) |
TQString | customProperty (const TQCString &app, const TQCString &key) const |
void | setNonKDECustomProperty (const TQCString &name, const TQString &value) |
void | removeNonKDECustomProperty (const TQCString &name) |
TQString | nonKDECustomProperty (const TQCString &name) const |
void | setCustomProperties (const TQMap< TQCString, TQString > &properties) |
TQMap< TQCString, TQString > | customProperties () const |
Static Public Member Functions | |
static TQStringList | secrecyList () |
static TQString | secrecyName (int) |
static TQString | statusName (Status) |
Protected Member Functions | |
virtual TQDateTime | endDateRecurrenceBase () const |
![]() | |
virtual void | customPropertyUpdated () |
virtual void | customPropertyUpdated () |
Additional Inherited Members | |
![]() | |
bool | mReadOnly |
Detailed Description
This class provides the base class common to all calendar components.
Definition at line 47 of file incidence.h.
Member Typedef Documentation
◆ List
typedef ListBase<Incidence> KCal::Incidence::List |
Definition at line 127 of file incidence.h.
Member Enumeration Documentation
◆ anonymous enum
anonymous enum |
enumeration for describing an event's secrecy.
Definition at line 125 of file incidence.h.
◆ Status
Constructor & Destructor Documentation
◆ Incidence() [1/2]
Incidence::Incidence | ( | ) |
Definition at line 34 of file incidence.cpp.
◆ Incidence() [2/2]
Incidence::Incidence | ( | const Incidence & | i | ) |
Definition at line 46 of file incidence.cpp.
◆ ~Incidence()
Incidence::~Incidence | ( | ) |
Definition at line 95 of file incidence.cpp.
Member Function Documentation
◆ addAlarm()
void Incidence::addAlarm | ( | Alarm * | alarm | ) |
Add an alarm which is associated with this incidence.
Definition at line 841 of file incidence.cpp.
◆ addAttachment()
void Incidence::addAttachment | ( | Attachment * | attachment | ) |
Add attachment.
Definition at line 674 of file incidence.cpp.
◆ addChildIncidence()
void Incidence::addChildIncidence | ( | TQString | childIncidence | ) |
Attach a child incidence to a parent incidence.
- Parameters
-
childIncidence is the child incidence to add
- Since
- 3.5.12
Definition at line 924 of file incidence.cpp.
◆ addRelation()
void Incidence::addRelation | ( | Incidence * | event | ) |
Add an event which is related to this event.
Definition at line 370 of file incidence.cpp.
◆ alarms()
const Alarm::List & Incidence::alarms | ( | ) | const |
All alarms that are associated with this incidence.
Definition at line 828 of file incidence.cpp.
◆ attachments() [1/2]
Attachment::List Incidence::attachments | ( | ) | const |
Return list of all associated attachments.
Definition at line 695 of file incidence.cpp.
◆ attachments() [2/2]
Attachment::List Incidence::attachments | ( | const TQString & | mime | ) | const |
Find a list of attachments with this mime type.
Definition at line 700 of file incidence.cpp.
◆ categories()
TQStringList Incidence::categories | ( | ) | const |
Return categories as a list of strings.
Definition at line 323 of file incidence.cpp.
◆ categoriesStr()
TQString Incidence::categoriesStr | ( | ) | const |
Return categories as a comma separated string.
Definition at line 328 of file incidence.cpp.
◆ childIncidences()
IncidenceList Incidence::childIncidences | ( | ) | const |
Returns an EventList of all child incidences.
- Returns
- EventList of all child incidences.
- Since
- 3.5.12
Definition at line 934 of file incidence.cpp.
◆ clearAlarms()
void Incidence::clearAlarms | ( | ) |
Remove all alarms that are associated with this incidence.
Definition at line 853 of file incidence.cpp.
◆ clearAttachments()
void Incidence::clearAttachments | ( | ) |
Remove and delete all attachments.
Definition at line 711 of file incidence.cpp.
◆ clearRecurrence()
void Incidence::clearRecurrence | ( | ) |
Removes all recurrence and exception rules and dates.
Definition at line 404 of file incidence.cpp.
◆ clone()
|
pure virtual |
Return copy of this object.
The returned object is owned by the caller.
Implemented in KCal::Event, KCal::Journal, and KCal::Todo.
◆ created()
TQDateTime Incidence::created | ( | ) | const |
Return time and date of creation.
Definition at line 246 of file incidence.cpp.
◆ deleteAttachment()
void Incidence::deleteAttachment | ( | Attachment * | attachment | ) |
Remove and delete a specific attachment.
Definition at line 681 of file incidence.cpp.
◆ deleteAttachments()
void Incidence::deleteAttachments | ( | const TQString & | mime | ) |
Remove and delete all attachments with this mime type.
Definition at line 686 of file incidence.cpp.
◆ deleteChildIncidence()
void Incidence::deleteChildIncidence | ( | TQString | childIncidence | ) |
Detach a child incidence from its parent incidence.
- Parameters
-
childIncidence is the child incidence to remove
- Since
- 3.5.12
Definition at line 929 of file incidence.cpp.
◆ description()
TQString Incidence::description | ( | ) | const |
Return long description.
Definition at line 280 of file incidence.cpp.
◆ doesRecur()
bool Incidence::doesRecur | ( | ) | const |
Forward to Recurrence::doesRecur().
Definition at line 416 of file incidence.cpp.
◆ dtEnd()
|
inlinevirtual |
Return the incidence's ending date/time as a TQDateTime.
Reimplemented in KCal::Event.
Definition at line 184 of file incidence.h.
◆ endDateForStart()
|
virtual |
Return the end time of the occurrence if it starts at the given date/time.
Definition at line 573 of file incidence.cpp.
◆ endDateRecurrenceBase()
|
inlineprotectedvirtual |
Return the end date/time of the base incidence (e.g.
due date/time for to-dos, end date/time for events). This method needs to be reimplemented by derived classes.
Reimplemented in KCal::Event, and KCal::Todo.
Definition at line 552 of file incidence.h.
◆ hasRecurrenceID()
bool Incidence::hasRecurrenceID | ( | ) | const |
Returns true if the incidence has recurrenceID, otherwise return false.
- See also
- setHasRecurrenceID(), setRecurrenceID(TQDateTime)
- Since
- 3.5.12
Definition at line 893 of file incidence.cpp.
◆ isAlarmEnabled()
bool Incidence::isAlarmEnabled | ( | ) | const |
Return whether any alarm associated with this incidence is enabled.
Definition at line 859 of file incidence.cpp.
◆ location()
TQString Incidence::location | ( | ) | const |
Return the event's/todo's location.
Do not use it with journal.
Definition at line 875 of file incidence.cpp.
◆ newAlarm()
Alarm * Incidence::newAlarm | ( | ) |
Create a new alarm which is associated with this incidence.
Definition at line 833 of file incidence.cpp.
◆ operator=()
Definition at line 113 of file incidence.cpp.
◆ operator==()
bool Incidence::operator== | ( | const Incidence & | i2 | ) | const |
Definition at line 162 of file incidence.cpp.
◆ priority()
int Incidence::priority | ( | ) | const |
Return priority.
The priority is a number between 1 and 9. 1 is highest priority. If the priority is undefined 0 is returned.
Definition at line 736 of file incidence.cpp.
◆ recreate()
void Incidence::recreate | ( | ) |
Recreate event.
The event is made a new unique event, but already stored event information is preserved. Sets uniquie id, creation date, last modification date and revision number.
Definition at line 208 of file incidence.cpp.
◆ recurrence()
Recurrence * Incidence::recurrence | ( | ) | const |
Return the recurrence rule associated with this incidence.
If there is none, returns an appropriate (non-0) object.
Definition at line 390 of file incidence.cpp.
◆ recurrenceID()
TQDateTime Incidence::recurrenceID | ( | ) | const |
Returns the incidence recurrenceID.
- Returns
- incidences recurrenceID value
- See also
- setRecurrenceID().
- Since
- 3.5.12
Definition at line 908 of file incidence.cpp.
◆ recurrenceType()
uint Incidence::recurrenceType | ( | ) | const |
Definition at line 410 of file incidence.cpp.
◆ recurrenceUpdated()
|
virtual |
Observer interface for the recurrence class.
If the recurrence is changed, this method will be called for the incidence the recurrence object belongs to.
Definition at line 942 of file incidence.cpp.
◆ recursAt() [1/2]
bool Incidence::recursAt | ( | const TQDateTime & | qdt | ) | const |
Returns true if the date/time specified is one on which the incidence will recur.
Definition at line 430 of file incidence.cpp.
◆ recursAt() [2/2]
bool Incidence::recursAt | ( | const TQDateTime & | qdt, |
Calendar * | cal | ||
) | const |
Returns true if the date/time specified is one on which the incidence will recur.
This function takes RECURRENCE-ID parameters into account
- Parameters
-
cal the calendar owning the incidence
Definition at line 459 of file incidence.cpp.
◆ recursOn() [1/2]
|
virtual |
Returns true if the date specified is one on which the incidence will recur.
Reimplemented in KCal::Todo.
Definition at line 422 of file incidence.cpp.
◆ recursOn() [2/2]
|
virtual |
Returns true if the date specified is one on which the incidence will recur.
This function takes RECURRENCE-ID parameters into account
- Parameters
-
cal the calendar owning the incidence
Definition at line 438 of file incidence.cpp.
◆ relatedTo()
Incidence * Incidence::relatedTo | ( | ) | const |
What event does this one relate to?
Definition at line 360 of file incidence.cpp.
◆ relatedToUid()
TQString Incidence::relatedToUid | ( | ) | const |
What event does this one relate to? This function should only be used when constructing a calendar before the related Incidence exists.
Definition at line 340 of file incidence.cpp.
◆ relations()
Incidence::List Incidence::relations | ( | ) | const |
All events that are related to this event.
Definition at line 365 of file incidence.cpp.
◆ removeAlarm()
void Incidence::removeAlarm | ( | Alarm * | alarm | ) |
Remove an alarm that is associated with this incidence.
Definition at line 847 of file incidence.cpp.
◆ removeRelation()
void Incidence::removeRelation | ( | Incidence * | event | ) |
Remove event that is related to this event.
Definition at line 377 of file incidence.cpp.
◆ resources()
TQStringList Incidence::resources | ( | ) | const |
Return list of current resources.
Definition at line 723 of file incidence.cpp.
◆ revision()
int Incidence::revision | ( | ) | const |
Return the number of revisions this event has seen.
Definition at line 259 of file incidence.cpp.
◆ schedulingID()
TQString Incidence::schedulingID | ( | ) | const |
Return the event's/todo's scheduling ID.
Does not make sense for journals If this is not set, it will return uid().
Definition at line 885 of file incidence.cpp.
◆ secrecy()
int Incidence::secrecy | ( | ) | const |
Return the event's secrecy.
Definition at line 793 of file incidence.cpp.
◆ secrecyList()
|
static |
Return list of all available secrecy states as list of translated strings.
Definition at line 817 of file incidence.cpp.
◆ secrecyName()
|
static |
Return human-readable translated name of secrecy class.
Definition at line 803 of file incidence.cpp.
◆ secrecyStr()
TQString Incidence::secrecyStr | ( | ) | const |
Return secrecy as translated string.
Definition at line 798 of file incidence.cpp.
◆ setCategories() [1/2]
void Incidence::setCategories | ( | const TQString & | catStr | ) |
Set categories based on a comma delimited string.
Definition at line 306 of file incidence.cpp.
◆ setCategories() [2/2]
void Incidence::setCategories | ( | const TQStringList & | categories | ) |
Set categories.
Definition at line 298 of file incidence.cpp.
◆ setCreated()
void Incidence::setCreated | ( | const TQDateTime & | created | ) |
Set creation date.
Definition at line 237 of file incidence.cpp.
◆ setCustomStatus()
void Incidence::setCustomStatus | ( | const TQString & | status | ) |
Sets the incidence status to a non-standard status value.
- Parameters
-
status non-standard status string. If empty, the incidence status will be set to StatusNone.
Definition at line 749 of file incidence.cpp.
◆ setDescription()
void Incidence::setDescription | ( | const TQString & | description | ) |
Set the long description.
Definition at line 273 of file incidence.cpp.
◆ setDtStart()
|
virtual |
Set starting date/time.
Reimplemented from KCal::IncidenceBase.
Reimplemented in KCal::Todo.
Definition at line 264 of file incidence.cpp.
◆ setFloats()
void Incidence::setFloats | ( | bool | f | ) |
Set whether the incidence floats, i.e.
has a date but no time attached to it.
Definition at line 229 of file incidence.cpp.
◆ setHasRecurrenceID()
void Incidence::setHasRecurrenceID | ( | bool | hasRecurrenceID | ) |
Sets if the incidence has recurrenceID.
- Parameters
-
hasRecurrenceID true if incidence has recurrenceID, otherwise false
- See also
- hasRecurrenceID(), recurrenceID()
- Since
- 3.5.12
Definition at line 898 of file incidence.cpp.
◆ setLocation()
void Incidence::setLocation | ( | const TQString & | location | ) |
Set the event's/todo's location.
Do not use it with journal.
Definition at line 868 of file incidence.cpp.
◆ setPriority()
void Incidence::setPriority | ( | int | priority | ) |
Set the incidences priority.
The priority has to be a value between 0 and 9, 0 is undefined, 1 the highest, 9 the lowest priority (decreasing order).
Definition at line 729 of file incidence.cpp.
◆ setReadOnly()
|
virtual |
Set readonly state of incidence.
- Parameters
-
readonly If true, the incidence is set to readonly, if false the incidence is set to readwrite.
Reimplemented from KCal::IncidenceBase.
Definition at line 222 of file incidence.cpp.
◆ setRecurrenceID()
void Incidence::setRecurrenceID | ( | const TQDateTime & | recurrenceID | ) |
Set the incidences recurrenceID.
- Parameters
-
recurrenceID is the incidence recurrenceID to set
- See also
- recurrenceID().
- Since
- 3.5.12
Definition at line 913 of file incidence.cpp.
◆ setRelatedTo()
void Incidence::setRelatedTo | ( | Incidence * | relatedTo | ) |
Point at some other event to which the event relates.
Definition at line 345 of file incidence.cpp.
◆ setRelatedToUid()
void Incidence::setRelatedToUid | ( | const TQString & | relatedToUid | ) |
Point at some other event to which the event relates.
This function should only be used when constructing a calendar before the related Incidence exists.
Definition at line 333 of file incidence.cpp.
◆ setResources()
void Incidence::setResources | ( | const TQStringList & | resources | ) |
Set resources used, such as Office, Car, etc.
Definition at line 716 of file incidence.cpp.
◆ setRevision()
void Incidence::setRevision | ( | int | rev | ) |
Set the number of revisions this event has seen.
Definition at line 251 of file incidence.cpp.
◆ setSchedulingID()
void Incidence::setSchedulingID | ( | const TQString & | sid | ) |
Set the event's/todo's scheduling ID.
Does not make sense for journals. This is used for accepted invitations as the place to store the UID of the invitation. It is later used again if updates to the invitation comes in. If we did not set a new UID on incidences from invitations, we can end up with more than one resource having events with the same UID, if you have access to other peoples resources.
Definition at line 880 of file incidence.cpp.
◆ setSecrecy()
void Incidence::setSecrecy | ( | int | sec | ) |
Sets secrecy status.
This can be Public, Private or Confidential. See separate enum.
Definition at line 786 of file incidence.cpp.
◆ setStatus()
void Incidence::setStatus | ( | Incidence::Status | status | ) |
Sets the incidence status to a standard status value.
See separate enum. Note that StatusX cannot be specified.
Definition at line 741 of file incidence.cpp.
◆ setSummary()
void Incidence::setSummary | ( | const TQString & | summary | ) |
Set short summary.
Definition at line 286 of file incidence.cpp.
◆ startDateTimesForDate()
|
virtual |
Calculates the start date/time for all recurrences that happen at some time on the given date (might start before that date, but end on or after the given date).
- Parameters
-
date the date where the incidence should occur
- Returns
- the start date/time of all occurences that overlap with the given date. Empty list if the incidence does not overlap with the date at all
Definition at line 486 of file incidence.cpp.
◆ startDateTimesForDateTime()
|
virtual |
Calculates the start date/time for all recurrences that happen at the given time.
- Parameters
-
datetime the date/time where the incidence should occur
- Returns
- the start date/time of all occurences that overlap with the given date/time. Empty list if the incidence does not happen at the given time at all.
Definition at line 533 of file incidence.cpp.
◆ status()
Incidence::Status Incidence::status | ( | ) | const |
Return the event's status.
Definition at line 757 of file incidence.cpp.
◆ statusName()
|
static |
Return human-readable translated name of status value.
Definition at line 769 of file incidence.cpp.
◆ statusStr()
TQString Incidence::statusStr | ( | ) | const |
Return the event's status string.
Definition at line 762 of file incidence.cpp.
◆ summary()
TQString Incidence::summary | ( | ) | const |
Return short summary.
Definition at line 293 of file incidence.cpp.
The documentation for this class was generated from the following files: