#include <resourcecalendar.h>
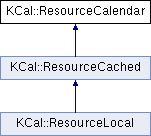
Public Slots | |
virtual void | setSubresourceActive (const TQString &, bool active) |
Signals | |
void | resourceChanged (ResourceCalendar *) |
void | resourceLoaded (ResourceCalendar *) |
void | resourceSaved (ResourceCalendar *) |
void | resourceLoadError (ResourceCalendar *, const TQString &error) |
void | resourceSaveError (ResourceCalendar *, const TQString &error) |
void | signalSubresourceAdded (ResourceCalendar *, const TQString &type, const TQString &subresource, const TQString &label) |
void | signalSubresourceRemoved (ResourceCalendar *, const TQString &, const TQString &) |
Public Member Functions | |
ResourceCalendar (const TDEConfig *) | |
void | clearException () |
void | setException (ErrorFormat *error) |
ErrorFormat * | exception () |
void | setResolveConflict (bool b) |
virtual void | writeConfig (TDEConfig *config) |
virtual TQString | infoText () const |
bool | load () |
bool | save (Incidence *incidence=0) |
virtual bool | isSaving () |
virtual TDEABC::Lock * | lock ()=0 |
virtual TDE_DEPRECATED bool | addIncidence (Incidence *) |
virtual bool | addIncidence (Incidence *, const TQString &subresource) |
virtual bool | deleteIncidence (Incidence *) |
Incidence * | incidence (const TQString &uid) |
virtual TDE_DEPRECATED bool | addEvent (Event *event)=0 |
virtual bool | addEvent (Event *event, const TQString &subresource)=0 |
virtual bool | deleteEvent (Event *)=0 |
virtual Event * | event (const TQString &uid)=0 |
virtual Event::List | rawEvents (EventSortField sortField=EventSortUnsorted, SortDirection sortDirection=SortDirectionAscending)=0 |
virtual Event::List | rawEventsForDate (const TQDate &date, EventSortField sortField=EventSortUnsorted, SortDirection sortDirection=SortDirectionAscending)=0 |
virtual Event::List | rawEventsForDate (const TQDateTime &qdt)=0 |
virtual Event::List | rawEvents (const TQDate &start, const TQDate &end, bool inclusive=false)=0 |
virtual bool | setValue (const TQString &key, const TQString &value) |
virtual TDE_DEPRECATED bool | addTodo (Todo *todo)=0 |
virtual bool | addTodo (Todo *todo, const TQString &subresource)=0 |
virtual bool | deleteTodo (Todo *)=0 |
virtual Todo * | todo (const TQString &uid)=0 |
virtual Todo::List | rawTodos (TodoSortField sortField=TodoSortUnsorted, SortDirection sortDirection=SortDirectionAscending)=0 |
virtual Todo::List | rawTodosForDate (const TQDate &date)=0 |
virtual TDE_DEPRECATED bool | addJournal (Journal *)=0 |
virtual bool | addJournal (Journal *journal, const TQString &subresource)=0 |
virtual bool | deleteJournal (Journal *)=0 |
virtual Journal * | journal (const TQString &uid)=0 |
virtual Journal::List | rawJournals (JournalSortField sortField=JournalSortUnsorted, SortDirection sortDirection=SortDirectionAscending)=0 |
virtual Journal::List | rawJournalsForDate (const TQDate &date)=0 |
virtual Alarm::List | alarms (const TQDateTime &from, const TQDateTime &to)=0 |
virtual Alarm::List | alarmsTo (const TQDateTime &to)=0 |
Incidence::List | rawIncidences () |
virtual void | setTimeZoneId (const TQString &timeZoneId)=0 |
virtual TQStringList | subresources () const |
virtual bool | canHaveSubresources () const |
virtual bool | subresourceActive (const TQString &) const |
virtual bool | subresourceWritable (const TQString &) const |
virtual const TQString | labelForSubresource (const TQString &resource) const |
virtual TQString | subresourceIdentifier (Incidence *incidence) |
virtual bool | removeSubresource (const TQString &resource) |
virtual bool | addSubresource (const TQString &resource, const TQString &parent) |
virtual TQString | subresourceType (const TQString &resource) |
virtual void | beginAddingIncidences () |
virtual void | endAddingIncidences () |
Protected Member Functions | |
virtual bool | doLoad ()=0 |
virtual bool | doSave ()=0 |
virtual bool | doSave (Incidence *) |
virtual void | addInfoText (TQString &) const |
void | loadError (const TQString &errorMessage=TQString()) |
void | saveError (const TQString &errorMessage=TQString()) |
Protected Attributes | |
bool | mResolveConflict |
Detailed Description
This class provides the interfaces for a calendar resource.
It makes use of the tderesources framework.
- Warning
- This code is still under heavy development. Don't expect source or binary compatibility in future versions.
Definition at line 57 of file resourcecalendar.h.
Member Function Documentation
◆ addEvent()
|
pure virtual |
Add event to resource.
- Deprecated:
- use addEvent(Event *,const TQString&) instead.
Implemented in KCal::ResourceCached.
◆ addIncidence() [1/2]
|
virtual |
Add incidence to resource.
Definition at line 101 of file resourcecalendar.cpp.
◆ addIncidence() [2/2]
|
virtual |
Add incidence to resource and subresource.
Definition at line 107 of file resourcecalendar.cpp.
◆ addInfoText()
|
inlineprotectedvirtual |
Add info text for concrete resources.
Called by infoText().
Reimplemented in KCal::ResourceCached.
Definition at line 439 of file resourcecalendar.h.
◆ addJournal()
|
pure virtual |
Add a Journal entry to the resource.
- Deprecated:
- use addJournal(Journal *,const TQString &) instead.
Implemented in KCal::ResourceCached.
◆ addSubresource()
|
virtual |
Add a subresource with the name.
- Parameters
-
resource and the parent id parent.
Definition at line 128 of file resourcecalendar.cpp.
◆ addTodo()
|
pure virtual |
Add a todo to the todolist.
- Deprecated:
- use addTodo(Todo *,const TQString &) instead.
Implemented in KCal::ResourceCached.
◆ alarms()
|
pure virtual |
Return all alarms which occur in the given time interval.
Implemented in KCal::ResourceCached.
◆ alarmsTo()
|
pure virtual |
Return all alarms which occur before given date.
Implemented in KCal::ResourceCached.
◆ beginAddingIncidences()
|
virtual |
Called when we starting adding a batch of incidences.
So we don't show the same warnings for each incidence.
Definition at line 241 of file resourcecalendar.cpp.
◆ canHaveSubresources()
|
inlinevirtual |
Is this subresource capable of having subresources or not?
Definition at line 351 of file resourcecalendar.h.
◆ clearException()
void ResourceCalendar::clearException | ( | ) |
Clears the exception status.
Definition at line 46 of file resourcecalendar.cpp.
◆ deleteEvent()
|
pure virtual |
Delete event from this resource.
Implemented in KCal::ResourceCached.
◆ deleteIncidence()
|
virtual |
Delete incidence from resource.
Definition at line 113 of file resourcecalendar.cpp.
◆ deleteJournal()
|
pure virtual |
Remove a Journal entry from calendar.
Implemented in KCal::ResourceCached.
◆ deleteTodo()
|
pure virtual |
Remove a todo from the todolist.
Implemented in KCal::ResourceCached.
◆ doLoad()
|
protectedpure virtual |
◆ doSave() [1/2]
|
protectedpure virtual |
◆ doSave() [2/2]
|
protectedvirtual |
Do the actual saving of the resource data.
Called by save(). Save one Incidence. The default implementation calls doSave() to save everything
Definition at line 201 of file resourcecalendar.cpp.
◆ endAddingIncidences()
|
virtual |
Called when we finish adding a batch of incidences.
- See also
- beginAddingIncidences()
Definition at line 245 of file resourcecalendar.cpp.
◆ event()
|
pure virtual |
Retrieves an event on the basis of the unique string ID.
Implemented in KCal::ResourceCached.
◆ exception()
ErrorFormat * ResourceCalendar::exception | ( | ) |
Returns an exception, if there is any, containing information about the last error that occurred.
Definition at line 58 of file resourcecalendar.cpp.
◆ incidence()
Incidence * ResourceCalendar::incidence | ( | const TQString & | uid | ) |
Return incidence with given unique id.
If there is no incidence with that uid, return 0.
Definition at line 91 of file resourcecalendar.cpp.
◆ infoText()
|
virtual |
Return rich text with info about the resource.
Adds standard info and then calls addInfoText() to add info about concrete resources.
Definition at line 68 of file resourcecalendar.cpp.
◆ isSaving()
|
inlinevirtual |
Return true if a save operation is still in progress, otherwise return false.
Definition at line 136 of file resourcecalendar.h.
◆ journal()
|
pure virtual |
Return Journal with given unique id.
Implemented in KCal::ResourceCached.
◆ labelForSubresource()
|
inlinevirtual |
What is the label for this subresource?
Definition at line 366 of file resourcecalendar.h.
◆ load()
bool ResourceCalendar::load | ( | ) |
Load resource data.
After calling this function all data is accessible by calling the incidence/event/todo/etc. accessor functions.
Whether data is actually loaded within this function or the loading is delayed until it is accessed by another function depends on the implementation of the resource.
If loading the data takes significant time, the resource should return cached values if available, and return the results via the resourceChanged signal. When the resource has finished loading, the resourceLoaded() signal is emitted.
Calling this function multiple times should have the same effect as calling it once, given that the data isn't changed between calls.
This function calls doLoad() which has to be reimplented by the resource to do the actual loading.
Definition at line 138 of file resourcecalendar.cpp.
◆ loadError()
|
protected |
A resource should call this function if a load error happens.
Definition at line 169 of file resourcecalendar.cpp.
◆ lock()
|
pure virtual |
Return object for locking the resource.
Implemented in KCal::ResourceLocal.
◆ rawEvents() [1/2]
|
pure virtual |
Get unfiltered events in a range of dates.
If inclusive is set to true, only events which are completely included in the range are returned.
Implemented in KCal::ResourceCached.
◆ rawEvents() [2/2]
|
pure virtual |
Return unfiltered list of all events in calendar.
Use with care, this can be a bad idea for server-based calendars.
Implemented in KCal::ResourceCached.
◆ rawEventsForDate() [1/2]
|
pure virtual |
Builds and then returns a list of all events that match the date specified.
Useful for dayView, etc. etc.
Implemented in KCal::ResourceCached.
◆ rawEventsForDate() [2/2]
|
pure virtual |
Get unfiltered events for date qdt.
Implemented in KCal::ResourceCached.
◆ rawIncidences()
Incidence::List ResourceCalendar::rawIncidences | ( | ) |
Returns a list of all incideces.
Definition at line 119 of file resourcecalendar.cpp.
◆ rawJournals()
|
pure virtual |
Return list of all journals.
Implemented in KCal::ResourceCached.
◆ rawJournalsForDate()
|
pure virtual |
Returns list of journals for the given date.
Implemented in KCal::ResourceCached.
◆ rawTodos()
|
pure virtual |
Return list of all todos.
Implemented in KCal::ResourceCached.
◆ rawTodosForDate()
|
pure virtual |
Returns list of todos due on the specified date.
Implemented in KCal::ResourceCached.
◆ removeSubresource()
|
virtual |
Remove a subresource with the id.
- Parameters
-
resource
Definition at line 133 of file resourcecalendar.cpp.
◆ resourceChanged
|
signal |
This signal is emitted when the data in the resource has changed.
The resource has to make sure that this signal is emitted whenever any pointers to incidences which the resource has previously given to the calling code, become invalid.
◆ resourceLoaded
|
signal |
This signal is emitted when loading data into the resource has been finished.
◆ resourceLoadError
|
signal |
This signal is emitted when an error occurs during loading.
◆ resourceSaved
|
signal |
This signal is emitted when saving the data of the resource has been finished.
◆ resourceSaveError
|
signal |
This signal is emitted when an error occurs during saving.
◆ save()
bool ResourceCalendar::save | ( | Incidence * | incidence = 0 | ) |
Save resource data.
After calling this function it is safe to close the resource without losing data.
Whether data is actually saved within this function or saving is delayed depends on the implementation of the resource.
If saving the data takes significant time, the resource should return from the function, do the saving in the background and notify the end of the save by emitting the signal resourceSaved().
This function calls doSave() which has to be reimplented by the resource to do the actual saving.
- Parameters
-
incidence if given as 0, doSave() is called to save all incidences, else doSave(incidence) is called to save only the given one
Definition at line 182 of file resourcecalendar.cpp.
◆ saveError()
|
protected |
A resource should call this function if a save error happens.
Definition at line 206 of file resourcecalendar.cpp.
◆ setException()
void ResourceCalendar::setException | ( | ErrorFormat * | error | ) |
Set exception for this object.
This is used by the functions of this class to report errors.
Definition at line 52 of file resourcecalendar.cpp.
◆ setSubresourceActive
|
virtualslot |
(De-)activate a subresource.
Definition at line 124 of file resourcecalendar.cpp.
◆ setTimeZoneId()
|
pure virtual |
◆ setValue()
|
virtual |
Sets a particular value of the resource's configuration.
The possible keys are resource specific.
This method is provided to make it possible to set resource-type specific settings without actually linking to the resource's library. Its use is discouraged, but in some situations the only possibility to avoid unwanted compiling and linking dependencies. E.g. if you don't want to link to the remote resource, but need to create a remote resource at the URL given in yourURL, you can use code like the following: KCal::ResourceCalendar *res = manager->createResource( "remote" ); if ( res ) { res->setTimeZoneId( timezone ); res->setResourceName( i18n("Test resource") ); res->setValue( "DownloadURL", yourURL ); manager->add( res ); }
Reimplemented in KCal::ResourceLocal.
Definition at line 219 of file resourcecalendar.cpp.
◆ signalSubresourceAdded
|
signal |
This signal is emitted when a subresource is added.
◆ signalSubresourceRemoved
|
signal |
This signal is emitted when a subresource is removed.
◆ subresourceActive()
|
inlinevirtual |
Is this subresource active or not?
Definition at line 356 of file resourcecalendar.h.
◆ subresourceIdentifier()
|
inlinevirtual |
Get the identifier of the subresource associated with a specified incidence.
- Returns
- the identifier of the subresource or an empty string.
Definition at line 378 of file resourcecalendar.h.
◆ subresources()
|
inlinevirtual |
If this resource has subresources, return a TQStringList of them.
In most cases, resources do not have subresources, so this is by default just empty.
Definition at line 346 of file resourcecalendar.h.
◆ subresourceType()
|
virtual |
Returns the type of the subresource: "event", "todo" or "journal", TQString() if unknown/mixed.
Definition at line 226 of file resourcecalendar.cpp.
◆ subresourceWritable()
|
virtual |
Is this subresource writable or not?
Definition at line 232 of file resourcecalendar.cpp.
◆ todo()
|
pure virtual |
Searches todolist for an event with this unique id.
- Returns
- pointer to todo or 0 if todo wasn't found
Implemented in KCal::ResourceCached.
The documentation for this class was generated from the following files: