#include <calendarlocal.h>
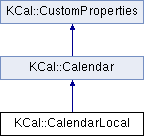
Public Member Functions | |
CalendarLocal (const TQString &timeZoneId) | |
bool | load (const TQString &fileName, CalFormat *format=0) |
bool | reload (const TQString &tz) |
bool | save (const TQString &fileName, CalFormat *format=0) |
void | close () |
void | closeEvents () |
void | closeTodos () |
void | closeJournals () |
void | save () |
bool | addEvent (Event *event) |
bool | deleteEvent (Event *event) |
bool | deleteChildEvents (Event *event) |
void | deleteAllEvents () |
Event * | event (const TQString &uid) |
Event::List | rawEvents (EventSortField sortField=EventSortUnsorted, SortDirection sortDirection=SortDirectionAscending) |
bool | addTodo (Todo *todo) |
bool | deleteTodo (Todo *) |
bool | deleteChildTodos (Todo *todo) |
void | deleteAllTodos () |
Todo * | todo (const TQString &uid) |
Todo::List | rawTodos (TodoSortField sortField=TodoSortUnsorted, SortDirection sortDirection=SortDirectionAscending) |
Todo::List | rawTodosForDate (const TQDate &date) |
bool | addJournal (Journal *) |
bool | deleteJournal (Journal *) |
bool | deleteChildJournals (Journal *journal) |
void | deleteAllJournals () |
Journal * | journal (const TQString &uid) |
Journal::List | rawJournals (JournalSortField sortField=JournalSortUnsorted, SortDirection sortDirection=SortDirectionAscending) |
Journal::List | rawJournalsForDate (const TQDate &date) |
Alarm::List | alarms (const TQDateTime &from, const TQDateTime &to) |
Alarm::List | alarmsTo (const TQDateTime &to) |
Event::List | rawEventsForDate (const TQDate &date, EventSortField sortField=EventSortUnsorted, SortDirection sortDirection=SortDirectionAscending) |
Event::List | rawEventsForDate (const TQDateTime &qdt) |
Event::List | rawEvents (const TQDate &start, const TQDate &end, bool inclusive=false) |
void | setTimeZoneIdViewOnly (const TQString &tz) |
![]() | |
Calendar (const TQString &timeZoneId) | |
virtual | ~Calendar () |
void | setProductId (const TQString &productId) |
TQString | productId () |
void | clearException () |
ErrorFormat * | exception () const |
void | setOwner (const Person &owner) |
const Person & | getOwner () const |
void | setTimeZoneId (const TQString &timeZoneId) |
virtual void | setTimeZoneIdViewOnly (const TQString &timeZoneId)=0 |
TQString | timeZoneId () const |
void | setLocalTime () |
bool | isLocalTime () const |
void | setModified (bool modified) |
bool | isModified () const |
virtual void | close ()=0 |
virtual void | closeEvents ()=0 |
virtual void | closeTodos ()=0 |
virtual void | closeJournals ()=0 |
virtual void | save ()=0 |
virtual bool | reload (const TQString &tz)=0 |
virtual bool | isSaving () |
TQStringList | categories () |
virtual bool | addIncidence (Incidence *incidence) |
virtual bool | deleteIncidence (Incidence *incidence) |
virtual Incidence::List | incidences () |
virtual Incidence::List | incidences (const TQDate &date) |
virtual Incidence::List | rawIncidences () |
Incidence * | incidence (const TQString &uid) |
Incidence * | incidenceFromSchedulingID (const TQString &sid) |
Incidence::List | incidencesFromSchedulingID (const TQString &UID) |
virtual bool | beginChange (Incidence *incidence) |
virtual bool | endChange (Incidence *incidence) |
Incidence * | dissociateOccurrence (Incidence *incidence, TQDate date, bool single=true) |
virtual bool | addEvent (Event *event)=0 |
virtual bool | deleteEvent (Event *event)=0 |
virtual Event::List | events (EventSortField sortField=EventSortUnsorted, SortDirection sortDirection=SortDirectionAscending) |
Event::List | events (const TQDateTime &qdt) |
Event::List | events (const TQDate &start, const TQDate &end, bool inclusive=false) |
Event::List | events (const TQDate &date, EventSortField sortField=EventSortUnsorted, SortDirection sortDirection=SortDirectionAscending) |
virtual Event::List | rawEvents (EventSortField sortField=EventSortUnsorted, SortDirection sortDirection=SortDirectionAscending)=0 |
virtual Event::List | rawEventsForDate (const TQDateTime &qdt)=0 |
virtual Event::List | rawEvents (const TQDate &start, const TQDate &end, bool inclusive=false)=0 |
virtual Event::List | rawEventsForDate (const TQDate &date, EventSortField sortField=EventSortUnsorted, SortDirection sortDirection=SortDirectionAscending)=0 |
virtual Event * | event (const TQString &uid)=0 |
virtual bool | addTodo (Todo *todo)=0 |
virtual bool | deleteTodo (Todo *todo)=0 |
virtual Todo::List | todos (TodoSortField sortField=TodoSortUnsorted, SortDirection sortDirection=SortDirectionAscending) |
virtual Todo::List | todos (const TQDate &date) |
virtual Todo::List | rawTodos (TodoSortField sortField=TodoSortUnsorted, SortDirection sortDirection=SortDirectionAscending)=0 |
virtual Todo::List | rawTodosForDate (const TQDate &date)=0 |
virtual Todo * | todo (const TQString &uid)=0 |
virtual bool | addJournal (Journal *journal)=0 |
virtual bool | deleteJournal (Journal *journal)=0 |
virtual Journal::List | journals (JournalSortField sortField=JournalSortUnsorted, SortDirection sortDirection=SortDirectionAscending) |
virtual Journal::List | journals (const TQDate &date) |
virtual Journal::List | rawJournals (JournalSortField sortField=JournalSortUnsorted, SortDirection sortDirection=SortDirectionAscending)=0 |
virtual Journal::List | rawJournalsForDate (const TQDate &date)=0 |
virtual Journal * | journal (const TQString &uid)=0 |
void | beginBatchAdding () |
void | endBatchAdding () |
virtual void | setupRelations (Incidence *incidence) |
virtual void | removeRelations (Incidence *incidence) |
void | setFilter (CalFilter *filter) |
CalFilter * | filter () |
virtual Alarm::List | alarms (const TQDateTime &from, const TQDateTime &to)=0 |
void | registerObserver (Observer *observer) |
void | unregisterObserver (Observer *observer) |
![]() | |
CustomProperties () | |
CustomProperties (const CustomProperties &) | |
bool | operator== (const CustomProperties &) const |
void | setCustomProperty (const TQCString &app, const TQCString &key, const TQString &value) |
void | removeCustomProperty (const TQCString &app, const TQCString &key) |
TQString | customProperty (const TQCString &app, const TQCString &key) const |
void | setNonKDECustomProperty (const TQCString &name, const TQString &value) |
void | removeNonKDECustomProperty (const TQCString &name) |
TQString | nonKDECustomProperty (const TQCString &name) const |
void | setCustomProperties (const TQMap< TQCString, TQString > &properties) |
TQMap< TQCString, TQString > | customProperties () const |
Protected Member Functions | |
void | incidenceUpdated (IncidenceBase *i) |
void | insertEvent (Event *event) |
void | appendAlarms (Alarm::List &alarms, Incidence *incidence, const TQDateTime &from, const TQDateTime &to) |
void | appendRecurringAlarms (Alarm::List &alarms, Incidence *incidence, const TQDateTime &from, const TQDateTime &to) |
![]() | |
void | setException (ErrorFormat *e) |
void | incidenceUpdated (IncidenceBase *incidenceBase) |
virtual void | doSetTimeZoneId (const TQString &) |
void | notifyIncidenceAdded (Incidence *incidence) |
void | notifyIncidenceChanged (Incidence *incidence) |
void | notifyIncidenceDeleted (Incidence *incidence) |
virtual void | customPropertyUpdated () |
void | setObserversEnabled (bool enabled) |
virtual void | customPropertyUpdated () |
Additional Inherited Members | |
![]() | |
void | calendarChanged () |
void | calendarSaved () |
void | calendarLoaded () |
void | batchAddingBegins () |
void | batchAddingEnds () |
![]() | |
static Incidence::List | mergeIncidenceList (const Event::List &events, const Todo::List &todos, const Journal::List &journals) |
static Event::List | sortEvents (Event::List *eventList, EventSortField sortField, SortDirection sortDirection) |
static Event::List | sortEventsForDate (Event::List *eventList, const TQDate &date, EventSortField sortField, SortDirection sortDirection) |
static Todo::List | sortTodos (Todo::List *todoList, TodoSortField sortField, SortDirection sortDirection) |
static Journal::List | sortJournals (Journal::List *journalList, JournalSortField sortField, SortDirection sortDirection) |
Detailed Description
This class provides a calendar stored as a local file.
Definition at line 36 of file calendarlocal.h.
Constructor & Destructor Documentation
◆ CalendarLocal()
CalendarLocal::CalendarLocal | ( | const TQString & | timeZoneId | ) |
Constructs a new calendar, with variables initialized to sane values.
Definition at line 44 of file calendarlocal.cpp.
◆ ~CalendarLocal()
CalendarLocal::~CalendarLocal | ( | ) |
Definition at line 57 of file calendarlocal.cpp.
Member Function Documentation
◆ addEvent()
|
virtual |
◆ addJournal()
|
virtual |
Add a Journal entry to calendar.
Implements KCal::Calendar.
Definition at line 652 of file calendarlocal.cpp.
◆ addTodo()
|
virtual |
Add a todo to the todolist.
Implements KCal::Calendar.
Definition at line 214 of file calendarlocal.cpp.
◆ alarms()
|
virtual |
Return all alarms, which ocur in the given time interval.
Implements KCal::Calendar.
Definition at line 314 of file calendarlocal.cpp.
◆ alarmsTo()
Alarm::List CalendarLocal::alarmsTo | ( | const TQDateTime & | to | ) |
Return all alarms, which ocur before given date.
Definition at line 309 of file calendarlocal.cpp.
◆ appendAlarms()
|
protected |
Append alarms of incidence in interval to list of alarms.
Definition at line 341 of file calendarlocal.cpp.
◆ appendRecurringAlarms()
|
protected |
Append alarms of recurring events in interval to list of alarms.
Definition at line 361 of file calendarlocal.cpp.
◆ close()
|
virtual |
Clears out the current calendar, freeing all used memory etc.
etc.
Implements KCal::Calendar.
Definition at line 92 of file calendarlocal.cpp.
◆ closeEvents()
|
virtual |
Clears out the current calendar, freeing all used memory etc.
etc.
Implements KCal::Calendar.
Definition at line 107 of file calendarlocal.cpp.
◆ closeJournals()
|
virtual |
Clears out the current calendar, freeing all used memory etc.
etc.
Implements KCal::Calendar.
Definition at line 133 of file calendarlocal.cpp.
◆ closeTodos()
|
virtual |
Clears out the current calendar, freeing all used memory etc.
etc.
Implements KCal::Calendar.
Definition at line 120 of file calendarlocal.cpp.
◆ deleteAllEvents()
void CalendarLocal::deleteAllEvents | ( | ) |
Deletes all events from this calendar.
Definition at line 194 of file calendarlocal.cpp.
◆ deleteAllJournals()
void CalendarLocal::deleteAllJournals | ( | ) |
Deletes all journals from this calendar.
Definition at line 702 of file calendarlocal.cpp.
◆ deleteAllTodos()
void CalendarLocal::deleteAllTodos | ( | ) |
Deletes all todos from this calendar.
Definition at line 265 of file calendarlocal.cpp.
◆ deleteChildEvents()
bool CalendarLocal::deleteChildEvents | ( | Event * | event | ) |
Deletes a child event from this calendar.
Definition at line 179 of file calendarlocal.cpp.
◆ deleteChildJournals()
bool CalendarLocal::deleteChildJournals | ( | Journal * | journal | ) |
Delete a child journal from this calendar.
Definition at line 687 of file calendarlocal.cpp.
◆ deleteChildTodos()
bool CalendarLocal::deleteChildTodos | ( | Todo * | todo | ) |
Deletes a child todo from this calendar.
Definition at line 250 of file calendarlocal.cpp.
◆ deleteEvent()
|
virtual |
Deletes an event from this calendar.
Implements KCal::Calendar.
Definition at line 160 of file calendarlocal.cpp.
◆ deleteJournal()
|
virtual |
Remove a Journal from the calendar.
Implements KCal::Calendar.
Definition at line 670 of file calendarlocal.cpp.
◆ deleteTodo()
|
virtual |
Remove a todo from the todolist.
Implements KCal::Calendar.
Definition at line 230 of file calendarlocal.cpp.
◆ event()
|
virtual |
Retrieves an event on the basis of the unique string ID.
Implements KCal::Calendar.
Definition at line 208 of file calendarlocal.cpp.
◆ incidenceUpdated()
|
protected |
Notification function of IncidenceBase::Observer.
Definition at line 499 of file calendarlocal.cpp.
◆ insertEvent()
|
protected |
inserts an event into its "proper place" in the calendar.
Definition at line 513 of file calendarlocal.cpp.
◆ journal()
|
virtual |
Return Journal with given UID.
Implements KCal::Calendar.
Definition at line 714 of file calendarlocal.cpp.
◆ load()
bool CalendarLocal::load | ( | const TQString & | fileName, |
CalFormat * | format = 0 |
||
) |
Loads a calendar on disk in vCalendar or iCalendar format into the current calendar.
Incidences already present are preserved. If an event of the file to be loaded has the same unique id as an incidence already present the new incidence is ignored.
To load a CalendarLocal object from a file without preserving existing incidences call close() before load().
- Returns
- true, if successful, false on error.
- Parameters
-
fileName the name of the calendar on disk. format the format to use. If 0, iCalendar and vCalendar will be used
Definition at line 62 of file calendarlocal.cpp.
◆ rawEvents() [1/2]
|
virtual |
Get unfiltered events in a range of dates.
If inclusive is set to true, only events are returned, which are completely included in the range. If inclusive is set to false, all events which overlap the range are returned. An event's entire time span is considered in evaluating whether it should be returned. For a non-recurring event, its span is from its start to its end date. For a recurring event, its time span is from its first to its last recurrence.
Implements KCal::Calendar.
Definition at line 560 of file calendarlocal.cpp.
◆ rawEvents() [2/2]
|
virtual |
Return unfiltered list of all events in calendar.
Implements KCal::Calendar.
Definition at line 643 of file calendarlocal.cpp.
◆ rawEventsForDate() [1/2]
|
virtual |
Builds and then returns a list of all events that match for the date specified.
useful for dayView, etc. etc.
Implements KCal::Calendar.
Definition at line 526 of file calendarlocal.cpp.
◆ rawEventsForDate() [2/2]
|
virtual |
Get unfiltered events for date qdt.
Implements KCal::Calendar.
Definition at line 638 of file calendarlocal.cpp.
◆ rawJournals()
|
virtual |
Return list of all journals.
Implements KCal::Calendar.
Definition at line 724 of file calendarlocal.cpp.
◆ rawJournalsForDate()
|
virtual |
Get unfiltered journals for a given date.
Implements KCal::Calendar.
Definition at line 729 of file calendarlocal.cpp.
◆ rawTodos()
|
virtual |
Return list of all todos.
Implements KCal::Calendar.
Definition at line 278 of file calendarlocal.cpp.
◆ rawTodosForDate()
|
virtual |
Returns list of todos due on the specified date.
Implements KCal::Calendar.
Definition at line 294 of file calendarlocal.cpp.
◆ reload()
|
virtual |
Reloads the contents of the storage into memory.
The associated file name must be known, in other words a previous load() must have been executed.
- Returns
- success or failure
Implements KCal::Calendar.
Definition at line 69 of file calendarlocal.cpp.
◆ save() [1/2]
|
inlinevirtual |
Sync changes in memory to persistant storage.
Implements KCal::Calendar.
Definition at line 96 of file calendarlocal.h.
◆ save() [2/2]
bool CalendarLocal::save | ( | const TQString & | fileName, |
CalFormat * | format = 0 |
||
) |
Writes out the calendar to disk in the specified format.
CalendarLocal takes ownership of the CalFormat object.
- Parameters
-
fileName the name of the file format the format to use
- Returns
- true, if successful, false on error.
Definition at line 80 of file calendarlocal.cpp.
◆ setTimeZoneIdViewOnly()
|
virtual |
Set the timezone of the calendar to be used for interpreting the events in the calendar.
This requires that the calendar is saved first, so the user is asked whether he wants to do that, or keep the timezone as is.
Implements KCal::Calendar.
Definition at line 744 of file calendarlocal.cpp.
◆ todo()
|
virtual |
Searches todolist for an event with this unique string identifier, returns a pointer or null.
Implements KCal::Calendar.
Definition at line 284 of file calendarlocal.cpp.
The documentation for this class was generated from the following files: