#include <resourcecached.h>
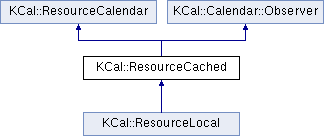
Public Types | |
enum | { ReloadNever , ReloadOnStartup , ReloadInterval } |
enum | { SaveNever , SaveOnExit , SaveInterval , SaveDelayed , SaveAlways } |
Public Member Functions | |
ResourceCached (const TDEConfig *) | |
void | readConfig (const TDEConfig *config) |
void | writeConfig (TDEConfig *config) |
void | setReloadPolicy (int policy) |
int | reloadPolicy () const |
void | setReloadInterval (int minutes) |
int | reloadInterval () const |
void | setSavePolicy (int policy) |
int | savePolicy () const |
void | setSaveInterval (int minutes) |
int | saveInterval () const |
TQDateTime | lastLoad () const |
TQDateTime | lastSave () const |
TDE_DEPRECATED bool | addEvent (Event *event) |
bool | addEvent (Event *event, const TQString &subresource) |
bool | deleteEvent (Event *) |
Event * | event (const TQString &UniqueStr) |
Event::List | events () |
Event::List | rawEvents (EventSortField sortField=EventSortUnsorted, SortDirection sortDirection=SortDirectionAscending) |
Event::List | rawEventsForDate (const TQDate &date, EventSortField sortField=EventSortUnsorted, SortDirection sortDirection=SortDirectionAscending) |
Event::List | rawEventsForDate (const TQDateTime &qdt) |
Event::List | rawEvents (const TQDate &start, const TQDate &end, bool inclusive=false) |
TDE_DEPRECATED bool | addTodo (Todo *todo) |
bool | addTodo (Todo *todo, const TQString &subresource) |
bool | deleteTodo (Todo *) |
Todo * | todo (const TQString &uid) |
Todo::List | rawTodos (TodoSortField sortField=TodoSortUnsorted, SortDirection sortDirection=SortDirectionAscending) |
Todo::List | rawTodosForDate (const TQDate &date) |
TDE_DEPRECATED bool | addJournal (Journal *journal) |
bool | addJournal (Journal *journal, const TQString &subresource) |
bool | deleteJournal (Journal *) |
Journal * | journal (const TQString &uid) |
Journal::List | rawJournals (JournalSortField sortField=JournalSortUnsorted, SortDirection sortDirection=SortDirectionAscending) |
Journal::List | rawJournalsForDate (const TQDate &date) |
Alarm::List | alarms (const TQDateTime &from, const TQDateTime &to) |
Alarm::List | alarmsTo (const TQDateTime &to) |
void | setTimeZoneId (const TQString &timeZoneId) |
TQString | timeZoneId () const |
const Person & | getOwner () const |
void | setOwner (const Person &owner) |
void | enableChangeNotification () |
void | disableChangeNotification () |
void | clearChange (Incidence *) |
void | clearChange (const TQString &uid) |
void | clearChanges () |
bool | hasChanges () const |
Incidence::List | allChanges () const |
Incidence::List | addedIncidences () const |
Incidence::List | changedIncidences () const |
Incidence::List | deletedIncidences () const |
void | loadCache () |
void | saveCache () |
void | clearCache () |
void | clearEventsCache () |
void | clearTodosCache () |
void | clearJournalsCache () |
void | cleanUpEventCache (const KCal::Event::List &eventList) |
void | cleanUpTodoCache (const KCal::Todo::List &todoList) |
KPIM::IdMapper & | idMapper () |
![]() | |
ResourceCalendar (const TDEConfig *) | |
void | clearException () |
void | setException (ErrorFormat *error) |
ErrorFormat * | exception () |
void | setResolveConflict (bool b) |
virtual void | writeConfig (TDEConfig *config) |
virtual TQString | infoText () const |
bool | load () |
bool | save (Incidence *incidence=0) |
virtual bool | isSaving () |
virtual TDEABC::Lock * | lock ()=0 |
virtual TDE_DEPRECATED bool | addIncidence (Incidence *) |
virtual bool | addIncidence (Incidence *, const TQString &subresource) |
virtual bool | deleteIncidence (Incidence *) |
Incidence * | incidence (const TQString &uid) |
virtual TDE_DEPRECATED bool | addEvent (Event *event)=0 |
virtual bool | addEvent (Event *event, const TQString &subresource)=0 |
virtual bool | deleteEvent (Event *)=0 |
virtual Event * | event (const TQString &uid)=0 |
virtual Event::List | rawEvents (EventSortField sortField=EventSortUnsorted, SortDirection sortDirection=SortDirectionAscending)=0 |
virtual Event::List | rawEventsForDate (const TQDate &date, EventSortField sortField=EventSortUnsorted, SortDirection sortDirection=SortDirectionAscending)=0 |
virtual Event::List | rawEventsForDate (const TQDateTime &qdt)=0 |
virtual Event::List | rawEvents (const TQDate &start, const TQDate &end, bool inclusive=false)=0 |
virtual bool | setValue (const TQString &key, const TQString &value) |
virtual TDE_DEPRECATED bool | addTodo (Todo *todo)=0 |
virtual bool | addTodo (Todo *todo, const TQString &subresource)=0 |
virtual bool | deleteTodo (Todo *)=0 |
virtual Todo * | todo (const TQString &uid)=0 |
virtual Todo::List | rawTodos (TodoSortField sortField=TodoSortUnsorted, SortDirection sortDirection=SortDirectionAscending)=0 |
virtual Todo::List | rawTodosForDate (const TQDate &date)=0 |
virtual TDE_DEPRECATED bool | addJournal (Journal *)=0 |
virtual bool | addJournal (Journal *journal, const TQString &subresource)=0 |
virtual bool | deleteJournal (Journal *)=0 |
virtual Journal * | journal (const TQString &uid)=0 |
virtual Journal::List | rawJournals (JournalSortField sortField=JournalSortUnsorted, SortDirection sortDirection=SortDirectionAscending)=0 |
virtual Journal::List | rawJournalsForDate (const TQDate &date)=0 |
virtual Alarm::List | alarms (const TQDateTime &from, const TQDateTime &to)=0 |
virtual Alarm::List | alarmsTo (const TQDateTime &to)=0 |
Incidence::List | rawIncidences () |
virtual void | setTimeZoneId (const TQString &timeZoneId)=0 |
virtual TQStringList | subresources () const |
virtual bool | canHaveSubresources () const |
virtual bool | subresourceActive (const TQString &) const |
virtual bool | subresourceWritable (const TQString &) const |
virtual const TQString | labelForSubresource (const TQString &resource) const |
virtual TQString | subresourceIdentifier (Incidence *incidence) |
virtual bool | removeSubresource (const TQString &resource) |
virtual bool | addSubresource (const TQString &resource, const TQString &parent) |
virtual TQString | subresourceType (const TQString &resource) |
virtual void | beginAddingIncidences () |
virtual void | endAddingIncidences () |
![]() | |
virtual void | calendarModified (bool, Calendar *) |
virtual void | calendarIncidenceAdded (Incidence *) |
virtual void | calendarIncidenceChanged (Incidence *) |
virtual void | calendarIncidenceDeleted (Incidence *) |
Static Public Member Functions | |
static bool | editorWindowOpen () |
static void | setEditorWindowOpen (bool open) |
Protected Slots | |
void | slotReload () |
void | slotSave () |
void | setIdMapperIdentifier () |
Protected Member Functions | |
void | calendarIncidenceAdded (KCal::Incidence *incidence) |
void | calendarIncidenceChanged (KCal::Incidence *incidence) |
void | calendarIncidenceDeleted (KCal::Incidence *incidence) |
virtual void | doClose () |
virtual bool | doOpen () |
bool | checkForReload () |
bool | checkForSave () |
void | checkForAutomaticSave () |
void | addInfoText (TQString &) const |
void | setupSaveTimer () |
void | setupReloadTimer () |
virtual TQString | cacheFile () const |
virtual TQString | changesCacheFile (const TQString &) const |
void | loadChangesCache (TQMap< Incidence *, bool > &, const TQString &) |
void | loadChangesCache () |
void | saveChangesCache (const TQMap< Incidence *, bool > &, const TQString &) |
void | saveChangesCache () |
![]() | |
virtual bool | doLoad ()=0 |
virtual bool | doSave ()=0 |
virtual bool | doSave (Incidence *) |
virtual void | addInfoText (TQString &) const |
void | loadError (const TQString &errorMessage=TQString()) |
void | saveError (const TQString &errorMessage=TQString()) |
Protected Attributes | |
CalendarLocal | mCalendar |
![]() | |
bool | mResolveConflict |
Additional Inherited Members | |
![]() | |
virtual void | setSubresourceActive (const TQString &, bool active) |
![]() | |
void | resourceChanged (ResourceCalendar *) |
void | resourceLoaded (ResourceCalendar *) |
void | resourceSaved (ResourceCalendar *) |
void | resourceLoadError (ResourceCalendar *, const TQString &error) |
void | resourceSaveError (ResourceCalendar *, const TQString &error) |
void | signalSubresourceAdded (ResourceCalendar *, const TQString &type, const TQString &subresource, const TQString &label) |
void | signalSubresourceRemoved (ResourceCalendar *, const TQString &, const TQString &) |
Detailed Description
This class provides a calendar resource using a local CalendarLocal object to cache the calendar data.
Definition at line 45 of file resourcecached.h.
Member Enumeration Documentation
◆ anonymous enum
anonymous enum |
Reload policy.
- See also
- setReloadPolicy(), reloadPolicy()
Definition at line 56 of file resourcecached.h.
◆ anonymous enum
anonymous enum |
Constructor & Destructor Documentation
◆ ResourceCached()
ResourceCached::ResourceCached | ( | const TDEConfig * | config | ) |
Definition at line 51 of file resourcecached.cpp.
◆ ~ResourceCached()
|
virtual |
Definition at line 62 of file resourcecached.cpp.
Member Function Documentation
◆ addedIncidences()
Incidence::List ResourceCached::addedIncidences | ( | ) | const |
Definition at line 541 of file resourcecached.cpp.
◆ addEvent() [1/2]
|
virtual |
Add event to calendar.
Implements KCal::ResourceCalendar.
Definition at line 159 of file resourcecached.cpp.
◆ addEvent() [2/2]
|
virtual |
Implements KCal::ResourceCalendar.
Definition at line 164 of file resourcecached.cpp.
◆ addInfoText()
|
protectedvirtual |
Add info text for concrete resources.
Called by infoText().
Reimplemented from KCal::ResourceCalendar.
Definition at line 687 of file resourcecached.cpp.
◆ addJournal() [1/2]
|
virtual |
Add a Journal entry to calendar.
Implements KCal::ResourceCalendar.
Definition at line 246 of file resourcecached.cpp.
◆ addJournal() [2/2]
|
virtual |
Implements KCal::ResourceCalendar.
Definition at line 251 of file resourcecached.cpp.
◆ addTodo() [1/2]
|
virtual |
Add a todo to the todolist.
Implements KCal::ResourceCalendar.
Definition at line 209 of file resourcecached.cpp.
◆ addTodo() [2/2]
|
virtual |
Implements KCal::ResourceCalendar.
Definition at line 214 of file resourcecached.cpp.
◆ alarms()
|
virtual |
Return all alarms, which ocur in the given time interval.
Implements KCal::ResourceCalendar.
Definition at line 278 of file resourcecached.cpp.
◆ alarmsTo()
|
virtual |
Return all alarms, which ocur before given date.
Implements KCal::ResourceCalendar.
Definition at line 273 of file resourcecached.cpp.
◆ allChanges()
Incidence::List ResourceCached::allChanges | ( | ) | const |
Definition at line 571 of file resourcecached.cpp.
◆ cacheFile()
|
protectedvirtual |
This method is used by loadCache() and saveCache(), reimplement it to change the location of the cache.
Definition at line 418 of file resourcecached.cpp.
◆ calendarIncidenceAdded()
|
protectedvirtual |
Notify the Observer that an Incidence has been inserted.
First parameter is a pointer to the Incidence that was inserted.
Reimplemented from KCal::Calendar::Observer.
Definition at line 480 of file resourcecached.cpp.
◆ calendarIncidenceChanged()
|
protectedvirtual |
Notify the Observer that an Incidence has been modified.
First parameter is a pointer to the Incidence that was modified.
Reimplemented from KCal::Calendar::Observer.
Definition at line 496 of file resourcecached.cpp.
◆ calendarIncidenceDeleted()
|
protectedvirtual |
Notify the Observer that an Incidence has been removed.
First parameter is a pointer to the Incidence that was removed.
Reimplemented from KCal::Calendar::Observer.
Definition at line 513 of file resourcecached.cpp.
◆ changedIncidences()
Incidence::List ResourceCached::changedIncidences | ( | ) | const |
Definition at line 551 of file resourcecached.cpp.
◆ changesCacheFile()
|
protectedvirtual |
Functions for keeping the changes persistent.
Definition at line 423 of file resourcecached.cpp.
◆ checkForAutomaticSave()
|
protected |
Definition at line 662 of file resourcecached.cpp.
◆ checkForReload()
|
protected |
Check if reload required according to reload policy.
Definition at line 674 of file resourcecached.cpp.
◆ checkForSave()
|
protected |
Check if save required according to save policy.
Definition at line 681 of file resourcecached.cpp.
◆ cleanUpEventCache()
void ResourceCached::cleanUpEventCache | ( | const KCal::Event::List & | eventList | ) |
Definition at line 354 of file resourcecached.cpp.
◆ cleanUpTodoCache()
void ResourceCached::cleanUpTodoCache | ( | const KCal::Todo::List & | todoList | ) |
Definition at line 383 of file resourcecached.cpp.
◆ clearCache()
void ResourceCached::clearCache | ( | ) |
Clear cache.
Definition at line 334 of file resourcecached.cpp.
◆ clearChange() [1/2]
void ResourceCached::clearChange | ( | const TQString & | uid | ) |
Definition at line 598 of file resourcecached.cpp.
◆ clearChange() [2/2]
void ResourceCached::clearChange | ( | Incidence * | incidence | ) |
Definition at line 593 of file resourcecached.cpp.
◆ clearChanges()
void ResourceCached::clearChanges | ( | ) |
Definition at line 295 of file resourcecached.cpp.
◆ clearEventsCache()
void ResourceCached::clearEventsCache | ( | ) |
Clear events cache.
Definition at line 339 of file resourcecached.cpp.
◆ clearJournalsCache()
void ResourceCached::clearJournalsCache | ( | ) |
Clear journals cache.
Definition at line 349 of file resourcecached.cpp.
◆ clearTodosCache()
void ResourceCached::clearTodosCache | ( | ) |
Clear todos cache.
Definition at line 344 of file resourcecached.cpp.
◆ deletedIncidences()
Incidence::List ResourceCached::deletedIncidences | ( | ) | const |
Definition at line 561 of file resourcecached.cpp.
◆ deleteEvent()
|
virtual |
Deletes an event from this calendar.
Implements KCal::ResourceCalendar.
Definition at line 171 of file resourcecached.cpp.
◆ deleteJournal()
|
virtual |
Remove a Journal from the calendar.
Implements KCal::ResourceCalendar.
Definition at line 225 of file resourcecached.cpp.
◆ deleteTodo()
|
virtual |
Remove a todo from the todolist.
Implements KCal::ResourceCalendar.
Definition at line 220 of file resourcecached.cpp.
◆ disableChangeNotification()
void ResourceCached::disableChangeNotification | ( | ) |
Definition at line 626 of file resourcecached.cpp.
◆ doClose()
|
protectedvirtual |
Virtual method from KRES::Resource, called when the last instace of the resource is closed.
Definition at line 701 of file resourcecached.cpp.
◆ doOpen()
|
protectedvirtual |
Opens the resource.
Dummy implementation, so child classes don't have to reimplement this method. By default, this does not do anything, but can be reimplemented in child classes
Definition at line 706 of file resourcecached.cpp.
◆ editorWindowOpen()
|
static |
Definition at line 631 of file resourcecached.cpp.
◆ enableChangeNotification()
void ResourceCached::enableChangeNotification | ( | ) |
Definition at line 621 of file resourcecached.cpp.
◆ event()
|
virtual |
Retrieves an event on the basis of the unique string ID.
Implements KCal::ResourceCalendar.
Definition at line 179 of file resourcecached.cpp.
◆ events()
Event::List KCal::ResourceCached::events | ( | ) |
Return filtered list of all events in calendar.
◆ getOwner()
const Person & KCal::ResourceCached::getOwner | ( | ) | const |
Return the owner of the calendar's full name.
Definition at line 717 of file resourcecached.cpp.
◆ hasChanges()
bool ResourceCached::hasChanges | ( | ) | const |
Definition at line 587 of file resourcecached.cpp.
◆ idMapper()
KPIM::IdMapper & ResourceCached::idMapper | ( | ) |
Returns a reference to the id mapper.
Definition at line 413 of file resourcecached.cpp.
◆ journal()
|
virtual |
Return Journal with given unique id.
Implements KCal::ResourceCalendar.
Definition at line 257 of file resourcecached.cpp.
◆ lastLoad()
TQDateTime KCal::ResourceCached::lastLoad | ( | ) | const |
Return time of last load.
◆ lastSave()
TQDateTime KCal::ResourceCached::lastSave | ( | ) | const |
Return time of last save.
◆ loadCache()
void ResourceCached::loadCache | ( | ) |
Loads the cache, this method should be called on load.
Definition at line 302 of file resourcecached.cpp.
◆ loadChangesCache() [1/2]
|
protected |
Definition at line 473 of file resourcecached.cpp.
◆ loadChangesCache() [2/2]
|
protected |
Definition at line 456 of file resourcecached.cpp.
◆ rawEvents() [1/2]
|
virtual |
Get unfiltered events in a range of dates.
If inclusive is set to true, only events are returned, which are completely included in the range.
Implements KCal::ResourceCalendar.
Definition at line 193 of file resourcecached.cpp.
◆ rawEvents() [2/2]
|
virtual |
Return unfiltered list of all events in calendar.
Implements KCal::ResourceCalendar.
Definition at line 204 of file resourcecached.cpp.
◆ rawEventsForDate() [1/2]
|
virtual |
Builds and then returns a list of all events that match for the date specified.
useful for dayView, etc. etc.
Implements KCal::ResourceCalendar.
Definition at line 184 of file resourcecached.cpp.
◆ rawEventsForDate() [2/2]
|
virtual |
Get unfiltered events for date qdt.
Implements KCal::ResourceCalendar.
Definition at line 199 of file resourcecached.cpp.
◆ rawJournals()
|
virtual |
Return list of all journals.
Implements KCal::ResourceCalendar.
Definition at line 262 of file resourcecached.cpp.
◆ rawJournalsForDate()
|
virtual |
Return list of journals for the given date.
Implements KCal::ResourceCalendar.
Definition at line 267 of file resourcecached.cpp.
◆ rawTodos()
|
virtual |
Return list of all todos.
Implements KCal::ResourceCalendar.
Definition at line 231 of file resourcecached.cpp.
◆ rawTodosForDate()
|
virtual |
Returns list of todos due on the specified date.
Implements KCal::ResourceCalendar.
Definition at line 241 of file resourcecached.cpp.
◆ readConfig()
void ResourceCached::readConfig | ( | const TDEConfig * | config | ) |
Definition at line 110 of file resourcecached.cpp.
◆ reloadInterval()
int ResourceCached::reloadInterval | ( | ) | const |
Return reload interval in minutes.
Definition at line 83 of file resourcecached.cpp.
◆ reloadPolicy()
int ResourceCached::reloadPolicy | ( | ) | const |
◆ saveCache()
void ResourceCached::saveCache | ( | ) |
Saves the cache back.
Definition at line 319 of file resourcecached.cpp.
◆ saveChangesCache() [1/2]
|
protected |
Definition at line 449 of file resourcecached.cpp.
◆ saveChangesCache() [2/2]
|
protected |
Definition at line 428 of file resourcecached.cpp.
◆ saveInterval()
int ResourceCached::saveInterval | ( | ) | const |
Return save interval in minutes.
Definition at line 105 of file resourcecached.cpp.
◆ savePolicy()
int ResourceCached::savePolicy | ( | ) | const |
◆ setEditorWindowOpen()
|
static |
Definition at line 636 of file resourcecached.cpp.
◆ setIdMapperIdentifier
|
protectedslot |
Definition at line 329 of file resourcecached.cpp.
◆ setOwner()
void KCal::ResourceCached::setOwner | ( | const Person & | owner | ) |
Set the owner of the calendar.
Should be owner's full name.
Definition at line 712 of file resourcecached.cpp.
◆ setReloadInterval()
void ResourceCached::setReloadInterval | ( | int | minutes | ) |
Set reload interval in minutes which is used when reload policy is ReloadInterval.
Definition at line 78 of file resourcecached.cpp.
◆ setReloadPolicy()
void ResourceCached::setReloadPolicy | ( | int | policy | ) |
Set reload policy.
This controls when the cache is refreshed.
ReloadNever never reload ReloadOnStartup reload when resource is started ReloadInterval reload regularly after given interval
Definition at line 66 of file resourcecached.cpp.
◆ setSaveInterval()
void ResourceCached::setSaveInterval | ( | int | minutes | ) |
Set save interval in minutes which is used when save policy is SaveInterval.
Definition at line 100 of file resourcecached.cpp.
◆ setSavePolicy()
void ResourceCached::setSavePolicy | ( | int | policy | ) |
Set save policy.
This controls when the cache is refreshed.
SaveNever never save SaveOnExit save when resource is exited SaveInterval save regularly after given interval SaveDelayed save after small delay SaveAlways save on every change
Definition at line 88 of file resourcecached.cpp.
◆ setTimeZoneId()
|
virtual |
Set id of timezone, e.g.
"Europe/Berlin"
Implements KCal::ResourceCalendar.
Definition at line 285 of file resourcecached.cpp.
◆ setupReloadTimer()
|
protected |
Definition at line 136 of file resourcecached.cpp.
◆ setupSaveTimer()
|
protected |
Definition at line 125 of file resourcecached.cpp.
◆ slotReload
|
protectedslot |
Definition at line 641 of file resourcecached.cpp.
◆ slotSave
|
protectedslot |
Definition at line 653 of file resourcecached.cpp.
◆ timeZoneId()
TQString ResourceCached::timeZoneId | ( | ) | const |
Definition at line 290 of file resourcecached.cpp.
◆ todo()
|
virtual |
Searches todolist for an event with this unique string identifier, returns a pointer or null.
Implements KCal::ResourceCalendar.
Definition at line 236 of file resourcecached.cpp.
◆ writeConfig()
|
virtual |
Reimplemented from KCal::ResourceCalendar.
Definition at line 147 of file resourcecached.cpp.
Member Data Documentation
◆ mCalendar
|
protected |
Definition at line 309 of file resourcecached.h.
The documentation for this class was generated from the following files: