#include <koincidenceeditor.h>
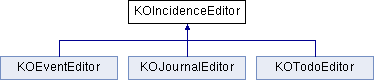
Public Slots | |
virtual void | editIncidence (Incidence *, const TQDate &, Calendar *)=0 |
virtual void | setIncidenceChanger (IncidenceChangerBase *changer) |
virtual void | init ()=0 |
void | addAttachments (const TQStringList &attachments, const TQStringList &mimeTypes=TQStringList(), bool inlineAttachment=false) |
void | addAttendees (const TQStringList &attendees) |
Signals | |
void | deleteAttendee (Incidence *) |
void | editCategories () |
void | updateCategoryConfig () |
void | dialogClose (Incidence *) |
void | editCanceled (Incidence *) |
void | deleteIncidenceSignal (Incidence *) |
void | signalAddAttachments (const TQStringList &attachments, const TQStringList &mimeTypes=TQStringList(), bool inlineAttachment=false) |
Public Member Functions | |
KOIncidenceEditor (const TQString &caption, Calendar *calendar, TQWidget *parent) | |
virtual void | modified () |
virtual void | reload ()=0 |
virtual void | setResource (ResourceCalendar *res, const TQString &subRes) |
virtual void | selectInvitationCounterProposal (bool enable) |
virtual void | selectCreateTask (bool enable) |
void | setRecurringIncidence (Incidence *originalIncidence, Incidence *incAfterDissociation) |
Protected Member Functions | |
virtual TQString | type () |
virtual TQStringList & | templates () const =0 |
virtual void | loadTemplate (CalendarLocal &)=0 |
void | setupAttendeesTab () |
void | setupDesignerTabs (const TQString &type) |
void | saveAsTemplate (Incidence *, const TQString &name) |
void | readDesignerFields (Incidence *i) |
void | writeDesignerFields (Incidence *i) |
TQWidget * | addDesignerTab (const TQString &uifile) |
void | setupEmbeddedURLPage (const TQString &label, const TQString &url, const TQString &mimetype) |
void | createEmbeddedURLPages (Incidence *i) |
virtual bool | processInput () |
virtual void | processCancel () |
void | cancelRemovedAttendees (Incidence *incidence) |
Protected Attributes | |
Calendar * | mCalendar |
KOEditorDetails * | mDetails |
KOAttendeeEditor * | mAttendeeEditor |
KOrg::IncidenceChangerBase * | mChanger |
TQPtrList< KPIM::DesignerFields > | mDesignerFields |
TQMap< TQWidget *, KPIM::DesignerFields * > | mDesignerFieldForWidget |
TQPtrList< TQWidget > | mEmbeddedURLPages |
TQPtrList< TQWidget > | mAttachedDesignerFields |
ResourceCalendar * | mResource |
TQString | mSubResource |
bool | mIsCounter |
bool | mIsCreateTask |
Incidence * | mRecurIncidence |
Incidence * | mRecurIncidenceAfterDissoc |
Detailed Description
This is the base class for the calendar component editors.
Definition at line 57 of file koincidenceeditor.h.
Constructor & Destructor Documentation
◆ KOIncidenceEditor()
KOIncidenceEditor::KOIncidenceEditor | ( | const TQString & | caption, |
Calendar * | calendar, | ||
TQWidget * | parent | ||
) |
Construct new IncidenceEditor.
Definition at line 58 of file koincidenceeditor.cpp.
Member Function Documentation
◆ addAttachments
|
slot |
Adds attachments to the editor.
Definition at line 357 of file koincidenceeditor.cpp.
◆ addAttendees
|
slot |
Adds attendees to the editor.
Definition at line 364 of file koincidenceeditor.cpp.
◆ editIncidence
|
pure virtualslot |
Edit an existing todo.
Implemented in KOTodoEditor, KOEventEditor, and KOJournalEditor.
◆ init
|
pure virtualslot |
Initialize editor.
This function creates the tab widgets.
Implemented in KOTodoEditor, KOJournalEditor, and KOEventEditor.
◆ modified()
|
inlinevirtual |
This incidence has been modified externally.
Reimplemented in KOTodoEditor, KOJournalEditor, and KOEventEditor.
Definition at line 70 of file koincidenceeditor.h.
◆ processInput()
|
inlineprotectedvirtual |
Process user input and create or update event.
Returns false if input is invalid.
Reimplemented in KOTodoEditor, KOJournalEditor, and KOEventEditor.
Definition at line 158 of file koincidenceeditor.h.
◆ setRecurringIncidence()
void KOIncidenceEditor::setRecurringIncidence | ( | Incidence * | originalIncidence, |
Incidence * | incAfterDissociation | ||
) |
This should be called when editing only one occurrence of a recurring incidence, before showing the editor.
It gives the editor a pointer to the original incidence, which contains all occurrences and a pointer to the original incidence already dissociated from the event (mEvent).
If the user presses ok/apply the changes made to the incAfterDissociation are commited to the callendar through mChanger.
If the user presses cancel we restore originalIncidence and all dissociations are discarded
Definition at line 410 of file koincidenceeditor.cpp.
The documentation for this class was generated from the following files: