#include <kolistview.h>
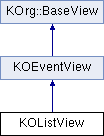
Public Slots | |
virtual void | updateView () |
virtual void | showDates (const TQDate &start, const TQDate &end) |
virtual void | showIncidences (const Incidence::List &incidenceList, const TQDate &date) |
void | clearSelection () |
void | showDates () |
void | hideDates () |
void | changeIncidenceDisplay (Incidence *, int) |
void | defaultItemAction (TQListViewItem *item) |
void | popupMenu (TQListViewItem *item, const TQPoint &, int) |
![]() | |
void | defaultAction (Incidence *) |
![]() | |
virtual void | showDates (const TQDate &start, const TQDate &end)=0 |
virtual void | showIncidences (const Incidence::List &incidenceList, const TQDate &date)=0 |
virtual void | updateView ()=0 |
virtual void | dayPassed (const TQDate &) |
virtual void | setIncidenceChanger (IncidenceChangerBase *changer) |
virtual void | flushView () |
virtual void | changeIncidenceDisplay (Incidence *, int)=0 |
virtual void | updateConfig () |
virtual void | clearSelection () |
virtual bool | eventDurationHint (TQDateTime &, TQDateTime &, bool &) |
Public Member Functions | |
KOListView (Calendar *calendar, TQWidget *parent=0, const char *name=0, bool nonInteractive=false) | |
virtual int | maxDatesHint () |
virtual int | currentDateCount () |
virtual Incidence::List | selectedIncidences () |
virtual DateList | selectedIncidenceDates () |
void | showDates (bool show) |
void | showAll () |
void | readSettings (TDEConfig *config) |
void | writeSettings (TDEConfig *config) |
void | clear () |
TQSize | sizeHint () const |
![]() | |
KOEventView (Calendar *cal, TQWidget *parent=0, const char *name=0) | |
virtual | ~KOEventView () |
KOEventPopupMenu * | eventPopup () |
TQPopupMenu * | newEventPopup () |
bool | isEventView () |
bool | supportsDateNavigation () const |
![]() | |
BaseView (Calendar *cal, TQWidget *parent=0, const char *name=0) | |
virtual | ~BaseView () |
void | setReadOnly (bool readonly) |
bool | readOnly () |
virtual void | setCalendar (Calendar *cal) |
virtual Calendar * | calendar () |
virtual void | setResource (ResourceCalendar *res, const TQString &subResource) |
ResourceCalendar * | resourceCalendar () |
TQString | subResourceCalendar () const |
virtual TQDateTime | selectionStart () |
virtual TQDateTime | selectionEnd () |
virtual CalPrinterBase::PrintType | printType () |
Protected Slots | |
void | processSelectionChange () |
![]() | |
void | popupShow () |
void | popupEdit () |
void | popupDelete () |
void | popupCut () |
void | popupCopy () |
virtual void | showNewEventPopup () |
Protected Member Functions | |
void | addIncidences (const Incidence::List &, const TQDate &date) |
void | addIncidence (Incidence *, const TQDate &date) |
KOListViewItem * | getItemForIncidence (Incidence *incidence) |
Additional Inherited Members | |
![]() | |
void | datesSelected (const DateList) |
void | shiftedEvent (const TQDate &olddate, const TQDate &newdate) |
![]() | |
void | incidenceSelected (Incidence *, const TQDate &) |
void | showIncidenceSignal (Incidence *, const TQDate &) |
void | editIncidenceSignal (Incidence *, const TQDate &) |
void | deleteIncidenceSignal (Incidence *) |
void | cutIncidenceSignal (Incidence *) |
void | copyIncidenceSignal (Incidence *) |
void | pasteIncidenceSignal () |
void | toggleAlarmSignal (Incidence *) |
void | dissociateOccurrenceSignal (Incidence *, const TQDate &) |
void | dissociateFutureOccurrenceSignal (Incidence *, const TQDate &) |
void | startMultiModify (const TQString &) |
void | endMultiModify () |
void | newEventSignal (ResourceCalendar *res, const TQString &subResource) |
void | newEventSignal (ResourceCalendar *res, const TQString &subResource, const TQDate &) |
void | newEventSignal (ResourceCalendar *res, const TQString &subResource, const TQDateTime &) |
void | newEventSignal (ResourceCalendar *res, const TQString &subResource, const TQDateTime &, const TQDateTime &) |
void | newTodoSignal (ResourceCalendar *res, const TQString &subResource, const TQDate &) |
void | newSubTodoSignal (Todo *) |
void | newJournalSignal (ResourceCalendar *res, const TQString &subResource, const TQDate &) |
![]() | |
Incidence * | mCurrentIncidence |
![]() | |
IncidenceChangerBase * | mChanger |
Detailed Description
This class provides a multi-column list view of events.
It can display events from one particular day or several days, it doesn't matter. To use a view that only handles one day at a time, use KODayListView.
multi-column list view of various events.
- See also
- KOBaseView, KODayListView
Definition at line 68 of file kolistview.h.
Member Function Documentation
◆ currentDateCount()
|
virtual |
Return number of currently shown dates.
A return value of 0 means no idea.
Implements KOrg::BaseView.
Definition at line 264 of file kolistview.cpp.
◆ maxDatesHint()
|
virtual |
provides a hint back to the caller on the maximum number of dates that the view supports.
A return value of 0 means no maximum.
Implements KOEventView.
Definition at line 259 of file kolistview.cpp.
◆ selectedIncidenceDates()
|
virtual |
- Returns
- a list of the dates of selected events. Most views can probably only select a single event at a time, but some may be able to select more than one.
Implements KOrg::BaseView.
Definition at line 281 of file kolistview.cpp.
◆ selectedIncidences()
|
virtual |
- Returns
- a list of selected events. Most views can probably only select a single event at a time, but some may be able to select more than one.
Implements KOrg::BaseView.
Definition at line 269 of file kolistview.cpp.
The documentation for this class was generated from the following files: