#include <koeventview.h>
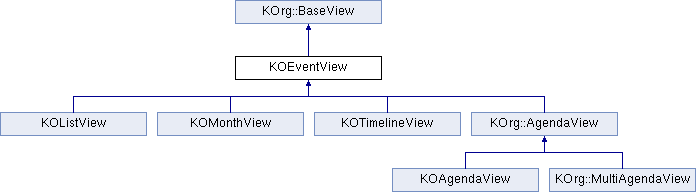
Public Slots | |
void | defaultAction (Incidence *) |
![]() | |
virtual void | showDates (const TQDate &start, const TQDate &end)=0 |
virtual void | showIncidences (const Incidence::List &incidenceList, const TQDate &date)=0 |
virtual void | updateView ()=0 |
virtual void | dayPassed (const TQDate &) |
virtual void | setIncidenceChanger (IncidenceChangerBase *changer) |
virtual void | flushView () |
virtual void | changeIncidenceDisplay (Incidence *, int)=0 |
virtual void | updateConfig () |
virtual void | clearSelection () |
virtual bool | eventDurationHint (TQDateTime &, TQDateTime &, bool &) |
Signals | |
void | datesSelected (const DateList) |
void | shiftedEvent (const TQDate &olddate, const TQDate &newdate) |
![]() | |
void | incidenceSelected (Incidence *, const TQDate &) |
void | showIncidenceSignal (Incidence *, const TQDate &) |
void | editIncidenceSignal (Incidence *, const TQDate &) |
void | deleteIncidenceSignal (Incidence *) |
void | cutIncidenceSignal (Incidence *) |
void | copyIncidenceSignal (Incidence *) |
void | pasteIncidenceSignal () |
void | toggleAlarmSignal (Incidence *) |
void | dissociateOccurrenceSignal (Incidence *, const TQDate &) |
void | dissociateFutureOccurrenceSignal (Incidence *, const TQDate &) |
void | startMultiModify (const TQString &) |
void | endMultiModify () |
void | newEventSignal (ResourceCalendar *res, const TQString &subResource) |
void | newEventSignal (ResourceCalendar *res, const TQString &subResource, const TQDate &) |
void | newEventSignal (ResourceCalendar *res, const TQString &subResource, const TQDateTime &) |
void | newEventSignal (ResourceCalendar *res, const TQString &subResource, const TQDateTime &, const TQDateTime &) |
void | newTodoSignal (ResourceCalendar *res, const TQString &subResource, const TQDate &) |
void | newSubTodoSignal (Todo *) |
void | newJournalSignal (ResourceCalendar *res, const TQString &subResource, const TQDate &) |
Public Member Functions | |
KOEventView (Calendar *cal, TQWidget *parent=0, const char *name=0) | |
virtual | ~KOEventView () |
virtual int | maxDatesHint ()=0 |
KOEventPopupMenu * | eventPopup () |
TQPopupMenu * | newEventPopup () |
bool | isEventView () |
bool | supportsDateNavigation () const |
![]() | |
BaseView (Calendar *cal, TQWidget *parent=0, const char *name=0) | |
virtual | ~BaseView () |
void | setReadOnly (bool readonly) |
bool | readOnly () |
virtual void | setCalendar (Calendar *cal) |
virtual Calendar * | calendar () |
virtual void | setResource (ResourceCalendar *res, const TQString &subResource) |
ResourceCalendar * | resourceCalendar () |
TQString | subResourceCalendar () const |
virtual Incidence::List | selectedIncidences ()=0 |
virtual DateList | selectedIncidenceDates ()=0 |
virtual TQDateTime | selectionStart () |
virtual TQDateTime | selectionEnd () |
virtual CalPrinterBase::PrintType | printType () |
virtual int | currentDateCount ()=0 |
virtual bool | isEventView () |
virtual bool | supportsDateNavigation () const |
Protected Slots | |
void | popupShow () |
void | popupEdit () |
void | popupDelete () |
void | popupCut () |
void | popupCopy () |
virtual void | showNewEventPopup () |
Protected Attributes | |
Incidence * | mCurrentIncidence |
![]() | |
IncidenceChangerBase * | mChanger |
Detailed Description
KOEventView is the abstract base class from which all other calendar views for event data are derived.
It provides methods for displaying appointments and events on one or more days. The actual number of days that a view actually supports is not defined by this abstract class; that is up to the classes that inherit from it. It also provides methods for updating the display, retrieving the currently selected event (or events), and the like.
Abstract class from which all event views are derived.
- See also
- KOListView, KOAgendaView, KOMonthView
Definition at line 55 of file koeventview.h.
Constructor & Destructor Documentation
◆ KOEventView()
KOEventView::KOEventView | ( | Calendar * | cal, |
TQWidget * | parent = 0 , |
||
const char * | name = 0 |
||
) |
Constructs a view.
- Parameters
-
cal is a pointer to the calendar object from which events will be retrieved for display. parent is the parent TQWidget. name is the view name.
Definition at line 47 of file koeventview.cpp.
◆ ~KOEventView()
|
virtual |
Destructor.
Views will do view-specific cleanups here.
Definition at line 54 of file koeventview.cpp.
Member Function Documentation
◆ datesSelected
|
signal |
when the view changes the dates that are selected in one way or another, this signal is emitted.
It should be connected back to the KDateNavigator object so that it changes appropriately, and any other objects that need to be aware that the list of selected dates has changed.
◆ defaultAction
|
slot |
Perform the default action for an incidence, e.g.
open the event editor, when double-clicking an event in the agenda view.
Definition at line 154 of file koeventview.cpp.
◆ eventPopup()
KOEventPopupMenu * KOEventView::eventPopup | ( | ) |
Construct a standard context menu for an event.
Definition at line 60 of file koeventview.cpp.
◆ isEventView()
|
inlinevirtual |
This view is an view for displaying events.
Reimplemented from KOrg::BaseView.
Definition at line 92 of file koeventview.h.
◆ maxDatesHint()
|
pure virtual |
provides a hint back to the caller on the maximum number of dates that the view supports.
A return value of 0 means no maximum.
Implemented in KOAgendaView, KOListView, KOMonthView, KOTimelineView, and KOrg::MultiAgendaView.
◆ newEventPopup()
TQPopupMenu * KOEventView::newEventPopup | ( | ) |
Construct a standard context that allows to create a new event.
Definition at line 86 of file koeventview.cpp.
◆ popupCopy
|
protectedslot |
Definition at line 131 of file koeventview.cpp.
◆ popupCut
|
protectedslot |
Definition at line 124 of file koeventview.cpp.
◆ popupDelete
|
protectedslot |
Definition at line 117 of file koeventview.cpp.
◆ popupEdit
|
protectedslot |
Definition at line 110 of file koeventview.cpp.
◆ popupShow
|
protectedslot |
Definition at line 103 of file koeventview.cpp.
◆ shiftedEvent
|
signal |
Emitted when an event is moved using the mouse in an agenda view (week / month).
◆ showNewEventPopup
|
protectedvirtualslot |
Definition at line 138 of file koeventview.cpp.
◆ supportsDateNavigation()
|
inlinevirtual |
Returns true if the view supports navigation through the date navigator ( selecting a date range, changing month, changing year, etc.
)
Reimplemented from KOrg::BaseView.
Definition at line 94 of file koeventview.h.
Member Data Documentation
◆ mCurrentIncidence
|
protected |
Definition at line 131 of file koeventview.h.
The documentation for this class was generated from the following files: