#include <komonthview.h>
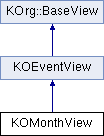
Public Slots | |
virtual void | updateView () |
virtual void | updateConfig () |
virtual void | showDates (const TQDate &start, const TQDate &end) |
virtual void | showIncidences (const Incidence::List &incidenceList, const TQDate &date) |
void | changeIncidenceDisplay (Incidence *, int) |
void | changeIncidenceDisplayAdded (Incidence *, MonthViewCell::CreateItemVisitor &) |
void | clearSelection () |
void | showEventContextMenu (Calendar *, Incidence *, const TQDate &) |
void | showGeneralContextMenu () |
void | setSelectedCell (MonthViewCell *) |
![]() | |
void | defaultAction (Incidence *) |
![]() | |
virtual void | showDates (const TQDate &start, const TQDate &end)=0 |
virtual void | showIncidences (const Incidence::List &incidenceList, const TQDate &date)=0 |
virtual void | updateView ()=0 |
virtual void | dayPassed (const TQDate &) |
virtual void | setIncidenceChanger (IncidenceChangerBase *changer) |
virtual void | flushView () |
virtual void | changeIncidenceDisplay (Incidence *, int)=0 |
virtual void | updateConfig () |
virtual void | clearSelection () |
Public Member Functions | |
KOMonthView (Calendar *cal, TQWidget *parent=0, const char *name=0) | |
virtual int | maxDatesHint () |
virtual int | currentDateCount () |
virtual Incidence::List | selectedIncidences () |
virtual DateList | selectedIncidenceDates () |
virtual TQDateTime | selectionStart () |
virtual TQDateTime | selectionEnd () |
virtual bool | eventDurationHint (TQDateTime &startDt, TQDateTime &endDt, bool &allDay) |
![]() | |
KOEventView (Calendar *cal, TQWidget *parent=0, const char *name=0) | |
virtual | ~KOEventView () |
KOEventPopupMenu * | eventPopup () |
TQPopupMenu * | newEventPopup () |
bool | isEventView () |
bool | supportsDateNavigation () const |
![]() | |
BaseView (Calendar *cal, TQWidget *parent=0, const char *name=0) | |
virtual | ~BaseView () |
void | setReadOnly (bool readonly) |
bool | readOnly () |
virtual void | setCalendar (Calendar *cal) |
virtual Calendar * | calendar () |
virtual void | setResource (ResourceCalendar *res, const TQString &subResource) |
ResourceCalendar * | resourceCalendar () |
TQString | subResourceCalendar () const |
virtual CalPrinterBase::PrintType | printType () |
Protected Slots | |
void | processSelectionChange () |
![]() | |
void | popupShow () |
void | popupEdit () |
void | popupDelete () |
void | popupCut () |
void | popupCopy () |
virtual void | showNewEventPopup () |
Protected Member Functions | |
void | resizeEvent (TQResizeEvent *) |
void | viewChanged () |
void | updateDayLabels () |
Additional Inherited Members | |
![]() | |
void | datesSelected (const DateList) |
void | shiftedEvent (const TQDate &olddate, const TQDate &newdate) |
![]() | |
void | incidenceSelected (Incidence *, const TQDate &) |
void | showIncidenceSignal (Incidence *, const TQDate &) |
void | editIncidenceSignal (Incidence *, const TQDate &) |
void | deleteIncidenceSignal (Incidence *) |
void | cutIncidenceSignal (Incidence *) |
void | copyIncidenceSignal (Incidence *) |
void | pasteIncidenceSignal () |
void | toggleAlarmSignal (Incidence *) |
void | dissociateOccurrenceSignal (Incidence *, const TQDate &) |
void | dissociateFutureOccurrenceSignal (Incidence *, const TQDate &) |
void | startMultiModify (const TQString &) |
void | endMultiModify () |
void | newEventSignal (ResourceCalendar *res, const TQString &subResource) |
void | newEventSignal (ResourceCalendar *res, const TQString &subResource, const TQDate &) |
void | newEventSignal (ResourceCalendar *res, const TQString &subResource, const TQDateTime &) |
void | newEventSignal (ResourceCalendar *res, const TQString &subResource, const TQDateTime &, const TQDateTime &) |
void | newTodoSignal (ResourceCalendar *res, const TQString &subResource, const TQDate &) |
void | newSubTodoSignal (Todo *) |
void | newJournalSignal (ResourceCalendar *res, const TQString &subResource, const TQDate &) |
![]() | |
Incidence * | mCurrentIncidence |
![]() | |
IncidenceChangerBase * | mChanger |
Detailed Description
The class KOMonthView represents the monthly view in KOrganizer.
It holds several instances of the class MonthViewCell.
KOMonthview represents the montly view in KOrganizer.
- See also
- KOBaseView, KODayListView, MonthViewCell
Definition at line 245 of file komonthview.h.
Member Function Documentation
◆ currentDateCount()
|
virtual |
Returns number of currently shown dates.
Implements KOrg::BaseView.
Definition at line 889 of file komonthview.cpp.
◆ eventDurationHint()
|
virtual |
Set the default start/end date/time for new events.
Return true if anything was changed
Reimplemented from KOrg::BaseView.
Definition at line 918 of file komonthview.cpp.
◆ maxDatesHint()
|
virtual |
Returns maximum number of days supported by the komonthview.
Implements KOEventView.
Definition at line 884 of file komonthview.cpp.
◆ selectedIncidenceDates()
|
virtual |
Returns dates of the currently selected events.
Implements KOrg::BaseView.
Definition at line 906 of file komonthview.cpp.
◆ selectedIncidences()
|
virtual |
Returns the currently selected events.
Implements KOrg::BaseView.
Definition at line 894 of file komonthview.cpp.
◆ selectionEnd()
|
virtual |
Returns the end of the selection, or an invalid TQDateTime if there is no selection or the view doesn't support selecting cells.
Reimplemented from KOrg::BaseView.
Definition at line 1021 of file komonthview.cpp.
◆ selectionStart()
|
virtual |
Returns the start of the selection, or an invalid TQDateTime if there is no selection or the view doesn't support selecting cells.
Reimplemented from KOrg::BaseView.
Definition at line 1012 of file komonthview.cpp.
The documentation for this class was generated from the following files: